Within this tutorial, we will show you how to use the sleep or usleep function in PHP to pause the script.
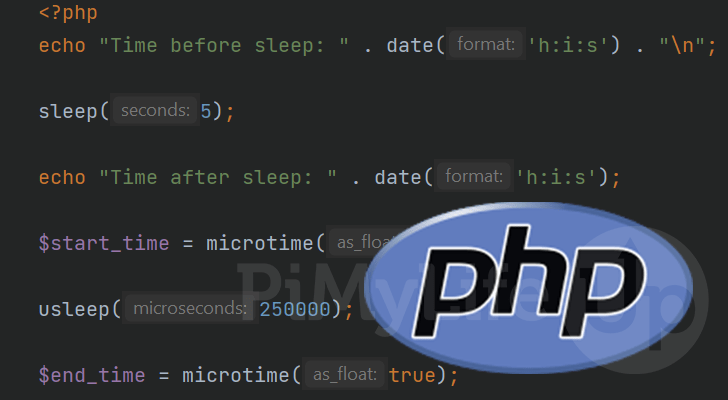
When writing your PHP scripts, there might be times when you feel like you will want to either pause/sleep the script.
Pausing the execution of a script is helpful in various scenarios. An example of usage is when performing a large number of network requests, you might want to sleep your PHP script to stop it from flooding the network.
You can also use it to prevent a script from consuming an abnormal amount of CPU resources. Using the sleep function between tasks can stop fast completing ones from quickly overwhelming a CPU.
The two main ways that you can sleep a script in PHP is to utilize either the “sleep()
” or “usleep()
“. Over the following sections, we will cover both of these.
A quick explanation between these is that the “sleep()
” function works in seconds, and “usleep()
” works in microseconds.
The PHP sleep() Function
Using PHP’s sleep function, it is possible to pause the execution of a script for a specified number of seconds.
The function only takes a single parameter called “$seconds
“, in which you set the sleep time in whole seconds.
Below you can see the definition for the sleep()
function within PHP. You can see its parameter is taken in as an integer and that it returns an integer.
sleep(int $seconds): int
Copy
The sleep() function will return zero (0
) when it successfully slept. However, if the sleeping of the script fails, it will return a Boolean false
.
As this function can return 0
or false
, make sure you use PHP’s identical operator (===
) when checking the return value. Otherwise, PHP’s type juggling will consider 0 and false to be the same.
Please note that the seconds can not be a fraction of a second. If you need a fraction of a second, use the usleep
function.
Example of Using the sleep() Function in PHP
Now that we know the syntax of the sleep() function let us write a quick PHP script so you can see how it works.
We will use PHP’s echo function to print the current time for this example. We can retrieve the current time thanks to the date()
function. Using the option “h:i:s
” will get the current hour, minute, and second.
We start by printing the string “Time before sleep:
“, concatenating the current time, and finally, the new line character (\n
).
We then use PHP’s sleep()
function to use, settings its single parameter to “5
” to sleep the script for 5 seconds.
Finally, to show you the script has sleep, we print the text “Time after sleep:
“, concatenating the current time.
<?php
echo "Time before sleep: " . date('h:i:s') . "\n";
sleep(5);
echo "Time after sleep: " . date('h:i:s');
?>
Copy
After running this script, you should see a result similar to what we have shown below.
With the time difference, you can see how the sleep function paused the execution of the script.
Time before sleep: 10:17:54
Time after sleep: 10:17:59
The usleep() Function in PHP
If you need to pause the execution of your PHP script for times shorter than a second, you will need to use the usleep() function.
With this function, you can specify the number of microseconds you want the script to sleep. A microsecond is one-millionth of a second.
For example, if you wanted to sleep your PHP script for a quarter of a second (0.25
), you would use the value 250000
.
Below, you can see the definition for the usleep() function within PHP. It has a single parameter that must always be an integer, and it returns an integer.
usleep(int $microseconds):int
Copy
Like the sleep function, usleep
will return 0
when it successfully slept. However, if the function fails to sleep for the whole time, it will return false
.
Please note that using micro sleeps longer than “1000000
” (1 second) may not be supported by the host system.
Example of Using the usleep() Function
For this example, we will use PHP’s usleep() function to sleep our script for 250000 microseconds (a quarter of a second).
We will need to use the time provided by the “microtime()
” function to track this. This provides us with the current Unix timestamp in microseconds.
Start the script by getting the current micro time and storing it in the “$start_time
” variable.
Then we can use the usleep()
function, passing in 250000
to sleep the script for 250000
microseconds.
After the sleep, we get the micro time again and store it in the “$end_time
” variable.
We then calculate the total execution time by subtracting the start time from the end time and storing it in the “$time
” variable.
Finally, we utilize the echo function to output how long the script took to run. This will show us how our script slept during execution.
<?php
$start_time = microtime(true);
usleep(250000);
$end_time = microtime(true);
$time = $end_time - $start_time;
echo $time;
?>
Copy
Below is an example of what PHP will print after running the above example.
You will notice that the time returned isn’t precisely the sleep time, as functions do not complete instantly.
0.25010299682617
Conclusion
Throughout this tutorial, we have shown you two ways that you can pause the execution of your PHP script.
The two functions we explored in this guide are PHP’s sleep()
, and usleep()
functions. Both have their particular uses but will pause the execution of the script.
Please comment below if you have any questions about using the sleep and usleep functions in PHP.
Be sure to check out our other PHP guides and our coding tutorials.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support