In this tutorial, we will be showing you how to use the strlen() function to retrieve the length of a string within PHP.
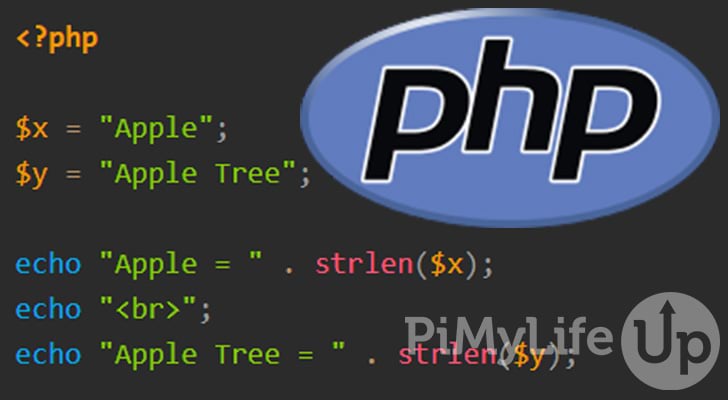
The strlen function within PHP measures the length of a given string. It is extremely useful for performing checks and comparisons that involve string data types. If you plan on using PHP quite a bit, you will eventually need to use this function.
I recommend taking the time to learn more about using strings in PHP if you are new to the language. They are handled similarly to most other programming languages, but there are a few things that you will need to learn.
This tutorial assumes you are using PHP 8.1 or newer. Older versions of PHP might behave slightly differently from what is described in this tutorial. Therefore, we recommend upgrading to the latest PHP version for optimal compatibility and security.
Syntax of the PHP strlen() Function
The syntax of the strlen function is very simple and will accept only a single parameter.
strlen($string)
$string is the string you are checking for the total length. The expected data type is a string, and any other type may result in an error or unexpected results.
The return value of this function will be an integer and contain the length of the string. If the string is empty, the function will return 0.
Examples of Using the strlen() Function
We will use a few examples to demonstrate how the strlen function works in PHP. It is a straightforward function, but there are a few things you need to be careful with, such as dealing with UTF-8 characters.
Counting a Basic String
In the example below, we check two different strings and output the length of each of them using echo. The function will count all characters, including whitespace and special characters.
It is important to note that the strlen()
function will return the string’s length in bytes. So, if you have characters that are multiple bytes (Some UTF-8 characters), you might not get the correct count that you are expecting. If that is the case, you can use an alternative method.
<?php
$x = "Apple";
$y = "Apple Tree";
echo "Apple = " . strlen($x);
echo "<br>";
echo "Apple Tree = " . strlen($y);
The output of the PHP script is below and showcases how strlen measured the two different strings stored in variables x
and y
.
Apple = 5
Apple Tree = 10
Converting UTF-8 Characters
If your string contains UTF-8 characters, you might get a string length that does not match your expectations. This issue is caused by the fact that strlen counts the number of bytes, not characters. UTF-8 characters can range from 1 to 4 bytes which can vary the total length of a string quite a bit.
To fix this issue, you can use mb_strlen()
which counts characters and not bytes, or you can simply decode the string before using it with str_len().
In our example below, we have “hello” translated into Greek “Χαίρετε“. We print out our three different methods of checking the length of the string.
<?php
$x = "Χαίρετε";
echo "Χαίρετε = " . strlen($x);
echo "<br>";
echo "UTF-8 Decode = " . strlen(utf8_decode($x));
echo "<br>";
echo "mb_strlen() = " . mb_strlen($x, 'utf8');
The output below demonstrates how strlen can provide you with a different count than what you may expect. By decoding the UTF-8 string, we can get a more accurate count on the number of characters. Alternatively, the mb_strlen()
function will provide us with the correct number.
This difference may only be an issue where you are expecting a certain number of characters, and you have forgotten to factor in UTF-8 characters or other characters represented by more than a single byte.
Χαίρετε = 14
UTF-8 Decode = 7
mb_strlen() = 7
Conclusion
This tutorial has taken you through the basics of using the PHP strlen function. We covered the basic usage of the function and how you can handle strings that contain UTF-8 characters. It is an extremely useful function you will likely use when handling strings in PHP.
If you are new to PHP or looking to learn more, I highly recommend looking at some of our other PHP tutorials. We cover the basics such as arrays, functions, switch statements, if else statements, and much more.
If we can improve this tutorial, please do not hesitate to let us know by leaving a comment below.