In this tutorial, we will teach you how to use the isset() function in PHP.
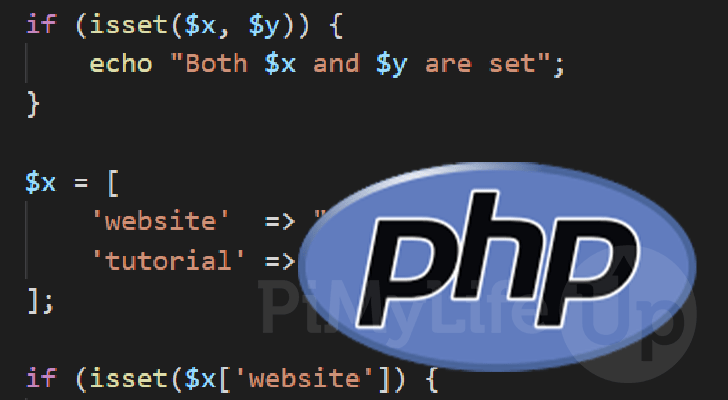
In PHP, isset() is one of the fundamental functions you should learn to utilize. It is a powerful function that lets you check whether a variable is declared and is not set to NULL.
The isset() function is especially useful to check if a key exists within an array. This is especially true when you rely on data from a third-party source where you can’t guarantee the values will exist.
This function works by checking PHP’s runtime memory to see if that particular variable exists. If the variable or array key exists, it then checks to see if that value is set to “null
“.
Over the following sections, we will show you how this function is defined and how to utilize it within your code.
Syntax of the isset() Function in PHP
Let us start by exploring the isset() function in PHP and how it is defined. Knowing the function’s syntax will make it much easier for you to utilize.
This function allows you to pass in as many variables as you want, but you must at least specify one. The isset() function will check each variable you specify to see if it is set.
Below you can see how the isset() function is defined in PHP. From this definition, you can see the expected data types of the parameters. Additionally, you can see that this function will return a Bool value (true
or false
)
isset(mixed $var, mixed ...$vars): bool
Let us start by going over the parameters that we can use to control the isset() function.
- This functions parameter is where you specify the variable that PHP will check exists. This variable can be any variable type, including an element within an array.
- PHP supports passing in multiple variables into the isset() function. You only need to specify each variable as a new parameter in the function,
Each variable passed in will have the same checks applied to it.
Now that we know what the parameters are let us explore the data that is returned by
The isset()
function will return true
when all variables passed into the function are declared, and their values are not null
.
If any of the variables you pass in are not declared, or their values are “null
“, PHP will return false
.
Table of isset() Return Values
Below you can see the sort of values that the isset() function will return depending on the passed in variable.
This table should give you a quick idea of what is considered to be “set” and what isn’t by PHP.
Expression | isset() Return |
---|---|
$x = “”; | true |
$x = []; | true |
$x = true; | true |
$x = null; | false |
$x is undefined | false |
Examples of using the isset() function in PHP
Now that we know how the isset() function is written in PHP ,let us explore some examples of how you can utilize it within your code.
Basic usage of the isset() Function
Let us start by exploring the most basic usage of the isset() function in PHP, and that is to check whether a single variable is set.
For this example, we will start by defining only a single variable. We will call this variable “x
” and assign it the string “PiMyLifeUp
“.
We use the isset() function within a conditional if statement, passing in the “$x
” variable into the parameter. Since we just declared this variable, we know PHP will echo the text "$x is set"
.
Our second conditional statement uses PHP’s isset()
function again. However, we are passing in a variable that hasn’t been declared previously this time.
Since this variable “is not set”, PHP will never print the text within this statement.
<?php
$x = "Hello";
if (isset($x)) {
echo "$x is set";
}
if (isset($y)) {
echo "$y is set";
}
?>
After running the code above, you should only end up with the following text. This text shows that we declared the “$x
” variable with a value that isn’t null.
The second if statement is never executed as the isset() function will return false
. This is because the “$y
” variable isn’t declared and therefore is not set.
Using isset() on Multiple Variables
For our second example, we will show you the isset() function takes multiple variables in PHP. This functionality is useful when you have multiple variables you need to be defined before running a block of code.
At the top of this example, we will declare a variable called “$x
” with the value “PiMyLifeUp
“.
Secondly, we declare a variable called “$y
” but will leave it unset by assigning it the value of null
.
Finally, we have our condition statement. We will use the isset() function to check if the “$x
” and “$y
” variables are declared.
<?php
$x = "PiMyLifeUp";
$y = null;
if (isset($x, $y)) {
echo "Both $x and $y are set";
}
?>
When using multiple variables with the isset() function, each one must be declared and have a value that is not null assigned to it.
While our “$x
” variable is considered set, our “$y
” variable is not so this example will print no text.
Using the isset() Function on an Array Key in PHP
For our last example, we will show you one of the best use cases for the isset() function in PHP. This use case is to use the isset() function to see if a key exists within an array.
Start the script by defining an associative array in PHP. For this example, we will have two elements, one called “website
” and one called “tutorial
“.
We then use the isset() function to check if our “$x
” array has a key named “website
” and has a valid value. If the value is set, we print the text “Website is
” followed by the value defined in the array.
After this, we have a second conditional statement that will use PHP’s isset() function to check whether the “published
” key is set.
If the “published
” key is set, we then echo the text “Tutorial published on
“, followed by the value stored in that key.
By using isset(), we can assure that we aren’t trying to access an element that doesn’t exist within the given array.
<?php
$x = [
'website' => "pimylifeup.com",
'tutorial' => "isset in PHP"
];
if (isset($x['website']) {
echo "Website is " . $['website'];
}
if (isset($x['published'])) {
echo 'Tutorial published on ' . $['published'];
}
?>
Using the code above, you will end up with the following result. The first isset() will return true since we defined the “website
” key within our “$x
” array.
The second conditional statement won’t run since the “published
” key is not declared within our “$x
” array meaning the isset() function will return false
.
Website is pimylifeup.com
Conclusion
In this tutorial, we have shown you how to use the isset() function within PHP.
This function is one of the most useful that you will use. It allows you to ensure that a variable is defined and has a value that is not null.
Please comment below if you have any questions about using the isset() function.
Be sure to check out our other PHP tutorials. Alternatively, we have many other programming guides if you want to learn a new language.