In this tutorial, we will be showing you how it is possible to generate an MD5 hash of a string within PHP.
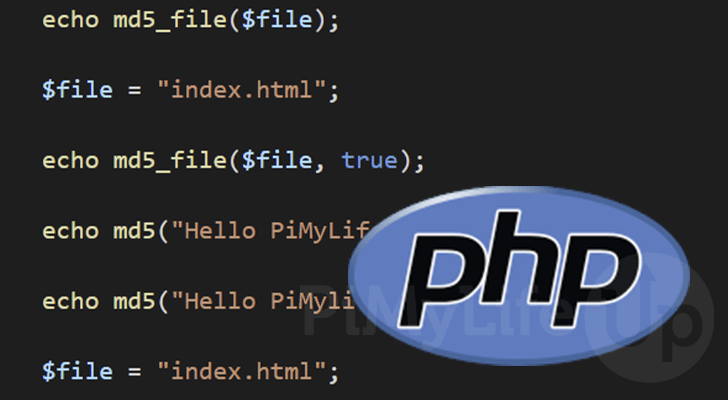
Before we get started, do not use MD5 when generating a hash for anything security-related, including passwords. This is because MD5 hashes can be generated incredibly fast on modern systems and are not considered cryptographically secure anymore.
While no longer helpful for security, MD5 still has its uses within PHP. While a fast hash is typically terrible for security reasons, it can still be helpful in many other cases.
One of these uses is to use it to verify the authenticity of a file. For example, a change in the hash indicates that the contents of a file are not identical.
Some public services even use an MD5 hash as a way of being able to pass in a string that should be somewhat private but not necessarily secure. A perfect example of one of these services is Gravatar’s popular global avatar system.
Gravatar’s avatar service utilizes an MD5 of the user’s email address to provide the avatar for that particular user. It allows a level of obfuscation and means the user’s email doesn’t have to be referenced within the URL.
Another example of where MD5 is still used by default is NGINX’s proxy cache. It uses an MD5 to generate a unique cache key for that request.
Over the next section, we will show you how you can generate an MD5 hash within PHP.
The md5() Function in PHP
PHP’s md5() function is incredibly straightforward to use. It only has two parameters that you have to worry about, and unless you want the binary data, the second is entirely optional.
If you want to generate the MD5 hash of a file, there is a different function called “md5_file()
” that we will cover later on.
Below you can see the syntax for the md5()
function within PHP.
md5(string $string, bool $binary = false): string
The first parameter ($string
) is where you will specify the data that you want PHP to generate the MD5 hash for. This data must be passed in as a PHP string.
The second parameter ($binary
) allows you to control whether the hash is returned as a 32-character hex string (false
) or whether to return the raw 16-bit binary (true
). This parameter is optional, and in most cases, won’t be needed.
Example of Returning an MD5 Hex String in PHP
In this example, we will use PHP to generate an MD5 hash of the string “Hello PiMyLIfeUp Viewer
“. Since we want the hex format, all we need to do is pass this string in the first parameter.
To show you the generated hash, we use PHP’s built-in echo function to output the result.
<?php
echo md5("Hello PiMyLifeUp Viewer");
?>
Using this little code snippet, you should end up with the following hash. As long as you use the same string, these should always remain the same.
977715e903c512d3ac436332cb50282d
Example of Getting the MD5 Binary Format
Like our previous example, we will again be using MD5 to generate a hash.
We will be using the same string, “Hello PiMyLifeUpViewer
“, but this time we will also be enabling the “binary” format by passing in “true
” to the second parameter.
By setting the second parameter to true, PHP’s md5()
function will return the hash as a raw 16-bit piece of binary.
<?php
echo md5("Hello PiMylifeUp Viewer", true);
?>
After running the above example, you should now have the MD5 hash displayed in its binary format. As shown below, this will look like a weird jumble of characters when displayed directly on the screen.
Typically most people will want to use the hex format and not the binary format.
�w��ӬCc2�P(-
Generating an MD5 Hash of a File in PHP
PHP has an in-built function that allows you to easily generate an MD5 hash of a file. This function is called “md5_file
“.
Using this function is relatively similar to the base md5()
function. However, the first parameter has changed.
Instead of specifying the string you want to be hashed, you will specify the path to a file you want PHP to generate the MD5 hash for.
The function definition for PHP’s md5_file function is as we have shown it below.
md5_file(string $filename, bool $binary = false): string|false
The “$filename
” parameter is where you must specify the path to the file you want to generate the MD5 hash of. Remember that this path must either be relative to the current PHP files location or be an absolute path.
The $binary
parameter allows you to control whether PHP returns the hash as a 32-character hex string (false
) or a 16-bit binary (true
). By default, this is set to false
, and it will suit most use cases.
On success, the md5_file function will return a string containing either the 32-character hex string or the 16 bits of binary. This differs depending on what you set for the “$binary
” parameter.
On failure, PHP will return a Boolean, false
. A failure typically occurs when PHP can’t access the specified file.
Example of Generating the MD5 Hash of a File using the Hex Format
For this example, we will be using PHP to generate the MD5 hash of our “index.html
” file that sits alongside our PHP script. We will store this filename in a variable called “$file
“.
We then utilize the md5_file function, passing the path to our file stored in the “$file
” variable into the first parameter.
The MD5 hash of the file will then be printed by PHP to the output, thanks to the echo keyword.
<?php
$file = "index.html";
echo md5_file($file);
?>
After running this code PHP, will have generated the hash for the file located in the same directory with “index.html
“.
The MD5 hash generated by PHP will be output. For our example file, we retrieved the following 32-character hex hash.
618c0ed040859c802890a570c283d201
Example of Retrieving the MD5 Hash of a File using the Binary Format
With this example, we will generate an MD5 hash for our file and have it output in its binary format.
Start this example by creating a variable called “$file
” and assigning it the path to your file. In our case, we will be using “index.html
“, which sits alongside our test PHP script.
Finally, we make a call to the md5_file()
function. We pass in the variable containing the path to our file in the first parameter.
The second parameter we set to true
. By setting the second parameter to true, we tell md5_file() to output the hash in binary format instead of hex.
The result of this hash will be output. As this is the binary format, the resulting hash might look like a weird jumble of symbols when displayed on your screen.
<?php
$file = "index.html";
echo md5_file($file, true);
?>
Below is an example of the binary output for our example file. You will likely want to stick with the hexadecimal string for most use cases.
a��@���(��p�
Conclusion
Throughout this tutorial, we have shown you how to generate an MD5 hash within PHP. While MD5 is basically useless for security, it still has various use cases.
PHP comes built-in with two functions that allow you to quickly generate an MD5 hash for either a string or file.
Please comment below if you have had any issues using either one of those functions.
Be sure to check out our many other PHP tutorials and our other coding guides.