In this guide, we will be showing you how you can check if a string is a number in PHP using is_numeric().
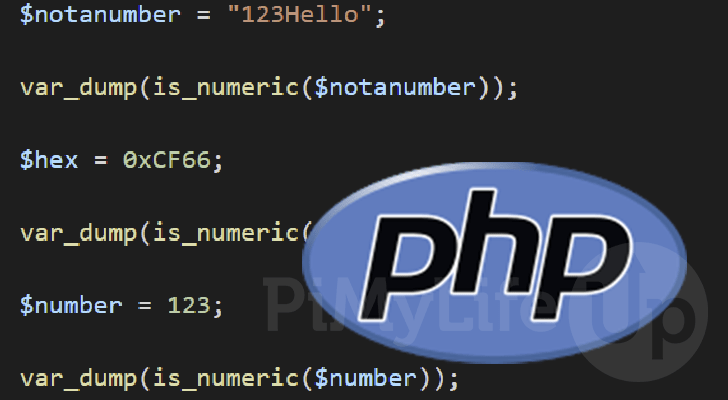
The is_numeric() function in PHP is incredibly straightforward to use and understand.
This function gives you a simple way to check whether the passed in variable is a number, or numeric string. However, a string that contains a floating-point number that uses a comma (,) instead of a dot (.) will fail this test.
You will find that the is_numeric() function is very useful when dealing with user input. For example, verifying that a user typed in a number into an input box.
Over the next section, we will show you how to use the is_numeric() function within your PHP scripts.
Definition of the is_numeric() Function in PHP
Let us start by exploring how the is_numeric() function is defined within PHP. It is a rather simplistic function that only has a single parameter.
The definition below shows you the function, the parameter, its expected variable type, and the variable type the function will return.
is_numeric(mixed $value): bool
Copy
The single parameter this function has takes in the value you want to check if it is numeric. In addition, this function will return a Boolean value (true or false).
If the value is a number or a numeric string, the function will return true
. Otherwise, this function will return false
.
How to use the is_numeric() Function in PHP
Now that we know the definition of the is_numeric() function within PHP let us explore how to put this function to use.
To showcase how this works with different values, let us run through several different examples. Each of these examples will show you how this function works.
The is_numeric() Function on a Number
For this first example, let us see the result of this function when it is used on a standard number. The expected result from this will be for PHP’s is_numeric() function to return true
.
We start this example by creating a variable called “$number
” and assigning it the number 123
.
Next, we utilize the is_numeric()
function to check if the “$number
” variable is numeric. We print this result by passing it directly into PHP’s var_dump() function.
The var_dump() function will show us the value that is returned.
<?php
$number = 123;
var_dump(is_numeric($number));
?>
Copy
After running this code snippet, you should have the following result. Since we passed in a number, we got back “true
“.
bool(true)
Checking if a Numeric String is a Number
For the second example, we will be showing how PHP’s is_numeric() can check whether a string contains a number. Again, we pass in a value that we know will cause the function to return true
.
The script is started with the creation of the “$number
” variable and its assignment with the string "1.25"
.
We then use the is_numeric()
function to check whether our “$number
” variable is, in fact, a numeric string. This is printed by wrapping the function call in “var_dump()
“.
<?php
$number = "1.25";
var_dump(is_numeric($number));
?>
Copy
The above code sample should end up printing the following value. Since the string we provided can be evaluated into a number.
bool(true)
is_numeric() with a Non-Number
Now that we have shown how PHP’s is_numeric() function works on a numeric string and a number let us pass in an invalid value.
We create a variable called “$notanumber
” and assign it the string "123Hello"
. Even though this string has a number, it is not a numeric string.
Next, we use the “is_numeric()
” function to check and return whether the “$notanumber
” string is numeric.
<?php
$notanumber = "123Hello";
var_dump(is_numeric($notanumber));
?>
Copy
Since our string contains elements that PHP can’t evaluate into a number, this function will return false
.
bool(false)
is_numeric() Evaluates All Number Types
PHP’s is_numeric() function can evaluate more than just integer and floating-point numbers.
For example, if your variable contains a hexadecimal number such as “0xcf66
” or a binary number like “0b10101
” it will still be evaluated as “numeric”.
To showcase this, let us write a quick PHP example to test how the is_numeric() function handles the number 0xCF66
.
Start this PHP script by creating a variable called “$hex
” and assign it the value 0xCF66
.
Like our previous examples, we use the “is_numeric()
” function to check whether its numeric, and use “var_dump()
” to print the resulting value.
<?php
$hex = 0xCF66;
var_dump(is_numeric($hex));
?>
Copy
Below you can see how the is_numeric() function evaluated our hexadecimal number as numeric.
bool(true)
Conclusion
In this tutorial, we showed you how you could utilize the is_numeric() function in the PHP language.
This function easily checks whether the given variable contains a number or a numeric string.
If you have any questions about using this function, please comment below.
To learn more check, out our numerous PHP tutorials, or if you want to learn a different language, browse our other coding guides.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support