In this tutorial, we will be showing you how to get the current page URL within PHP.
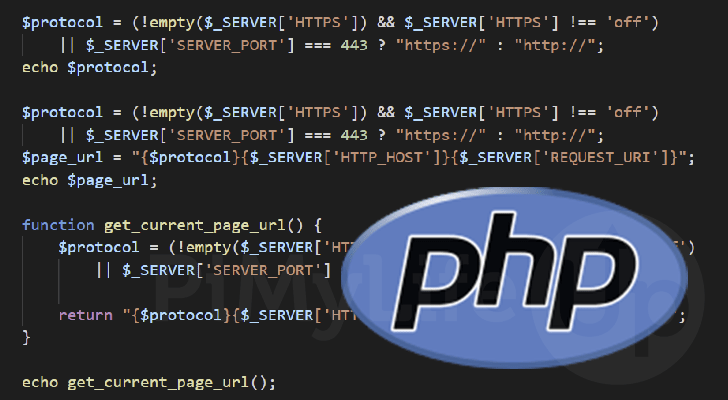
The PHP language features super global constants that can contain various information. You can identify one of these global constants by the usage of a single underline at the start of their name.
The super global that we will be using, in particular, is called “$_SERVER
“. This PHP constant contains information about the current request, including headers, paths, and script locations.
Using the information that this constant provides, we will be able to use PHP to get the current page URL.
Before we proceed any further, there are security implications from using most variables stored in this constant. For example, a client can set both “HTTP_HOST
” and “REQUEST_URL
” to any value.
Over the next section, we will show you how you can retrieve each part of the
Table of Contents
Getting the Current Page URL in PHP
There isn’t a singular function or variable that we can utilize to build the current page URL in PHP. Instead, we have to piece the URL back together using several values.
Over the next couple of sections, we will quickly go over each variable we will be using and showcase the sort of data it gives us.
If you prefer to skip the breakdown, go to the “Putting it All Together” section.
Retrieving the Hostname within PHP
The first piece of information we need to get the current page URL is the hostname. The hostname is like a label that identifies the device. In the case of a URL, this is often the website’s domain name.
For example, the hostname for our website is “pimylifeup.com
“.
The hostname is set within the “$_SERVER
” array under the key “HTTP_HOST
“. Our little code snippet below shows you how simple it is to grab this value.
<?php
echo $_SERVER['HTTP_HOST'];
?>
Running this little snippet, you will end up with just the hostname for your current page. We will use this to build the full page URL within PHP eventually.
For example, we got the following value from running this on our local development server.
development.local
Getting the Request URI in PHP
The second piece of information we need to get the current page URL in PHP is the request URI. The URI is the part of the URL after the hostname, including the query string.
For example with the URL “https://pimylifeup.com/category/coding/php/?p=2
” the request URI would be “/category/coding/php/?p=2
“.
Like the hostname, the request URI is also available within the “$_SERVER
” super global constant.
You can get this value by using the “REQUEST_URI
” key of the “$_SERVER
” array, as shown below.
<?php
echo $_SERVER['REQUEST_URI'];
?>
Testing this out again in our little “test.php
” script, we got the following.
/test.php?t=pimylifeup
Checking for HTTP or HTTPS within PHP
The final part we need to get the current page URL in PHP is the protocol that was utilized for this connections. This matters as it tells us whether the current URL contains “http
” or “https
“.
The protocol is probably the most difficult to retrieve, but it is still very straightforward.
To check whether the user used HTTPS for the current request, we can check the “$_SERVER
” super global and see if the “HTTPS
” variable was not set to “off
“.
The usage of PHP’s ternary operator (? :
) allows us to simplify this conditional statement into a single line. If “$_SERVER['HTTPS']
” is not equal to “off
” we set protocol to “https://
“, other we set it to “http://
“.
<?php
$protocol = $_SERVER['HTTPS'] !== 'off' ? "https://" : "http://";
echo $protocol;
?>
While it would be nice if that were all we needed to do, there is a chance the “HTTPS
” key is unavailable or empty.
We can also check whether the “SERVER_PORT
” key is set to 443
(Port used by HTTPS by default).
Let us re-adjust the code, now using PHP’s “empty()
” function on “$_SERVER['HTTPS']
” to see if it is not empty. If it is empty we utilize the or operator (||
) to check whether the “SERVER_PORT
” key is set to 443
.
<?php
$protocol = (!empty($_SERVER['HTTPS']) && $_SERVER['HTTPS'] !== 'off') || $_SERVER['SERVER_PORT'] === 443 ? "https://" : "http://";
echo $protocol;
?>
Running either one of these code examples should leave you with the protocol used for the incoming connection.
Our development server is set up to serve over HTTPS, so we got the following result.
https://
Putting it All together to Get the Current Page URL in PHP
Now that we know how to get the protocol, hostname, and request URI, we can use it to build a full-page URL.
First, we will work out the protocol using the “$_SERVER
” array using both the “HTTPS
” and “SERVER_PORT
” keys. We will store the protocol in a variable called “$protocol
“.
After we have worked out the correct protocol, we can use it to build the current page URL using PHP’s string expansion.
We create this PHP string using double quotes, referencing each of the following variables within the string in the following order “$protocol
“, “$_SERVER['HTTP_HOST']
” and “$_SERVER['REQUEST_URI']
“.
When PHP processes the string, it will expand each variable into its value.
Finally, we use PHP’s echo keyword to output the value we have stored in the “$page_url
” variable.
<?php
$protocol = (!empty($_SERVER['HTTPS']) && $_SERVER['HTTPS'] !== 'off') || $_SERVER['SERVER_PORT'] === 443 ? "https://" : "http://";
$page_url = "{$protocol}{$_SERVER['HTTP_HOST']}{$_SERVER['REQUEST_URI']}";
echo $page_url;
?>
After running this piece of PHP, you will get the current page URL output. For our example, we get the following URL.
https://development.local/test.php
Writing a Function to Get the Current Page URL in PHP
Let us wrap this up by writing a function to allow you to get the current page URL in PHP quickly.
With this we are creating a function called “get_current_page_url()
“. Within this function, we use the same code previously.
However, this time instead of setting the page URL to a variable, we will instead return it as a string.
<?php
function get_current_page_url() {
$protocol = (!empty($_SERVER['HTTPS']) && $_SERVER['HTTPS'] !== 'off') || $_SERVER['SERVER_PORT'] === 443 ? "https://" : "http://";
return "{$protocol}{$_SERVER['HTTP_HOST']}{$_SERVER['REQUEST_URI']}";
}
?>
Now whenever you need the current page URL you can utilize “get_current_page_url()
“.
Conclusion
In this guide, we have shown you how it is possible to get the current page URL within PHP.
Thanks to the “$_SERVER
” global constant, all of the information we need to build the page URL is available. When using this in a production environment, you should consider sanitizing the values to ensure they are safe.
If you have any questions about how to get the current page URL in PHP, please comment below.
Please check out our many other PHP tutorials, or if you want to learn a new language, check out our coding guides.