In this guide, we will be showing you how to use the var_dump function within the PHP language.
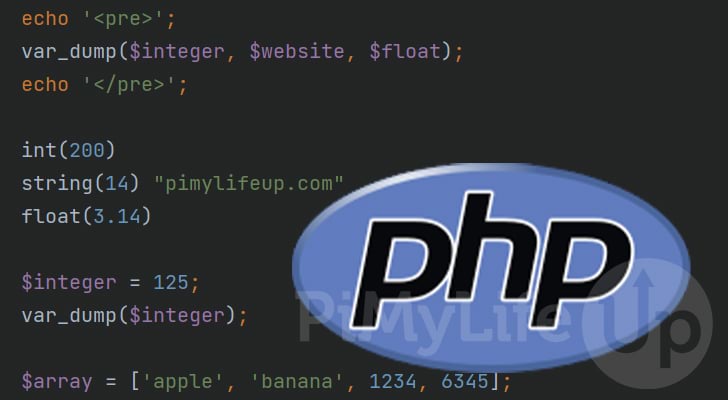
The var_dump()
function is incredibly useful for debugging variables. The function dumps all information you may want to know about a variable.
After passing a variable into var_dump
, it will output the type of the variable and the value stored within it. It is a better alternative to using PHP’s built-in print or echo when debugging the value of a variable.
Within this guide, we will show you how to use the var_dump
function in PHP, and give examples of how to best use the function.
Syntax of the PHP var_dump Function
The var_dump
function in PHP is incredibly straightforward and has a simple syntax.
Looking at the syntax below, you can see that the var_dump
function has two parameters.
The first parameter is required and is where you will specify your first variable.
The second parameter is optional, but allows you to input any additional variables you want to dump, make sure you separate each one with a comma (,
).
var_dump($VARIABLE, ...$VARIBLES);
This function will handle all the grunt work of getting information about your PHP variables. It will print out both the value and type of the variable.
When used on an array, the “var_dump
” function will run through it, printing the key, type, and value of each element within the array.
Preserving Output from var_dump()
To preserve newlines within var_dump
‘s output, you will need to wrap it in pre
tags, as shown below.
The pre
tag tells the web browser that this is preformatted text and should be presented as it is written.
echo '<pre>';
var_dump($VARIABLE);
echo '</pre>';
Examples of Using var_dump in PHP
Within the following examples, we will show you how the var_dump function within PHP treats a variety of variables types.
While these examples are relatively simple, they will give you an idea of the data var_dump
outputs.
Using var_dump on a Number
For our first example, we will create a simple variable called “integer
” and store the number “125
” in it.
We will then simply use var_dump
to print the type and value of the variable after it has been assigned.
<?php
$integer = 125;
var_dump($integer);
If you were to load this bit of code up in your web browser, you will see that it would output the following.
int(125)
You can see that PHP printed out the type of our variables as int
(Short for integer), and its value, which was set to 125
.
When using PHP’s var_dump
function on a number, PHP will write the value within the brackets of the data type. For example, int(VALUE)
or float(VALUE)
.
Outputting the Type and Value of a String
PHP’s var_dump function outputs its data slightly differently for PHP’s string data type. We will show this behavior by creating a string called “website
” and storing the value “pimylifeup.com
” in it.
After our string variable has been created we will pass it into the var_dump
functions parameter.
<?php
$website = "pimylifeup.com";
var_dump($website);
After running this bit of code, you will see the variables type and value output within the browser.
string(14) "pimylifeup.com"
First, you see the the type of the variable, which in this case is “string
“. Then, within the brackets next to the type, you can see the length of the string, which in our case is 14
characters.
After that, the string itself is output between the two double-quotes. Typically var_dump
will use the following format when used on a string variable: string(LENGTH) "STRING VALUE"
.
Example of using PHP’s var_dump Function on an Array
For this example, we will show you how the var_dump
function in PHP handles arrays.
Within this array, we will be using mixed types. The first two values will be strings. The last two will be integers.
To make the output from var_dump
easier to read, we will wrap the function in “pre
” tags.
<?php
$array = ['apple', 'banana', 1234, 6345];
echo '<pre>';
var_dump($array);
echo '</pre>';
When you run this small PHP script, you should end up with a result, as we have shown below.
array(4) {
[0]=>
string(5) "apple"
[1]=>
string(6) "banana"
[2]=>
int(1234)
[3]=>
int(6345)
}
You can see that the “var_dump()
” function outputs every array element.
The dump for an array starts with its variable type and the number of elements. The basic syntax is array(NUMBER OF ELEMENTS)
.
All of the elements within the array are then referenced within the curly brackets ({ }
).
For each element, the key ([0]
), variable type and its value are output by the var_dump()
function.
Using PHP’s var_dump Function on Multiple Variables
As mentioned earlier in this guide, PHP’s var_dump
function allows you to pass multiple variables in a single function call.
This is useful when you have several variables you want to dump the information for but don’t want to write several var_dump
function calls.
For this example, let us create three variables with different values.
- First, a variable called “
integer
” with the value of “200
“. - Secondly, a variable called “
website
” with the value “pimylifeup.com
“. - Finally, a variable called “
float
” with a floating number of “3.14
“.
With all the variables defined, we can pass each one into the “var_dump
” function, separating each one by a comma so they are regarded as a new parameter.
Additionally, as we are using multiple variables we should use the “pre
” tag so that new lines are preserved during output.
<?php
$integer = 200;
$website = "pimylifeup.com";
$float = 3.14;
echo '<pre>';
var_dump($integer, $website, $float);
echo '</pre>';
Below you can see how var_dump
easily handled each variable we passed in. Print out each of their data types and their corresponding values.
int(200)
string(14) "pimylifeup.com"
float(3.14)
Conclusion
Hopefully, you now have a solid understanding of using the var_dump
function in PHP.
It is an incredibly useful debugging tool as it allows you to print the value and type of any variable easily.
If you have any questions about using the var_dump()
function, feel free to comment below.
To learn more about the PHP language, check out our numerous PHP tutorials.