In this tutorial, we will discuss how to use and write a foreach loop in PHP.
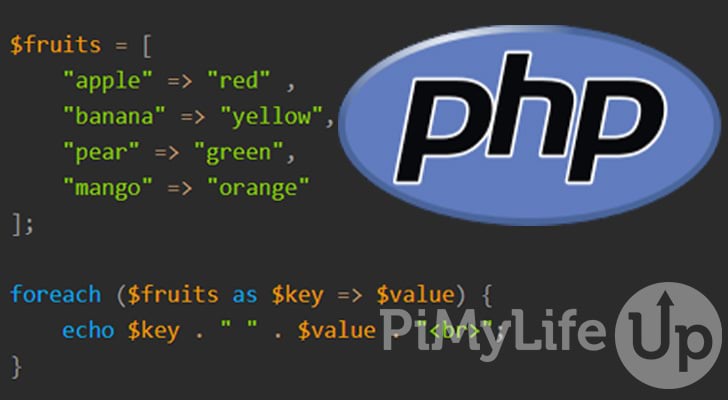
A foreach loop in PHP is perfect for looping through elements part of an indexed array or an associative array. The loop will start from the first element of the array and work through all the elements in order until it reaches the end. You can also iterate through objects.
There are a few reasons why you may pick a foreach loop over a regular for loop. For example, a foreach loop can be a bit easier to read and understand when dealing with arrays. You can also avoid using the count()
function. But, of course, there are many other reasons why you may want to pick one over the other.
You will likely need to use nested foreach loops when handling multidimensional arrays. However, you will be writing a single depth foreach loop most of the time.
This tutorial will take you through a wide range of topics on using a foreach loop in PHP. We touch on topics such as arrays, associative arrays, multidimensional arrays, editing elements, using the break statement, and using the continue statement.
Table of Contents
foreach Loop Syntax
There are two different ways you can write a foreach loop in PHP.
Our first example iterates through an array assigning the current element’s value to the variable $value
. You can use the $value
variable anywhere within the foreach code block.
foreach ($array as $value) {
//code to execute
}
Copy
Our second example is for associative arrays, which will contain a range of keys. On every iteration, the current key will be stored in the $key
variable and the element value in the $value
variable.
foreach ($array as $key => $value) {
//code to execute
}
Copy
You can write a foreach loop using the alternative syntax. The example below shows the alternative syntax for our first example, but you can use it on either type of foreach loop.
foreach ($array as $value) :
//code to execute
endforeach;
Copy
If you need to edit array elements while in the loop, you can precede the $value
variable with &
. Using &
will ensure that the variable is assigned the reference to the value and not simply a copy of the value.
How to Use a foreach Loop in PHP
Foreach loops are the perfect way of iterating through data stored in an array. Unlike a regular for loop, you do not need to know the array’s length as the foreach loop will continue until it reaches the last element. You can use break or continue if you need to exit the loop early or skip an iteration.
Below we go through all the main features of handling a foreach loop, including writing a foreach statement for both a regular array and an associative array.
A foreach Loop for an Indexed Array
In this example, we create a simple PHP foreach loop that will iterate through an array containing a range of strings.
On every iteration, the value of the current element is copied to the $value
variable. Inside the loop code block, you can use the value how you see fit by referencing the $value
variable.
The code below simply iterates through our array and outputs each value using echo.
<?php
$fruits = ["apple", "banana", "pear", "mango"];
foreach ($fruits as $value) {
echo $value . "<br>";
}
?>
Copy
Below is the output from the above code. As you can see, each array element has been printed in order.
apple
banana
pear
mango
A foreach Loop for an Associative Array
If you are using an associative array, the method above will still work; however, you will only have access to the value of each element. To also have the key value available, you must specify it within the foreach statement.
The example below will have the key value available in the $key
variable and the element value available in the $value
variable. Each iteration will progress to the next element until it reaches the end of the array.
<?php
$fruits = [
"apple" => "red" ,
"banana" => "yellow",
"pear" => "green",
"mango" => "orange"
];
foreach ($fruits as $key => $value) {
echo $key . " " . $value . "<br>";
}
?>
Copy
In the output below, you can see the key value followed by the element value for each element in the array.
red apple
yellow banana
green pear
orange mango
A foreach Loop for a Multidimensional Array
If you have a multidimensional array, you will need to nest a foreach loop inside another foreach loop to access the elements of the inner arrays. The outer loop will iterate through each of the arrays, while the inner loop will iterate through each array’s elements.
You can use foreach on very deep multidimensional arrays, but keep in mind that performance and readability will begin to suffer. The most popular types are two-dimensional arrays and three-dimensional arrays. Anything deeper than three is not very common as they become hard to manage.
In our example below, our outer loop will cycle through each array stored in our $fruits
array. The inner loop will cycle through each element of the current array.
<?php
$fruits = [
["apple", "red"],
["banana", "yellow"],
["pear", "green"],
["mango", "orange"]
];
foreach ($fruits as $value) {
foreach ($value as $value2) {
echo $value2 . "<br>";
}
}
?>
Copy
The output showcases how our nested foreach loop works in the code example above. Each element is printed before it moves onto the next array. $value
is the current array, and $value2
is the current element inside our $value
array.
apple
red
banana
yellow
pear
green
mango
orange
Editing Values within a foreach Loop
If you try editing values using a PHP foreach loop, you will need to add &
to the start of the $value
variable. Using &
before the $value
variable will tell PHP to assign $value
a reference to the original value and not use a copy of the variable value.
We pass in the reference rather than the value in the example below. Using the reference will allow us to edit the array elements inside the foreach loop.
We also unset
the $value
after we exit the loop. If you do not unset the $value
variable, it will still contain the reference to our last item. If you reuse $value
without first using unset, you may end up with unexpected behavior.
<?php
$num = [1, 2, 3, 4];
echo "Using the reference (&) <br>";
print_r($num);
foreach ($num as &$value) {
$value += 1;
}
unset($value);
echo "<br>";
print_r($num);
?>
Copy
In the output below, you can see that the array’s values have been altered.
Using the reference (&)
Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 )
Array ( [0] => 2 [1] => 3 [2] => 4 [3] => 5 )
In our example below, we do not use the reference and simply use a copy of the array’s element value. PHP will not keep any alterations if you use the element’s value.
<?php
$num = [1, 2, 3, 4];
echo "Using the Value <br>";
print_r($num);
foreach ($num as $value) {
$value += 1;
}
echo "<br>";
print_r($num);
?>
Copy
As you can see in the output, none of our changes to the array are made, and the array appears untouched outside the foreach loop.
Using the Value
Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 )
Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 )
Breaking Out of a foreach Loop
Like most other PHP loops, you can use the break statement to end the loop early. You may want to end the loop for various reasons. For example, you found the data you were looking for and performed the required changes.
In our example below, we exit the foreach loop when our value equals “banana”.
<?php
$fruits = ["apple", "banana", "pear", "mango"];
foreach ($fruits as $value) {
if($value == "banana")
break;
echo $value . "<br>";
}
?>
Copy
Since banana was our second element in the array, we only output the value of the first element in the array.
apple
Using continue in a foreach Loop
If you need to skip or end an iteration of the foreach loop early, you can use the continue statement. There are plenty of reasons why you may want to use continue. For example, skipping an iteration can be helpful if you do not need to process the data in that specific element.
In our code below, we end the current iteration early when the value equals “banana”.
<?php
$fruits = ["apple", "banana", "pear", "mango"];
foreach ($fruits as $value) {
if($value == "banana")
continue;
echo $value . "<br>";
}
?>
Copy
As you can see in our output below, we skipped outputting the value when it was equal to “banana”. After “banana”, we continued to output the rest of the values.
apple
pear
mango
Conclusion
By the end of this tutorial, I hope you have a good understanding of how to use a foreach loop within PHP. It is a standard loop that is great for handling data within arrays, so it is important that you understand how they work.
I suggest that you look into other loops if you are new to programming. Each loop has its use case, so I recommend learning about while loops, for loops, and do-while loops. Understanding and using them correctly can help improve the readability and performance of your code.
If we can improve this tutorial, please do not hesitate to let us know by leaving a comment below.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support