In this guide, we will be showing you how to use the if, else, and elseif conditional statements in PHP.
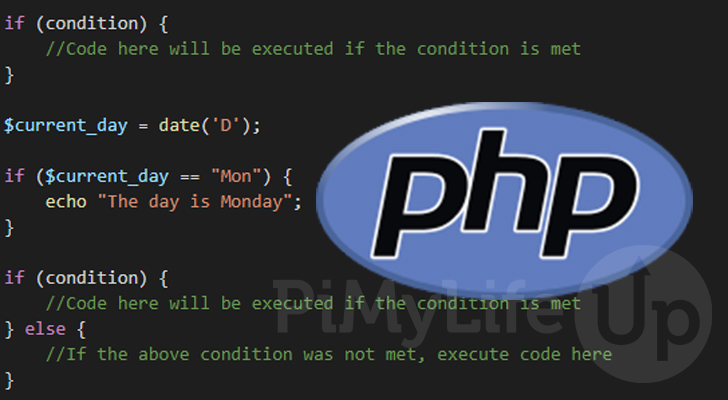
Conditional statements are one of the first things you should learn when you are starting out with the PHP language.
These statements allow you to perform an action when your stated condition is met. They are a critical part of writing a script in PHP.
Over the next few sections, we will touch on three of these conditional statements. We will be touching on the “if
“, “if...else
“, “if...elseif
“, “if...elseif...else
” conditional statements as well as nested if statements.
Code contained within both the if
and else if
statements will only run if the statement returns true
.
Table of Contents
- The if Statement
- The if…else Statement
- The if…elseif Statement
- The if…elseif…else Statement
- Nested if Statements
- Conclusion
The if Statement in PHP
The first PHP conditional statement that we will be touching on is the if
statement. It is the most simple statement you can use in the PHP language.
The if statement allows you to check if a particular condition evaluates to true. If the statement is true, PHP will run the code within the curly brackets ({}
).
Below is an example of how you would write a simple if statement within your PHP script.
if (condition) {
//Code here will be executed if the condition is met
}
Copy
Example of Using the if Statement
We will now run you through a simple PHP script we wrote to show you how the if statement works.
Within this example, we will get the current day using the date()
function and store it in a variable called “$current_day
“.
Using the if
statement within our PHP script, we will check to see if the current day equals “Mon
“.
If our day is Monday, then our script will use the echo statement to print out the text “The day is Monday
“.
<?php
$current_day = date('D');
if ($current_day == "Mon") {
echo "The day is Monday";
}
Copy
The if…else Statement in PHP
The next PHP conditional statement we will be looking at is the else statement. You must always use an else statement last in your condition chain. For example, after an “if
” or “else if
” statement.
The else
statement allows you to perform a task if your if
condition returned false
.
This conditional statement is useful when you want code to be executed if your if
statement condition isn’t met.
Below is an example of a typical if...else
statement when written in a PHP script.
if (condition) {
//Code here will be executed if the condition is met
} else {
//If the above condition was not met, execute code here
}
Copy
Example of Using an if…else Statement
Now let us go into a simple example of using the if...else
statement. We have expanded upon our if statement example.
If our “$current_day
” variable is equal to “Mon
“, then our script will print out the text “The day is Monday
“.
If the “$current_day
” variable is not equal to “Mon
“, PHP will run the code within our else
statement.
Within this else statement, we use echo to display the text “The day is not Monday
” to the output.
<?php
$current_day = date('D');
if ($current_day == "Mon") {
echo "The day is Monday";
} else {
echo "The day is not Monday".
}
Copy
The if…elseif Statement in PHP
The PHP language allows you to add a conditional statement after an if
statement. This is what is called the “elseif
” statement. You can also write the “elseif
” statement as “else if
“.
They are used after an if
statement. It allows you to check if a conditional statement returns true if your previous statement returns false
.
This is useful if you want to check another condition if the first one proved to be false.
Below is an example of writing an if...elseif
statement within a PHP script.
if (conditon) {
//Code here will be executed if the condition is met
} elseif (condition) {
//If the last condition wasn't met, check this condition.
//If this condition is true, then run the code in this block
}
Copy
Example of Using the if…elseif Statement
Let us run through the following example. This example will show you how a if...elseif
statement can be written within a PHP script.
With this example, we retrieve the current day of the week and store using the “date()
” function and store it into our “$current_day
” variable.
We then have our first if statement. This conditional statement checks if our current day equals “Mon
“.
If the day is not equal to “Mon
“, it falls through to our elseif
statement.
Within this elseif
statement, we check if the current day is equal to “Tue
“, we will echo the text “The day is Tuesday
“.
<?php
$current_day = date('D');
if ($current_day == "Mon") {
echo "The day is Monday";
} elseif ($current_day == "Tue") {
echo "The day is Tuesday";
}
Copy
The if…elseif…else Statement in PHP
The if...elseif...else
statement shows how you can combine three conditional statements in PHP.
You always need to start a conditional statement by using the if statement.
After this, you can now use either an “else
” or “elseif
” statement. As we want to check for an additional condition, we will be utilizing the “elseif
” statement.
You can add as many elseif
statements as you would like. So you can use them one after another without issue. However, it is better to use a switch statement in some cases.
Finally, this chain is ended with an else
statement. If the first if
statements weren’t met, then PHP will run the code within this statement.
Below is an example of how you would write the “if...elseif...else
” statement within a PHP script.
if (condition) {
//Code here will be executed if the condition is met
} elseif (condition) {
//If previous condition wasn't met, check this condition is met
//Runs the code within this block when the condition is met
} else {
//If none of the previous conditions were met, run this code
}
Copy
Example of Using the if…elseif…else Statement
With this example, we retrieve the current day using the date function and store it within the “$current_day
” variable.
With our first if
statement in this PHP script, we check if the current day is set to “Mon
“. The text “The day is Monday
” will be displayed if it currently Monday.
If the day isn’t Monday, it will fall through to our second conditional statement. With the “elseif
” statement, we check if the “$current_day
” variable is set to “Tue
“. If the current day is Tuesday, we use echo to print the text “The Day is Tuesday
“.
If the previous two conditional statements return false, the code will fall into the else
statement.
Within this else statement, we use the PHP echo statement to print out the text “The day is not Monday or Tuesday
“.
<?php
$current_day = date('D');
if ($current_day == "Mon") {
echo "The day is Monday";
} elseif ($current_day == "Tue") {
echo "The day is Tuesday";
} else {
echo "The day is not Monday or Tuesday";
}
Copy
Nested if Statements in PHP
Another useful way to use if statements within PHP is to nest them within each other. Using an if statement in this way, you can check for a condition if the first statement returns true.
A nested statement allows you to run a second conditional statement if the first statement returns true.
Below we have included an example of writing a nested if statement within PHP.
if (condition) {
//Code will be run if the above condition is true
if (condition) {
//The condition will be checked, only if the above was true
//Code will run if the above condition is true
}
}
Copy
Example of Using a Nested if Statement
We will show you a basic usage of nested if statements for this PHP example.
Like our previous examples, we start by storing the current day within our "$current_day
” variable.
We then utilize the if
statement to check if the “$current_day
” variable equals “Mon
.”. If the condition evaluates to true, PHP will run the code block within that statement.
The first thing we do within our if statement is echo the text “The day is Monday
“. We then create a new variable called “$current_hour
” where we utilize the date()
function to retrieve the current hour in 24-hour format.
We then get to our nested if statement. For the condition for this statement, we check to see if the current hour is greater than (>
) or equal to (=
) the number 18
(6:00 PM in 24 hour time).
If our nested condition matches we use echo to show the text “It is night time
“.
<?php
$current_day = date('D');
if ($current_day == "Mon") {
echo "The day is Monday";
$current_hour = date('H');
if ($current_hour >= 18) {
echo "It is night time";
}
}
Copy
Conclusion
Within this guide, you will have learned how to use various forms of the if conditional statement within the PHP programming language. These forms include the if
, if..else
, if...elseif
, and if...elseif...else
statements.
Conditional statements are a core part of the PHP language as they help you perform different actions depending on the results of your conditions.
Additionally, we also touched on how you can use nested if statements.
If you have any questions about using if
statements within the PHP language, please comment below.
Be sure to check out our other PHP tutorials or if you are interested in other programming languages, check out our general coding guides.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support