In this tutorial, we will go through the steps of checking if an array is empty in the PHP programming language.
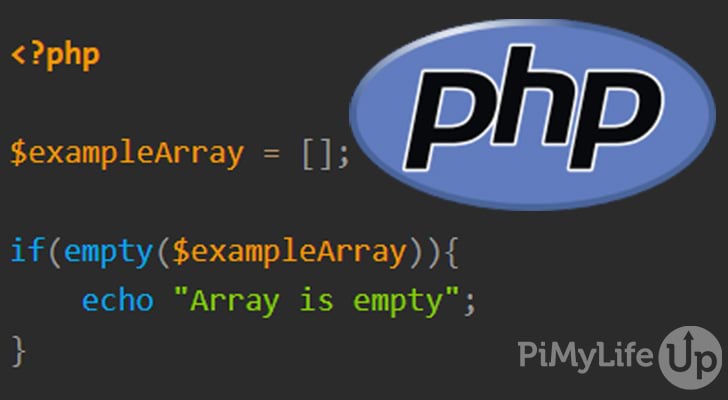
There are a few different methods that you can use to check if an array is empty in PHP. In this tutorial, we will touch on using the not operator and the empty and count function.
Depending on your goal, you might need to check if an array is empty before proceeding to a section of code. For example, you might require the array to be empty. Another reason is that you may want to check that the array contains data before proceeding.
Using one of the options below will allow you to check to see if an array is empty before proceeding with code. Each of these methods will work, so it will come down to personal preference on which option you choose. For example, using the empty function might be preferable if you are unsure if the variable will even exist.
Using the empty
Function
The first method that I will touch on is the empty function. You can use the empty function to check if any variable is empty, but in this case, we will use it on an array.
The empty functions’ syntax is very straightforward as it only accepts a single variable. The variable you supply to the function will be the array you wish to check if it is empty. The function will return true or false depending on if the array is empty.
empty(mixed $var): bool
In the code below, we create an empty array to test the empty function. We also create an if statement that contains our empty function and the array variable. Since our array is empty, we expect the echo to print our string to the browser or terminal.
<?php
$exampleArray = [];
if(empty($exampleArray)){
echo "Array is empty";
}
If you run the script above, you will get the output below. However, if you add an element into the array and rerun the script, you should not get any output as the array is no longer empty.
Array is empty
Using the count
Function
Using the count function is another way to check if an array is empty. The count function will count all the elements within a countable object or array. Since an empty array will have 0
elements, you can use count to check how many elements an array contains.
Below is the syntax of the count function. The function accepts two arguments, but only the first argument is required.
- The first argument expects a countable object or array.
- The second optional argument expects the mode. By default, the mode is set to
COUNT_NORMAL
, but you can change it toCOUNT_RECURSIVE
. Using the recursive option will have count recursively count the array, which is important for multidimensional arrays. - Finally, the function will return an
int
(the final count).
count(countable $var, $mode = COUNT_RECURSIVE): int
The code below demonstrates how to use the count function to check if an array is empty. We first create an empty array to test. Second, we have an if statement with the condition that if the count of the array is 0 (empty), then run the code inside the block. Inside the code block, we echo that the array is empty.
<?php
$exampleArray = [];
if(count($exampleArray) == 0){
echo "Array is empty";
}
Since our array has no elements, the count function will return 0
. The echo statement will run as the if condition is met, resulting in the output below.
Array is empty
Using the not (!
) Operator
This example involves using the not (!
) operator to check if an array is empty. Since an empty array evaluates to false, we can use the not operator to change it so it evaluates to true.
In the example below, we create an empty array named exampleArray
. In our if statement, we have a condition that uses the not operator to inverse the result of the empty array. So instead of the empty array evaluating to false, it will evaluate to true.
<?php
$exampleArray = [];
if(!$exampleArray){
echo "Array is empty";
}
As expected, our script above will output the following line since our array is empty. You can try adding an element into the array if you want to verify the if statement’s condition works correctly.
Array is empty
Conclusion
I hope you now have a good understanding of how you can check if an array is empty in PHP. We touched on several different methods of performing the check, and you can use any of them to get the result you require.
There is plenty more to learn about the PHP programming language. For example, I recommend checking out our tutorial on the basics of arrays within PHP. There are also plenty of functions that you can use to help manage arrays.
Please let us know if you notice a mistake or if an important topic is missing from this guide.