In this tutorial, we will discuss how to use and write a for loop in PHP.
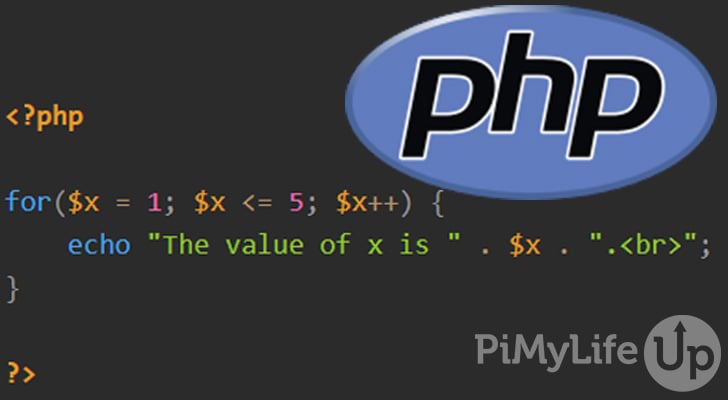
The for loop is an essential control structure within the PHP programing language. The syntax is roughly the same as most programming languages and is relatively easy to understand.
A for loop is perfect when you know how many times you want to loop through a code block. Alternatively, you can use a foreach loop instead of a regular for loop if you wish to iterate through an array or object.
Unlike a while loop, a for loop accepts three parameters. The first parameter is the creation of our counter variable. The second parameter is the condition. The last parameter is the amount to increase or decrease our counter value on each iteration. As long as the condition is true, our loop will continue to iterate.
Like every type of loop, you can nest for loops as many times as you want. However, it is good practice to keep nested loops to a minimum as they can become hard to understand and be performance heavy if you’re processing a large dataset.
Table of Contents
for Loop Syntax
The syntax for a for
loop in PHP is straightforward and roughly the same as for
loops in most other programming languages.
A for loop code block starts with for
followed by three parameters separated by semicolons and enclosed in parenthesis. The loop code block itself will need to be enclosed with curly brackets.
for (initialize; condition; increment) {
// Code here will be executed on each iteration
}
- Initialize is where you create the counter variable and assign it a value.
- Condition is the condition that is evaluated on every loop. If the condition equals true, it will continue to loop. Otherwise, if it is false, it will stop.
- Increment is for increasing or decreasing the counter value on every iteration.
How to Use a for Loop in PHP
In this tutorial, we go through the basics of writing a valid for loop within the PHP programming language. We will also cover topics such as skipping an iteration or breaking out of the loop entirely.
I highly recommend that you take a bit of time to learn PHP operators as you will need to understand these to build conditions. Conditional statements are used in various programming control structures, such as if-else statements, switch statements, loops, and more.
Writing a Standard for Loop
In this example, we will write a basic for loop to demonstrate how they work in PHP. I will quickly go through each piece of the code in the examples below.
Example 1
We have three parameters in our for loop parenthesis that I will quickly explain.
$x = 1
initializes the loop counter variable and sets its value to1
.$x <= 5
is the condition that states continue to loop for as long as$x
is equal to or less than5
.$x++
will increment our counter variable$x
by1
on every iteration.
Inside our for loop code block, we simply echo the value of x
on every iteration.
<?php
for($x = 1; $x <= 5; $x++) {
echo "The value of x is " . $x . ".<br>";
}
?>
Below is the output from the above code. As you can see, our loop continued to iterate until $x
no longer matched the condition because it was greater than 5
.
The value of x is 1.
The value of x is 2.
The value of x is 3.
The value of x is 4.
The value of x is 5.
Example 2
In this example, we decrement our counter variable and use larger values.
$y = 100
initializes our counter variable and sets the value to100
.$y >= 100
is the condition that states continue to loop for as long as$y
is equal to or greater than50
.$y -= 10
will decrement our counter variable$y
by10
on every iteration.
On every iteration, we echo the value of $y
along with the line break HTML element.
<?php
for($y = 100; $y >= 50; $y -= 10) {
echo "The value of y is " . $y . ".<br>";
}
?>
The output below shows that each loop iteration decrements our variable $y
. Once $y
no longer matched the condition of it being greater or equal to 50
, the loop is completed.
The value of y is 100.
The value of y is 90.
The value of y is 80.
The value of y is 70.
The value of y is 60.
The value of y is 50.
Breaking Out of a for Loop
Breaking out of a for loop is the same as every loop with PHP. All you need to do is use the break statement. You will need to place the break
statement inside a conditional block, so it is only run when certain requirements are met.
In our example below, we loop through until $z
equals 3
. Once the condition is met, we hit our break statement and exit out of the loop.
<?php
for($z = 1; $z <= 3; $z++) {
if($z == 3) {
echo "Breaking out of the script.";
break;
}
echo "The value of z is " . $z . ".<br>";
}
?>
The output clearly demonstrates the behavior of using the break statement. Once $z
was equal to 3
, the loop exited, and PHP reached the end of our script.
The value of z is 1.
The value of z is 2.
Breaking out of the script.
Using continue Inside a for Loop
You can end an iteration of the for loop early by using the continue
statement. Skipping an iteration may be important in cases where you do not want a particular dataset processed, and it’s safe to proceed to the next iteration.
In our example below, we will skip the iteration whenever $x
equals 3
.
<?php
for($x = 1; $x <= 5; $x++) {
if($x == 3) {
echo "Skipping.<br>";
continue;
}
echo "The value of x is " . $x . ".<br>";
}
?>
As you can see in the output below, when $x
was equal to 3
, we printed “skipping” instead of the value of $x
. The for loop continued to iterate until it no longer matched the condition within the for loops parenthesis.
The value of x is 1.
The value of x is 2.
Skipping.
The value of x is 4.
The value of x is 5.
Conclusion
You will likely find yourself using for loops a lot in PHP programming, so they are incredibly important to understand. I hope that this guide has been able to teach all the basics on a for loop and that you can now read and write them.
The for loop is not the only one you can use within PHP. Other loops, such as a while loop or foreach loops, are extremely useful but have pros and cons. I highly recommend that you take the time to learn as many control structures as possible as they may help drastically improve your code’s readability and performance.
If we can improve this tutorial, please do not hesitate to let us know by leaving a comment below.