In this tutorial, we will go through how to create, edit, and delete array elements in the PHP programming language.
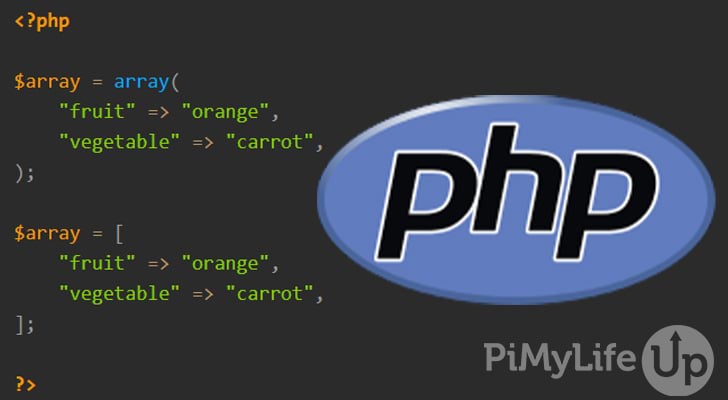
Arrays are among the most used data structures within programming languages such as PHP so understanding them is an absolute must. This tutorial takes you through many topics to help you learn the basics.
An array is excellent for storing multiple values inside a single variable. You can arrange the data, so it is easy to access using associative arrays. For more complex data, you can use arrays within arrays, also known as multidimensional arrays.
We will show you how to create, access, delete, and update arrays and their elements throughout this tutorial. Also, we discuss using these tasks with indexed, associative, and multidimensional arrays.
This tutorial assumes you are using PHP 8.0 or newer. There might be slight differences in behavior with older versions of PHP than what’s described in this tutorial.
Table of Contents
- Creating an Array in PHP
- Accessing Array Elements in PHP
- Adding Elements to an Array
- Updating Array Elements
- Removing Elements from an Array
- Conclusion
Creating an Array in PHP
There are a couple of different types of array that you can use in PHP. This section will cover how you can create an index array, associative array, and multidimensional array. Each of them has its pros and cons.
You can create a new array two different ways in PHP. First, the long syntax uses array()
. The second is the short syntax that uses []
.
Below is an example of both methods of creating an array in PHP.
<?php
$array = array(
"fruit" => "orange",
"vegetable" => "carrot",
);
$array = [
"fruit" => "orange",
"vegetable" => "carrot",
];
?>
Copy
Note: The short syntax for arrays is not supported in PHP versions earlier than 5.4.
Indexed Arrays
An indexed array uses integers for the location of elements. The count starts at 0 and increases by 1 for every element. So, for example, an array with 6 elements will have the first element at position 0 and the sixth element at position 5.
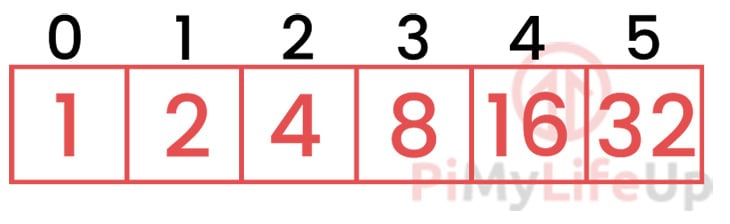
In the example below, we create a basic array that relies on an numeric index.
<?php
$array = [
"apple",
"orange",
"banana",
"mango",
"pear",
];
print_r($array);
?>
Copy
In our output below, you can see how the index for each element increments by 1.
Array
(
[0] => apple
[1] => orange
[2] => banana
[3] => mango
[4] => pear
)
Associative Arrays
An associative array uses keys rather than incremental integers to identify elements. Each value can be paired with a key, which can make accessing data easier when dealing with specific data.
In the example below, we create an associative array with different pieces of information as a key value pair.
<?php
$fruit = [
"type" => "Apple",
"healthy" => "Yes",
"calories" => 95,
"size" => "Medium",
];
print_r($fruit);
?>
Copy
The output below shows how our array is structured.
Array
(
[type] => Apple
[healthy] => Yes
[calories] => 95
[size] => Medium
)
Mixed Arrays
In PHP, you do not need to specify a key for every value. PHP will use a numeric index if you do not specify a key.
We do not include a key for the first and last element in the example below.
<?php
$fruit = [
"Apple",
"healthy" => "Yes",
"calories" => 95,
"Medium",
];
print_r($fruit);
?>
Copy
Since we did not specify a key for the first and last element, they are given numeric indexes. However, the integer indexes still increment by one, so our first element is 0, and our last element is 1.
Array
(
[0] => Apple
[healthy] => Yes
[calories] => 95
[1] => Medium
)
Multidimensional Arrays
A multidimensional array is an array of arrays and is often used to store data when a singular array is insufficient. For example, multidimensional arrays are extremely useful for data that you might usually represent in table form, such as timetables.
PHP allows deep multidimensional arrays, but it is recommended to keep them to only two or three levels deep. Therefore, you will most likely come across multidimensional arrays that are two or three levels deep. They are known as two-dimensional arrays or three-dimensional arrays.
The example below shows how to create a 2d array and a 3d array in PHP.
<?php
echo "2D Array";
$fruit = [
["Apple", "Red"],
["Banana", "Yellow"],
["Pear","Green"],
];
print_r($fruit);
echo "3D Array";
$fruitAndVeg = [
[
["Apple", "Red"],
["Banana", "Yellow"],
["Pear","Green"],
],
[
["Carrot", "Orange"],
["Lettuce", "Green"],
["Potato", "Yellow"],
],
];
print_r($fruitAndVeg);
?>
Copy
Below is the output from the print_r
function. We will go into more detail on accessing individual elements further in the tutorial.
2D Array
(
[0] => Array
(
[0] => Apple
[1] => Red
)
[1] => Array
(
[0] => Banana
[1] => Yellow
)
[2] => Array
(
[0] => Pear
[1] => Green
)
)
3D Array
(
[0] => Array
(
[0] => Array
(
[0] => Apple
[1] => Red
)
[1] => Array
(
[0] => Banana
[1] => Yellow
)
[2] => Array
(
[0] => Pear
[1] => Green
)
)
[1] => Array
(
[0] => Array
(
[0] => Carrot
[1] => Orange
)
[1] => Array
(
[0] => Lettuce
[1] => Green
)
[2] => Array
(
[0] => Potato
[1] => Yellow
)
)
)
Accessing Array Elements in PHP
The method of accessing arrays is roughly the same for each type of array. This section will touch on accessing index arrays, associative arrays, and multidimensional arrays.
Accessing Indexed Array Elements
As discussed in the previous section, indexed arrays work using integers as reference elements. The count starts at 0
and goes higher the longer the array. For example, if an array has 10
elements, the first element is 0
, and the last element is 9
.
In our example below, we access the elements within our array using the index values.
<?php
$fruit = ["Apples", "Oranges", "Pears"];
echo $fruit[0] . " and " . $fruit[2] . " are my favorites.";
?>
Copy
The code above will give the following output. Element 0
or fruit[0]
contains our string Apples, and element 2
or fruit[2]
contains our string Pears.
Apples and Pears are my favorites.
You can also loop through an index array by using a for loop. You will need to get the array length by using the count
function.
<?php
$fruit = ["Apples", "Oranges", "Pears"];
$arrayLength = count($fruit);
for($x = 0; $x < $arrayLength; $x++){
echo $fruit[$x] . "<br>";
}
?>
Copy
Accessing Associative Array Elements
If you are using an associative array, you will need to use keys to access elements within the array. However, if you have a mix of keys and numeric indexes, you will need to use an integer to access the elements that do not use a key.
In our example below, we demonstrate how to access specific elements using the key.
<?php
$fruit = [
"type" => "Apple",
"healthy" => "Yes",
"calories" => 95,
"size" => "Medium",
];
echo "A " . $fruit['size'] . " " . $fruit['type'] . " has ~" . $fruit['calories'] . " calories.";
?>
Copy
The following output is a result of running the code above. Each key referenced a string or integer stored within the array element.
A medium apple has ~95 calories.
Using a foreach loop, you can loop through the entire array, printing out the key, value, or both.
<?php
$fruit = [
"type" => "Apple",
"healthy" => "Yes",
"calories" => 95,
"size" => "Medium",
];
foreach($fruit as $key => $value) {
echo "Key: " . $key . " Value: " . $value . "<br>";
}
?>
Copy
Accessing Multidimensional Array Elements
Accessing elements within a multidimensional array is as simple as repeating the same thing you will do for a single layer array.
To access elements within a 2D array, you must point to two indexes. For example, $example[0][1]
will access the second element within the first array. A 3D array is similar, but uses three indexes $example[0][1][0]
.
You can make multidimensional arrays as deep as you want, but accessing the data could become very complex and messy. So most people stick to multidimensional arrays that are only two or three levels deep.
In our example below, we have a 2D array and a 3D array that we access several elements and output them.
<?php
$fruit = [
["Apple", "Red"],
["Banana", "Yellow"],
["Pear","Green"],
];
$fruitAndVeg = [
[
["Apple", "Red"],
["Banana", "Yellow"],
["Pear","Green"],
],
[
["Carrot", "Orange"],
["Lettuce", "Green"],
["Potato", "Yellow"],
],
];
echo "2D Array<br>";
echo "First Array, Element 1: " . $fruit[0][1] . "<br>";
echo "Third Array, Element 0: " . $fruit[2][0] . "<br>";
echo "3D Array<br>";
echo "First Array, First Inner Array, Element 1: " . $fruitAndVeg[0][0][1] . "<br>";
echo "Second Array, Third Inner Array, Element 0: " . $fruitAndVeg[1][2][0] . "<br>";
?>
Copy
Below is the output from the above code. You can quickly see how you can easily access multidimensional arrays and output specific elements.
2D Array
First Array, Element 1: Red
Third Array, Element 0: Pear
3D Array
First Array, First Inner Array, Element 1: Red
Second Array, Third Inner Array, Element 0: Potato
Adding Elements to an Array
This section will go through how to add new elements to an existing array. The process is straightforward in PHP and does not require helper functions or methods. However, you can use the array_push()
function.
Using Square Brackets
To add a new value to an array, you can simply assign it to the array. In our example below, we add three new elements to the array.
The first addition, we do not specify a key or index, so PHP will simply add it to the end of the array. Then, it can be accessed using an index.
Our second addition, uses an index that does not already exist. Since we are adding it with the value of 10, any new elements added afterward that don’t specify an index will start from 11.
Lastly, our third addition uses a key that does not already exist.
<?php
$array = [
"apple",
"orange",
"banana",
];
$array[] = "mango";
$array[10] = "pear";
$array["key"] = "cherry";
print_r($array);
?>
Copy
The output below shows our new elements in the array.
Array
(
[0] => apple
[1] => orange
[2] => banana
[3] => mango
[10] => pear
[key] => cherry
)
You can also use functions to add new elements to an array. For example, array_push()
is great for adding new elements into an indexed array. You can also use array_merge()
for merging two arrays or adding elements that use keys.
Updating Array Elements
Updating array elements is almost the same as adding new elements.
To update an array element, you will need to specify the index or key inside square brackets next to the array variable ($fruits[0]
). Next, we simply specify the value we wish to assign to the array element ($fruits[0] = "mango";
).
In our example below, we demonstrate how you can update an element using the index or a key. Multidimensional arrays are essentially the same method, but you may need to add more square brackets to edit the correct element.
<?php
$fruits = [
"apple",
"orange",
"banana",
];
$fruits[0] = "mango";
echo "Indexed ";
print_r($fruits);
$apple = [
"type" => "Apple",
"healthy" => "Yes",
"calories" => 95,
"size" => "Medium",
];
$apple["type"] = "Orange";
echo "Associative ";
print_r($apple);
?>
Copy
In the output below, you can see we have successfully updated the specified elements within the array.
In the first array, we changed element 0 from apple to mango. The second array, we updated the value assigned to the type key from Apple to Orange.
Indexed Array
(
[0] => mango
[1] => orange
[2] => banana
)
Associative Array
(
[type] => Orange
[healthy] => Yes
[calories] => 95
[size] => Medium
)
Removing Elements from an Array
The last topic we will touch on within this tutorial is removing certain elements from the array. If you do not know the index or key of the element you may need to use array_search()
or a for loop to find the element.
There are quite a few ways you can remove elements from an array. For this guide, we will touch on using unset()
, array_pop()
, and array_splice()
. However, you can use other functions such as array_diff()
, array_diff_key()
, and array_filter()
to remove elements as well.
Using unset()
Using unset()
to remove an element from an array is very straightforward. Specify the array and element you wish to delete as a parameter and it will remove it.
It is important to note that unset()
will not alter indexes. For example, if you remove an element in the middle of an index array, that index will simply no longer exist. If you wish to reindex the array, you may want to use array_values()
.
The example below demonstrates removing an element with unset()
and later using array_values()
to correct the index.
<?php
$fruits = [
"apple",
"orange",
"banana",
];
echo "Before Deletion ";
print_r($fruits);
unset($fruits[1]);
echo "After Deletion ";
print_r($fruits);
$fruits = array_values($fruits);
echo "After Reindexing ";
print_r($fruits);
?>
Copy
In the output below we can see the behavior of using unset()
. The behavior is the same if you were using string keys.
If you require the indexes to be numerically incremented by one, I highly recommend running array_values()
on the array. However, do not use it if you are using string keys.
Before Deletion
Array
(
[0] => apple
[1] => orange
[2] => banana
)
After Deletion
Array
(
[0] => apple
[2] => banana
)
After Reindexing
Array
(
[0] => apple
[1] => banana
)
Using array_pop()
If you require the last element to be removed from the array you can use the array_pop()
function. The function also returns the value of the last item so you can use it if required.
In the example below, we demonstrate how you can use the array_pop()
function.
<?php
$fruits = [
"apple",
"orange",
"banana",
];
echo "Before Deletion ";
print_r($fruits);
$last_element = array_pop($fruits);
echo "After Deletion ";
print_r($fruits);
echo "The last element was " . $last_element;
?>
Copy
In the output below you can see the last element was removed and stored in our variable $last_element
.
Before Deletion
Array
(
[0] => apple
[1] => orange
[2] => banana
)
After Deletion
Array
(
[0] => apple
[1] => orange
)
The last element was banana
Using array_splice()
We will briefly touch on using the array_splice()
function to delete elements from an array. You can use this function to perform different tasks, but for this tutorial we will keep it to deleting items.
Using array_splice()
will have all the keys reindexed whenever a change is made, unlike unset()
. It will also not alter associative keys, unlike array_values()
.
The array_splice()
function accepts four parameters, but we only need to use the first three. Below is a very basic explanation of each parameter.
- The first parameter is the array you wish to alter.
- Second parameter is the element you wish to use as a starting point for removing elements.
- The third parameter is the number of elements you wish to remove.
- Lastly, the fourth parameter we do not use, but it will accept a replacement array.
array_splice($array, $offset, $length, $replacement)
Copy
In our example below, we use the array_splice()
function to remove the second element (1
). We set the third parameter to 1
as we only want to remove one element.
<?php
$fruits = [
"apple",
"orange",
"banana",
];
echo "Before Deletion ";
print_r($fruits);
array_splice($fruits, 1, 1);
echo "After Deletion ";
print_r($fruits);
?>
Copy
As you can see in our output below, the element with an index of 1
was removed from the array. The array was also reindexed automatically.
Before Deletion Array
(
[0] => apple
[1] => orange
[2] => banana
)
After Deletion Array
(
[0] => apple
[1] => banana
)
Conclusion
I hope you now have a good understanding of all the basics of PHP arrays. They are an essential part of coding, so I highly recommend that you practice using them in code.
Arrays have a huge amount of helper functions that you can use to alter or access data quickly in the array. For example, you can use functions to merge, split, sort, and much more. These will be helpful, so I recommend learning some of them.
If we can improve this tutorial, please do not hesitate to let us know by leaving a comment below.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support