In this tutorial, we will take you through using the PHP header function to send raw HTTP headers.
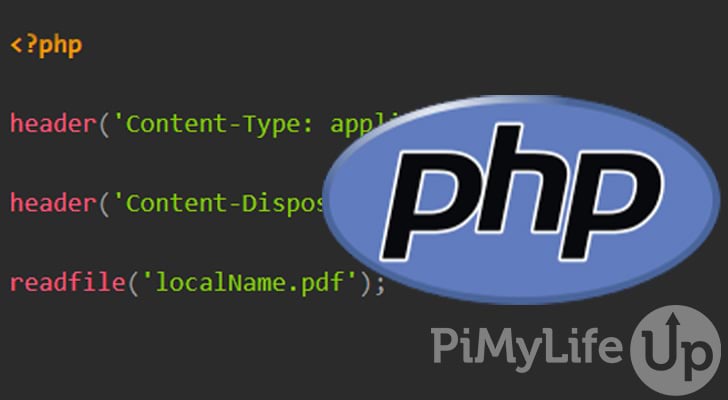
You can use the header function in PHP to set and send HTTP headers to the end-user. HTTP headers are useful as they allow both the client and server to pass information with a request or response. In addition, you can use these headers to control caching, specify a content type, redirect users, and much more.
There is a wide range of supported headers that you can use, so I recommend taking the time to learn them if you plan on becoming an expert in web development. We will cover a couple of examples in this tutorial.
This tutorial will take you through the syntax of the header function, a few examples of using the function, and a common error code associated with using the header function.
Syntax of the PHP header() Function
The header function accepts three parameters, but only the first parameter is required.
It is crucial that you use the header function before sending any output to the end-user. If you try using the function after output, you will likely see an error.
header(header, replace, http_response_code)
Header is where you specify the header string to send. This parameter is required and will result in an error if not specified.
There are two special headers that you can use with this function. I will quickly explain them below.
The first case involves using a header that starts with HTTP/
. You can use this to specify the status code to send. For example, HTTP/1.1 404 Not Found
will result in a 404 error page when the user hits the page.
The second case involves starting the header with location:
. After location, you can specify a redirection URL. For example, Location: https://google.com
will result in a 302 redirect to Google unless a 201 or 3XX status code has already been sent.
Replace is an optional parameter and defaults to TRUE if not specified. It indicates whether PHP should replace the header if it already exists. Specifying FALSE will allow multiple headers of the same type.
http_response_code is an optional parameter and can be used to force a specific response code.
Examples of using the header() Function
There are many different headers that you can set using the header function in PHP. In this section, we will take you through some of the different ways you can use headers.
Return a Specific Status Code
You can specify a specific status code to return as a header. There are many status codes that you can send to a browser. For example, the following example will send a 404 status code to the browser. Using $_SERVER["SERVER_PROTOCOL"]
, we can also ensure that the correct protocol is being used, for example HTTP/1.0
.
<?php
header($_SERVER["SERVER_PROTOCOL"]." 404 Not Found");
The example below is the response we received from our local web development server.

Download Files
In this example, we show you how to specify a file for a user to download.
We first indicate the content type, which is a PDF file. You can specify other content types such as images, audio, text, video, and other applications.
Secondly, we use a Content-Disposition
header to indicate the file should be downloaded rather than displayed in the web browser. Finally, in the same header, we specify the filename
, which will be the name of the file once it has been downloaded.
Lastly, we use the readfile
function to read a file and output it to the buffer. The parameter of this function should be the location and name of the file you would like available for download.
The code below is very basic, which you may want to expand upon if you wish to use it on a production server.
<?php
header('Content-Type: application/pdf');
header('Content-Disposition: attachment; filename="downloadName.pdf"');
readfile('localName.pdf');
The headers below are from our local web development server. A file was downloaded when we hit the PHP script.
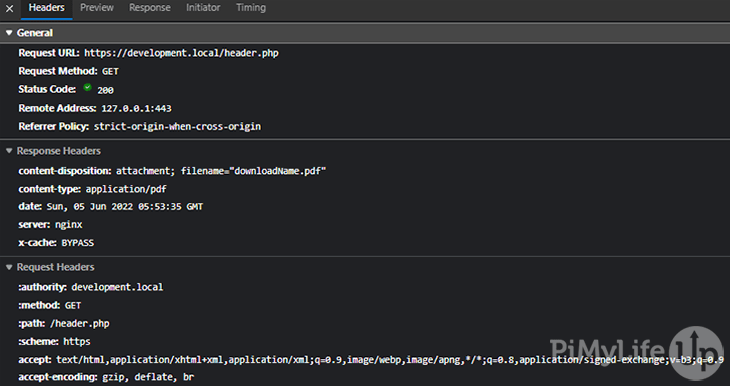
Caching Headers
Using headers to control caches is a valuable tool, especially if you are dealing with proxy or CDN caches. I recommend reading up on the different cache-control headers as there is quite a bit to cover.
The first header uses the Cache-Control
header with the no-cache
, no-store
, must-revalidate
directive.
no-cache
indicates the browser must validate the response with the origin server on each reuse. However, it allows the response to be stored in a cache.
no-store
indicates the response must not be cached at all.
must-revalidate
indicates that if the response becomes stale, it must be validated before you can use it again.
Our second header specifies a expires
header set to a date in the past. Having a past date means the response is always considered stale and thus is unlikely to be cached.
<?php
header("Cache-Control: no-cache, no-store, must-revalidate");
header("Expires: Wed, 11 Jan 1984 05:00:00 GMT");
Our browser can see that our cache-control and expires headers exist along with their data.
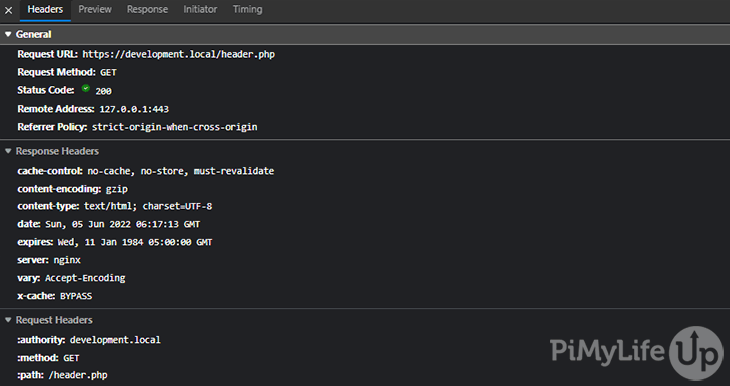
Redirect Users
Our last example is using headers to redirect the user to a different page. By default, the status code of using this header is 302 Temporary Redirect. However, you can change this by indicating a different code as a third parameter.
In the example below, we use the location header to redirect anyone who hits our PHP script to the Google search engine.
<?php
header("Location: https://google.com/");
exit;
As you can see in the screenshot below, our script redirected us to Google using the 302 temporary redirect status code.
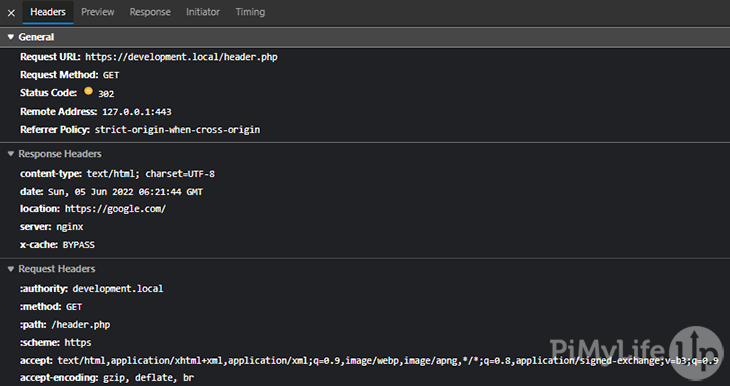
Headers Already Sent Error
You may receive the following error when you attempt to use the headers function. The warning indicates there is an issue somewhere within your PHP files. Diagnosing the issue can be straightforward or complicated.
Warning: Cannot modify header information - headers already sent by...
If you receive the above error, it means that data has been outputted and headers sent. The output may be intentional or unintentional. For example, warnings, error messages, or even whitespace outside PHP tags, can cause the headers to be sent prematurely. Functions such as print, echo, and other functions that output data can also cause headers to be sent.
The error message should contain enough information to locate the culprit of headers being sent early. The fix may be as easy as removing the offending code or moving your header logic before output begins.
Conclusion
I hope by now you have a basic understanding of how you can use the header function in PHP. We have covered a few useful examples and the most common error you may have with this function.
There are many more useful functions that you can use in PHP to help build a useful program. For example, you may also find functions such as sleep useful for controlling the execution of your scripts.
If we can improve this tutorial, please do not hesitate to let us know by leaving a comment below.