In this guide, we will be showing you how to use the explode function in PHP.
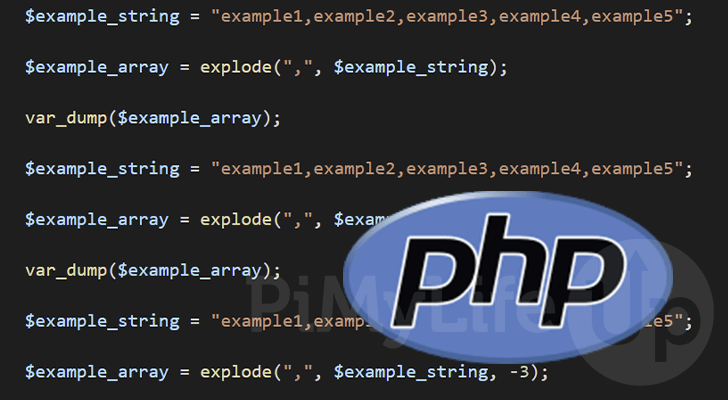
PHP’s built-in explode function lets you take a string and convert it to an array. It is the opposite of PHP’s implode() function that converts an array to a string.
This function achieves this by taking the string and using the specified separator to split the string. PHP will place each split piece of the string in a new element in the array.
You can even limit the number of times the explode function will split a string. This feature is useful when you only need a limited number of elements in your array.
The primary usage of the explode() function is to handle strings separated in a standardized way. For example, a CSV file uses commas to separate each value. You can convert the file into an easier-to-process array using the explode function.
Over the next section, we will show you the definition of PHP’s explode function and how to utilize it.
Definition of the explode() Function in PHP
In this section, let us explore how the explode function is defined within PHP.
This definition will help you see how exactly this function is used and what each of its parameters expects.
Below you can see the definition of the function. We will explore the parameters shortly.
explode(string $separator, string $string, int $limit = PHP_INT_MAX): array
From the above definition, you can see that the explode function has three parameters that you can use. The first two parameters are required, and the third is optional.
$separator
– The first parameter allows you to set the string that PHP will use to split the passed in string.
For example, if you were dealing with a CSV file, you would use “,
” as the separator.$string
– With the second parameter, you can specify the string you want to be split and converted to an array.
This string should use whatever you specified in the “$separator
” field to separate values.$limit
– The final field is optional but allows you to limit the number of separations performed on a string.
By default, this value is set to the constant “PHP_INT_MAX
“, this constant stores the maximum size of an integer.
If you set the limit to a positive value, the returned array will contain up to the defined amount. The last element will contain the rest of the string that wasn’t separated.
If you set this to a negative value, all elements except the last number specified will be returned.
When you set the limit to0
, PHP will treat this as if you used the value1
.
PHP’s explode function will return an array. This array will contain an array of strings, split using the specified separator.
Examples of using the explode() Function in PHP
In this section, we will explore how the explode() function is used within PHP and how it behaves under various conditions.
Basic Usage of the explode() Function
Let us show you the typical usage of the explode() function within PHP.
With this example, we will start with a simple string that uses a comma as a separator. The string that we will be setting to our “$example_string
” variable is "example1,example2,example3,example4,example5"
.
Our next step is to utilize the explode()
function. We pass a comma (,
) in as the first parameter as this is the separator we used in our string.
The second parameter we pass in is our “$example_string
” variable. This is the string that we will be exploding, converting it from a string to an array.
We will assign the result of the explode() function to the “$example_array
” variable.
Finally, we print out this array by utilizing PHP’s var_dump function. This function allows us to see the array and each element placed into it.
<?php
$example_string = "example1,example2,example3,example4,example5";
$example_array = explode(",", $example_string);
var_dump($example_array);
?>
With the result below, you can see how PHP converted our string to an array. Since we defined the comma (,
) as our separator, it was stripped as PHP built the array.
array(5) {
[0]=>
string(8) "example1"
[1]=>
string(8) "example2"
[2]=>
string(8) "example3"
[3]=>
string(8) "example4"
[4]=>
string(8) "example5"
}
Using the explode() Function with a Positive Limit
For this example, we will be showing you how the PHP explode() function handles a positive limit value.
We will be using the same example string as our previous example and assigning it to the variable called “$example_string
“. But this time we will be utilizing the “$limit
” parameter.
By setting this limit to 3
, the first two elements will be strings that PHP split out. The third element will contain the rest of the string that hasn’t been separated.
The resulting array that is stored in the “$example_array
” variable will be printed by the “var_dump()
” function.
<?php
$example_string = "example1,example2,example3,example4,example5";
$example_array = explode(",", $example_string, 3);
var_dump($example_array);
?>
With the result from this example code, you can see how PHP handles positive limits. The first two elements of the array contain split elements.
The third and final array element contains the rest of the string that wasn’t separated due to the “limit
“.
array(3) {
[0]=>
string(8) "example1"
[1]=>
string(8) "example2"
[2]=>
string(26) "example3,example4,example5"
}
How to use the PHP explode() Function with a Negative Limit
In this example, we will explore how the explode function will handle a negative limit.
Let us re-use the same basic code from our previous two examples. We set our “$example_string
” variable to "example1,example2,example3,example4,example5"
.
On the following line, we utilize the explode function. We set the first parameter to a comma (,
). Then in the next parameter, we pass in our “$example_string
” variable.
Finally, we set our limit to negative three (-3
). This will mean that explode()
will return an array containing all elements except the last 3
. Unlike a positive limit, you will not get an element containing the dropped parts of the string.
The result from the explode function will be stored in the “$example_array
” variable and then printed using “var_dump()
“.
<?php
$example_string = "example1,example2,example3,example4,example5";
$example_array = explode(",", $example_string, -3);
var_dump($example_array);
?>
Below you can see the array produced by our the explode() function in PHP. It converted our string to an array, leaving us with all but two elements.
array(2) {
[0]=>
string(8) "example1"
[1]=>
string(8) "example2"
}
Conclusion
Throughout this tutorial, we showed you how to use the explode() function within PHP.
This function is powerful and easily allows you to convert a string to an array. It passes the string, splitting it each time it runs into the specified separator.
Please comment below if you have any questions about using the explode() function.
If you want to find out more about this language, check out our many other PHP tutorials. We also have a wealth of other coding guides that you can explore.