In this tutorial, you will learn how to use the substr() function in PHP.
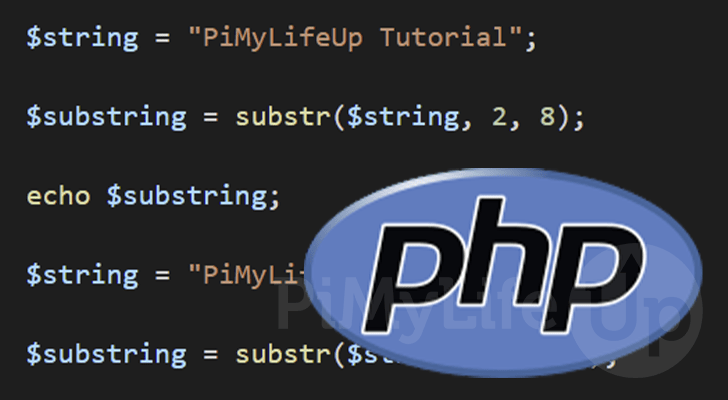
PHP’s substr() function allows you to take a string and only return a portion of it. In addition, the function gives you control over both the offset and the length of the string.
The string that this function returns is referred to as the substring. It has a wealth of uses within PHP as it allows you to cut up a string easily.
Over the following few sections, we will cover using the substr() function, and give some code examples.
Please note if you are using a string that contains Unicode characters, you should use the “mb_substr()” function instead.
Table of Contents
Definition of PHP’s substr() Function
Let us start by exploring how the substr() function is defined within PHP, as this will help you utilize it later.
With this definition, you can see each parameter and its expected variable type. Finally, it specifies that the substr() function will return a string.
substr(string $string, int $offset, ?int $length = null): string
Copy
Now that we have seen how the function is defined, we can explore each of the three parameters.
$string
– With the first parameter, you will specify the string you want to retrieve a substring from.
The returned value will be a subset of this string.$offset
– Theoffset
is the second parameter. This value defines the position in which PHP will start the substring.
If you set theoffset
to a negative value, the returned string will start from the end of the string, counting back by the specified value.
If theoffset
is longer than the string length, then an empty string will be returned by this function.$length
– The length parameter is entirely optional but is used to control the returned string’s length
When no length has been specified, the function will grab from the specified offset to the end of the string.
If thelength
is positive, then thesubstr()
function will only return up to the number of characters specified.
If thelength
is negative, then PHP will remove the number of characters specified from the end of the string.
If you set thelength
to zero (0
), then an empty string will be returned by this function.
In PHP, the substr()
function will return the extracted part of the string. If PHP can’t extract any string, PHP will return an empty string.
Please note that the offset value starts from 0. So, for example, with the string “PiMyLifeUp
“, the character “P
” is at position 0
.
Examples of Using the substr() Function in PHP
Now that we know how the substr() function is defined within PHP let us show you how you can utilize it to get a substring from a string.
In the following examples, we will explore the different ways that you can utilize this function.
Using the substr() Function in PHP with the Default Length
We will start these examples by showing you how PHP’s substr() function works when using the default length.
You utilize the default length by simply not using the third parameter of the substr()
function. Setting the third parameter to “null
” will also achieve the same thing.
This PHP script is started by creating a variable called “$string
” and assigning it a PHP string with the value "PiMyLifeUp Tutorial"
.
We then put the substr()
function to use by using the following parameters.
- In the first parameter, we pass in our “
$string
” variable.
The value passed in here is what PHP will generate the substring from. - By using the second parameter, we are setting the offset, which in this example will be set to
11
.
The offset is the position PHP will start the substring. - We will be excluding the third parameter entirely since we aren’t setting a length
With no length set, the substr() function will grab from position 11 to the end of the string.
The substring that is returned by this function will be stored in the variable “$substring
“.
Finally, we use PHP’s echo statement to output the value that is now stored in the “$substring
” variable.
<?php
$string = "PiMyLifeUp Tutorial";
$substring = substr($string, 11);
echo $substring;
?>
Copy
After running this PHP example, you should end up with the following substring.
Tutorial
Utilizing a Negative Offset with the substr() Function
By setting a negative offset, the substr() function will count backward from the last position in the string. So if you set a negative offset of 2 (-2), it will go forward from the second last character in the string.
To showcase this, let us create a variable called “$string
” and assign it the value "PiMyLifeUp Tutorial"
.
We use PHP’s substr() function to create a substring from our “$string” variable using the following parameters.
- For the first parameter, we pass in the string we want to grab a substring for.
In our example, this will be our “$string
” variable. - Now for the second parameter, we will be setting the offset.
The offset will be set to negative 8. Meaning the returned string will start from the 8th character counting backward.
Below you can see what our PHP example looks like.
<?php
$string = "PiMyLifeUp Tutorial";
$substring = substr($string, -8);
echo $substring;
?>
Copy
After running the above code example, you should end up with the following result.
Tutorial
Using a Positive Length with substr()
With this example, let us explore using a positive length with the substr() function in PHP. Since we are using a positive value, it will limit the output to the number of characters.
At the top of the script, we define the string we will be getting the substring of. We set our “$string
” variable to "PiMyLifeUp Tutorial"
.
Next, we utilize the substr() function, utilizing the following parameters.
- For the “string” parameter, we simply pass in our “
$string
” variable.
PHP will generate the substring from this string. - In the second parameter, we set the offset to “
2
“.
By setting this value to2
, we are grabbing from the third character in the string. - Finally, we set the length parameter to
8
.
Setting the length to 8 will limit the length of the substring to eight characters.
The resulting substring will be stored within our variable “$substring
“.
Finally, we output the result of our substr()
function call by using the “echo
” keyword.
<?php
$string = "PiMyLifeUp Tutorial";
$substring = substr($string, 2, 8);
echo $substring;
?>
Copy
Below you can see the substring that PHP generated from the usage of the substr() function.
MyLifeUp
Setting a Negative Length with the substr() Function in PHP
This section, will show you what happens when you set a negative length with PHP’s substr() function.
By setting a negative length, the substr() function will get the rest of the string from the offset, then omit the last number of characters.
Like our previous examples, we start our script by creating a variable called “$string
” and setting the value to "PiMyLifeUp Tutorial"
.
We then utilize the substr() function in our PHP example, using the following parameters.
- In the first parameter, we pass in the “
$string
” variable.
The substring will be created from this passed-in string. - For the second parameter, we are set our offset.
The offset for this example will be11
. This should be the position just after the space in our example string. - Finally, we set the limit to negative 5 (
-5
).
Setting this to negative, it will reduce the resulting substring starting from the end.
We store the result of our call to substr()
in our “$substring
” variable then output it by using the echo statement.
<?php
$string = "PiMyLifeUp Tutorial";
$substring = substr($string, 11, -5);
echo $substring;
?>
Copy
The PHP example above will produce the following substring. First, grabbing the string from position 11, then reducing the result by 5 characters.
Tut
Conclusion
Throughout this tutorial, we have shown you how to use the substr() function in PHP.
This function allows you to easily grab a section of a string by specifying a specific offset.
If you have any questions about using substr(), please comment below.
Be sure to check out our many other PHP tutorials or our other coding guides.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support