In this tutorial, we will be showing you how to use the implode function in PHP.
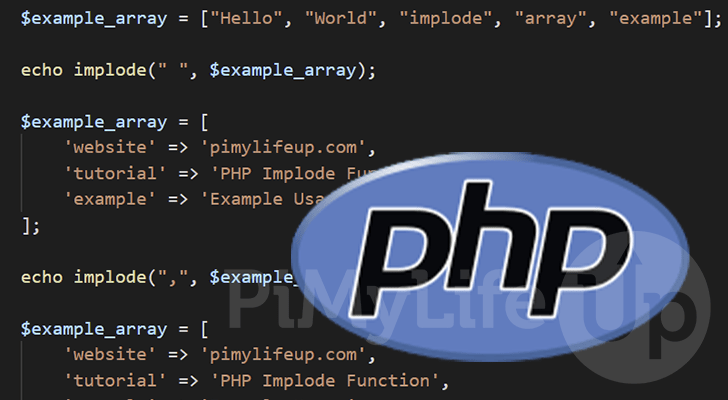
In PHP, the implode function allows you to take an array and convert it to a string.
The function achieves this by joining each array element together. In addition, it features a parameter that allows you to control the separator used between each element.
Using PHP to implode an array into a string can be helpful in several cases. One of these such cases is using this function to build a comma-separated list from your array.
For example, you could store data as an array but have it presented as an editable, comma-separated list in a textbox. You can then build it back into an array by using the explode function when you save this data.
Over the following few sections, we will be showing you how to use the implode function in PHP.
Syntax of PHP’s implode Function
The implode function’s definition is relatively simple and only has two parameters that you need to worry about.
Below you can see the definition of the implode function within PHP. This definition shows you the required data type for each parameter and its return type.
implode(string $seperator, array $array):string
Copy
Let us quickly go over the two parameters that this function has.
- The “
$seperator
” parameter allows you to specify a string that PHP will use to separate each array element. If you don’t want any spacing between elements, you can use an empty string (""
).
The value you pass to the first parameter must be a PHP string. - The second parameter (
$array
) is where you specify the array that you want to implode.
This function only works on flat arrays ([]
), it will not work on multi-dimensional arrays([ [ ] ]
).
The implode function returns a string that contains all of your array elements joined together.
If you pass in an empty array, PHP will return an empty string.
Utilizing the implode Function in PHP
Now that you understand how the implode function is defined in PHP. Let us explore some code examples that utilize this function.
Over the next few sections, we will show you the different behaviors of the implode function.
The Basic Usage of PHP’s implode() Function
Let us start these examples by focusing on the most basic usage of the implode function on PHP.
To start this example, we need to create an array that we will implode, converting it from an array to a string.
This array will be called “$example_array
” and contain the elements "Hello"
, "World"
, "implode"
, "array"
, and "example"
.
After this, we use the “implode()
” function to convert the array to a string. In the first parameter, we pass in our separator, which will be a string containing a single space(" "
).
In the second parameter, we will pass in the array assigned to our “$example_array
” variable.
Finally, we use PHP’s echo keyword to print the string returned by the implode()
function.
<?php
$example_array = ["Hello", "World", "implode", "array", "example"];
echo implode(" ", $example_array);
?>
Copy
Below is the output that you will get after running the above example.
You can see how the “implode()
” function joined each array element, using a single space as a separator.
Hello World implode array example
How the implode() Function Handles an Associative Array
PHP will strip out the array keys when an associative array is passed into the implode() function. This means PHP will join only the values of the array together.
While it is possible to work around this behavior, let us first show the default way the implode function will handle an associative array.
Default Handling of an Associative Array
To showcase this, we will create a simple associative array and fill it in with some example data.
You will use the “implode()
” function just like any other array, passing it into the first parameter. For our separator, we will pass in a single comma (,
).
We output the result of this function by using the echo keyword.
<?php
$example_array = [
'website' => 'pimylifeup.com',
'tutorial' => 'PHP Implode Function',
'example' => 'Example Usage'
];
echo implode(",", $example_array);
?>
Copy
Below we have shown the output from this PHP example.
You can see how the implode function stripped out the array keys and joined each value together, using a comma as the separator
pimylifeup.com,PHP Implode Function,Example Usage
Using implode() on an Arrays Keys
If you need to perform an implode on an array’s keys, there happens to be a way to work around the implode functions behavior.
We can achieve this by utilizing the “array_keys()
” function. This function will retrieve all keys from an array and return them as an array.
In this example, we use the same data as previously, except this time we utilize the “array_keys()
” function and pass in our example array. The result from this function will be stored in the “$array_keys
” variable.
We then implode the array of keys like we would any other array.
<?php
$example_array = [
'website' => 'pimylifeup.com',
'tutorial' => 'PHP Implode Function',
'example' => 'Example Usage'
];
$array_keys = array_keys($example_array);
echo implode(",", $array_keys);
?>
Copy
Below, you can see that the keys for our array were joined together and printed. A single comma separates each value (,
).
website,tutorial,example
Conclusion
Throughout this tutorial, we have shown you how to use the implode() function within PHP.
It allows you to convert an array into a string within PHP easily. This has many use cases, such as needing the array formatted as a specific string.
Please leave a comment below if you have any issues using the implode function in PHP.
Be sure also to check out our other PHP tutorials. We also have other coding guides if you want to learn a different language.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support