This tutorial will show you how to use the date() function within your PHP scripts.
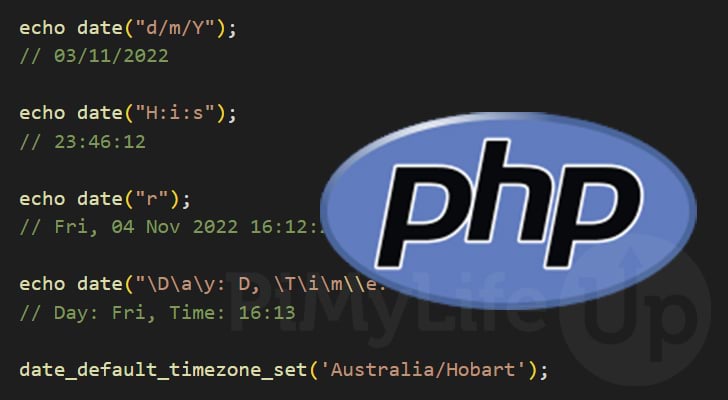
The date() function in PHP allows you to print the date and time using the specified format.
You can get the date and time from any point by specifying a Unix timestamp. However, if you don’t specify a timestamp, then the date() function will use the current date and time as retrieved from the “time()” function.
While this function may initially seem confusing, it is relatively simple once you know what characters are replaced within the string.
Over the following sections, you will learn all there is to know about using this function within your scripts.
Syntax of the date() Function in PHP
Before we show you how to use the date() function within PHP, let us start by exploring its syntax. The syntax shows you how the function is written, what data it expects, and what data it returns.
When writing the date or time format, five characters are commonly used to separate each value. These are good values as they are guaranteed not to be replaced by the date() function.
date(string $format, ?int $timestamp = null): string
Let us explore this syntax by going more in-depth on the two parameters.
format
– The first parameter allows you to specify the format for the outputted string. What you specify here will dictate the resulting output by this function.
For example, you can use PHP’s date() function to only print the current year. You can also format the string to print the day, month, year, and time if you choose to.
We will shortly explore the values you will use to format your date() strings.timestamp
– The second parameter is entirely optional but is where you can specify a Unix timestamp.
PHP’s date() function will use the current server time if this option is not included or is set to null.
A Unix timestamp is a number typically presented as an integer like the following “1667472356
“.
Formatting the Timestamp for PHP’s date() Function
Formatting the timestamp for PHP’s date() function requires you to know the letters that PHP will substitute in the outputted string.
When writing the format for the date or time there is five different characters you will want to use to separate each value. These are good values as they are guaranteed not to be replaced by the date() function.
- Hyphen (
-
) - Dot (
.
) - Forward Slash (
/
) - Semicolon (
:
) - Space (
Please note that this string is case sensitive as certain letters will have a different replacement depending on whether they are lowercase or uppercase.
Date Replacement Characters
To get started, let us first explore the letters used to represent the date within PHP. We will split these into three different categories covering the “day“, “month“m and “year“.
Day
d
– This is the day of the month expressed as a two-digit number with leading zeroes (01
to31
).j
– Day of the month without leading zeroes (1
to31
).S
– English ordinal suffix for the day of the month. (st
,nd
,rd
, orth
).D
– The day of the week expressed as an abbreviated string (Mon
,Tue
,Wed
, etc…).l
– The full name for the day of the week (Monday
,Tuesday
,Wednesday
, etc…).w
– A number representation of the current day of the week (0
for Sunday,6
for Monday).z
– Current day of the year (0
to365
).W
– Current week number of the year using the ISO-8601 standard (Weeks start on Monday)
Month
m
– The month is expressed as a number with leading zeroes (01
to12
).n
– Month is expressed as a number without leading zeroes (1
to12
).M
– The month expressed as a three-letter string (Jan
,Feb
,Mar
,Apr
, etc…).F
– Replaced with the full name of the month (January
,February
,March
,April
, etc…).t
– The number of days for the month in the provided timestamp (31
,30
,28
,29
).
Year
y
– Outputs the year as a two-digit number (98
,18
,20
,22
).Y
– This letter is replaced with the year but as a four-digit number (1998
,2018
,2020
,2022
).o
– The year is output using the ISO-8601 standard (1998
,2018
,2020
,2022
)
Example of using the date() Function to Output the Day
Let us show you this in practice by writing a short PHP script that uses the date() function to print the current date.
With the output from this snippet, you will see the letter “d
” was replaced with the day of the month, “m
” was swapped with the month expressed as a number, and finally, “Y
” was changed with the year as a four-digit number.
We will use PHP’s echo statement to print out the string returned by the date() function.
<?php
echo date("d/m/Y");
?>
Below you can see an example of what was output by our above example.
03/11/2022
Time Replacement Characters
The next lot of replacement characters we will explore are the ones that PHP’s date() function will replace with the current time.
Hours
h
– Replaced with the hour using the 12-hour format with leading zeroes (01
to12
).g
– Swapped with the 12-hour format without leading zeroes (1
to12
).H
– The hour expressed using the 24-hour format with leading zeroes (00
to23
).G
– The current hour using the 24-hour format without leading zeroes (0
to23
).a
– Lowercase ante meridian or post meridian depending on the time (am
orpm
)A
– Uppercase ante meridian or post meridian depending on the time (AM
orPM
)
Minutes
i
– Swapped with the minute (00
to59
).
Seconds
s
– Switched with the current second from the provided timestamp (00
to59
).u
– This letter is switched out with the microseconds from the timestamp (E.G.,22.082300
).U
– Seconds since the Unix epoch (E.G.,1667536995
)
Timezone
e
– This letter is replaced with the time zone (Australia/Hobart
,UTC
,GMT
).T
– Abbreviation of the timezone (EST
,MDT
,GMT
,AEST
, etc…).O
– Difference to GMT without colon between hours and minutes (E.G.,+1100
).P
– Difference to GMT with a colon between hours and minutes (E.G.,+11:00
).Z
– The timezone offset is expressed as seconds (-43200
to50400
).I
– Whether the provided timestamp is in daylight saving time (1
for Daylight Saving Time, or0
otherwise).
Example of using the date() Function to Output the Time
To showcase this in action, let us write a short PHP script that uses the date() function to print the current time using 24-hour format.
Since we want the time using the 24-hour format we start our format string with a capital “H
“, followed by “i
” for the minute and “s
” for the second.
<?php
echo date("H:i:s");
?>
Running the above code, you will end up with a result as shown below. Since we didn’t specify a timestamp, this will be your system’s current time.
23:46:12
Full Date/Time Character Replacements for use with the date() Function
The date() function in PHP has several characters that will be replaced with the full date and time using different standards.
c
– The date and time using the ISO 8601 standard (E.G.,2004-02-12T15:19:21+00:00
)r
– The date and time using the RFC 2822/RFC 5322 standard (E.G.,Thu, 21 Dec 2000 16:01:07 +0200
)
Let us use the “r
” letter within the date() functions format to showcase how this works within PHP to output the date and time.
<?php
echo date("r");
?>
If you were to run the example above, you would get the date and time output with the following output.
Fri, 04 Nov 2022 16:12:23 +1100
Escaping Characters in the PHP date() Format String
To use letters within your PHP date() format string without it being swapped out with a date or time, then you need to escape it.
You can escape a reserved character by placing a backward slash (\
) in front of it. For example, to use the letter “a
” within the format string, you would use “\a
“.
To give an example of how this works, let us output the current day and time.
<?php
echo date("\D\a\y: D, \T\i\m\\e: H:i");
?>
After running the above example, you will end up with a result similar to the one we have shown below.
Day: Fri, Time: 16:13
Getting the Current Date and Time in PHP
One of the key reasons you might want to use the date() function within your PHP script is to get the current date and time.
The previous sections covered the various characters you can use in your format string. We will now put these all to use within the following example.
1. You will need to decide whether you want to use your server’s time zone or a different one. By default, PHP will get the server time.
Instead of using a different time zone, you will first need to call the “date_default_timezone_set()
” function.
Within this function’s singular parameter, you will specify the time zone for your area. For our example, this will be “Australia/Hobart
“.
You can find the time zone to specify for this function by browsing the official PHP time zone manual.
date_default_timezone_set('Australia/Hobart');
2. With the time zone set, you can now use PHP’s date() function to output the current date and time.
Here we put all of the characters we learned earlier to use. We start the format string by specifying the date, using a forward slash as the separator.
The letter “d
” is replaced with the current day of the month. We then use the “m
” letter to specify the current month. Finally, to finish the date, we use the capital letter “Y
” to print the year as a four-digit number.
Next, we utilize the letter “h
” to specify the current hour, “i
” to specify the minute, and finally, “s
” to specify the current second.
We end the format string by using the letter “a
” to print “am
” or “pm
“. If you prefer to use 24-hour time, remove “a
” from the timestamp, and change the letter “h
” to a capital “H
“.
echo date('d/m/Y h:i:s a');
3. Once put together, your script will look like the following. Using the date function like this, you can get the current date and time in one string.
<?php
date_default_timezone_set('Australia/Hobart');
echo date('d/m/Y h:i:s a');
?>
4. If you were to run this code, you should see the script output the current date and time.
04/11/2022 09:05:54 pm
Conclusion
At this point in the tutorial, you should know how to use the date() function in PHP.
This function is the best way to get the date or time within your PHP scripts.
Please comment below if you have questions about using PHP’s date() function.
If you found this guide helpful, we have plenty of other PHP tutorials worth exploring. Likewise, to learn a new language, we have many other coding guides.