In this tutorial, we will be showing you how to use strings within the PHP language.
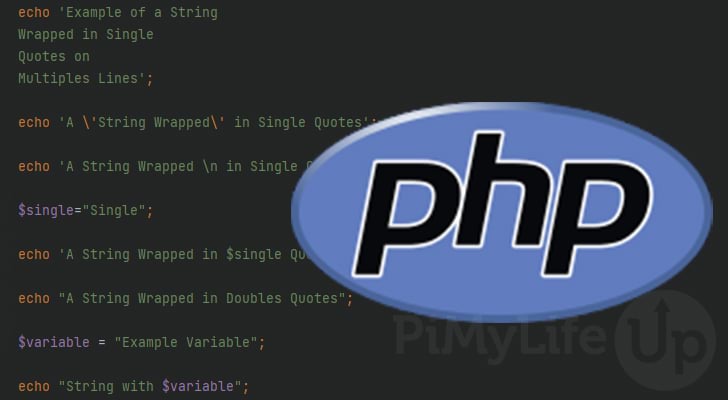
In PHP, a string is a data type that allows you to store a series of characters. The PHP language only supports 256 characters as each letter is stored as a byte. A byte only has a max of 256 different values.
There are various ways that you can define a string within PHP. Each of these methods behaves differently and have their pros and cons.
These different methods of specifying a string are the following.
- Single Quotes (
' '
) - Double Quotes (
" "
) - Heredoc Syntax (
<<<IDENTIFIER
) - Nowdoc Syntax (
<<<'IDENTIFIER'
)
Over this guide, we will be going over each of the different ways to define a string within the PHP language.
If you are just getting started with PHP, we recommend focusing on single and double-quotes. They are the most straightforward ways of defining a string and are what you will likely use the most.
Single Quoted Strings in PHP
You can define a string within the PHP language by wrapping it in single quotes (' '
).
echo 'a String wrapped in Single Quotes';
Copy
When you use single quotes, PHP will not expand special characters within the string. It only supports escaping the single quote/apostrophe ('
) or the backslash character (\
).
Single quoted PHP strings are slightly faster than double-quoted strings and should be used in cases where you don’t want the string evaluated.
If you want to use special characters or variables within the string you will need to use double-quoted strings.
Example of Using Single Quotes to Define a string in PHP
Over the following few sections, we will explore some of the various behaviors of single quotes within PHP.
We will explore how single quotes are used and how to deal with its various edge cases
Basic Usage of Single Quotes
The simplest way to use single quotes in PHP is to contain a string within them (' '
).
For example if we wanted to print the text “Example of a String Wrapped in Single Quotes
” in PHP we could use the following code.
<?php
echo 'Example of a String Wrapped in Single Quotes';
?>
Copy
Multiple Lines using Single Quotes
As single quotes in PHP do not support expanding escaped characters like “\n
” you will have to embed the new lines in the string.
This means that you need to define the string over multiples lines, as shown below.
<?php
echo 'Example of a String
Wrapped in Single
Quotes on
Multiples Lines';
?>
Copy
Using A single Quote within Single Quotes in a String
If you are writing a string where you want to include a single quote or apostrophe, you will need to escape it.
You can escape a single quote ('
) by using a backslash (\
) immediately before it like so “\'
“.
For example, if we wanted to show a quoted string within the string, we can write it like the following.
<?php
echo 'A \'String Wrapped\' in Single Quotes';
?>
Copy
Single Quotes Don’t Expand
PHP will not expand escaped characters or variables within your string when you use single quotes.
For example, using the line feed sequence “\n
” within a single-quoted string, will simply be printed as “\n
“.
<?php
echo 'A String Wrapped \n in Single Quotes';
?>
Copy
Likewise, if you tried to include a PHP variable within the single-quoted string, it would be just interpreted as characters.
<?php
$single="Single";
echo 'A String Wrapped in $single Quotes';
?>
Copy
Double Quote Strings in PHP
The second way to define strings within PHP is to use double (" "
) around a sequence of characters. Double quotes are helpful when you want the contents of the string to be evaluated.
echo "A String Wrapped in Doubles Quotes";
Copy
The advantage of doubled quoted strings is that PHP will expand values within the string. So, for example, PHP will expand an escaped character like “\n
” into a linefeed.
The most crucial feature of double-quoted strings is that PHP will expand variable names back out into their value.
Supported Escaped Characters
When a string is encased in double-quotes in PHP, it has support for several escape sequences. You escape a character by including a backslash (/
) before the character.
You can see some of the various escape sequences supported by PHP by referencing our table below.
Escape Sequences | Replacement |
---|---|
\n | Newline (Linefeed) Character |
\r | Carriage Return Character |
\t | Horizontal Tab Character |
\v | Vertical Tab Character |
\e | Escape Character |
\f | Form feed character (Page break) |
\\ | Backslash Character |
\$ | Dollar Sign Character |
\' | Single Quote |
\" | Double Quote |
\x[0-9A-Fa-f]{1,2} | Hexadecimal Character |
\u{[0-9A-Fa-f]+} | Unicode Character |
Example of Using Double Quoted Strings
Over the next section, we will show you various ways to utilize double quote (" "
) strings in PHP.
These examples will show you how PHP handles double quote strings.
Basic Usage of a Double Quoted String in PHP
To use double quotes in PHP, you need to place a double quote (" "
) before starting your string and one after.
For example, we would use the following to print the text “This is a double-quoted string
“.
<?php
echo "This is a double-quoted string";
?>
Copy
Multi-Line Strings using Double Quotes
With double quotes in PHP, you can create multi-line strings in a couple of ways.
The first of these ways is to embed the new lines directly into your string. PHP will automatically handle these
<?php
echo "Example of a
Multi-Line String using
Embedded New Lines";
?>
Copy
Alternatively, you can insert newlines into your string with the new line character “\n
“.
When PHP passes the double-quoted string, it will expand the escaped character sequence into a new line.
<?php
echo "Example of a\nMulti-line String\nUsing New Line Character";
?>
Copy
Escaping Double Quotes in a Double Quote String in PHP
If you use a doubled quoted string in PHP and want to use double quotes, you will need to escape them.
To escape a double quote, we need to place a backslash (\
) before the double quote ("
).
For example, we would use the following code if we wanted to use the string “Double quotes string "with double" quotes
“.
<?php
echo "Double quoted string \"with double\" quotes";
?>
Copy
Strings in PHP Using Double Quotes Expands
The biggest advantage of using double quotes to define a string is PHP will expand variables and escaped characters.
When PHP finds a variable name, it will perform a lookup and insert that value into the string in its place.
<?php
$variable = "Example Variable";
echo "String with $variable";
?>
Copy
Using the Dollar Sign in Double Quoted Strings
As a dollar sign symbol signifies the start of a variable within a double string, you may need to escape it.
To escape the dollar sign ($
), you simply place a backslash before it “\”.
With our example below, you can see that PHP won’t insert the value of our variable into the string thanks to the backslash.
<?php
$variable = "Example Variable";
echo "String with no \$variable";
?>
Copy
Using the Heredoc Syntax in PHP
The third way of defining a string within PHP is called the heredoc syntax. This is an ever so slightly more complicated syntax with its advantages, especially for code readability.
- First, you don’t need to worry about escaping single or double quotes within a heredoc string, thanks to the way it is defined.
- Secondly, like double-quoted strings, variables and other character sequences are expanded.
To define a string in PHP using heredoc, you need to use three less than signs “<<<
” followed immediately by the identifier. In addition, the identifier must follow the standard PHP naming rules.
The string must end with the identifier you specified on a new line without whitespace.
Below, you can see an example of a correctly written heredoc string when written in PHP.
echo <<<IDENTIFIER
This is an example
of a valid heredoc
string in PHP
IDENTIFIER;
Copy
Examples of Using heredoc in PHP
As PHP’s Heredoc string syntax can be a little hard to understand at first, let us explore several different ways to utilize it.
You will quickly see that the Heredoc syntax makes the code much more readable when dealing with multiple lines of text.
Simple Usage of heredoc in PHP
Below is an example of writing a single-line string using the heredoc syntax within PHP. For this example, we will store the string “Hello World.
” within the variable called “$string
“.
While this isn’t the best usage of the heredoc syntax, it gives you an idea of how to utilize it.
<?php
$string = <<<IDENTIFIER
Hello world.
IDENTIFIER;
echo $string;
?>
Copy
Writing a Multiline String Using the heredoc Syntax
Writing a multiline string is very simple with the heredoc syntax in PHP. Simply embed new lines into the string.
Below is an example of how you can write a multi-line string within PHP.
<?php
$string = <<<IDENTIFIER
Hello world.
This is an example of heredoc.
Fairly simple.
IDENTIFIER;
echo $string;
?>
Copy
Using Single and Double Quotes within PHPs heredoc Syntax
One of the most significant advantages of using the heredoc syntax in PHP is that you do not have to worry about escaping single ('
) or double quotes ("
).
Not escaping these symbols makes your string a lot more readable without changing the actual functionality.
Below you can see how we have written a string using the heredoc syntax, including single or double quotes.
<?php
$string = <<<IDENTIFIER
Hello World
"Double Quotes"
Or 'Single Quotes'
IDENTIFIER;
echo $string;
?>
Copy
The heredoc Syntax Expands Variables and Escape Character Sequences
Another advantage of the heredoc string syntax is that it will still expand variables and escape character sequences.
For example, if you wanted to include the value of your variable called “$example
” within the string, all you need to do is include it in the string, as shown below.
Additionally, escape sequences like “\n
” or “\t
” will be expanded as well.
<?php
$example="Hello World";
$string = <<<IDENTIFIER
Hello World
Variable Value is: $example
\n\nHeredoc Expands Variables\n\n
\tAs well as Escaped Characters
INDENTIFIER;
Copy
The Nowdoc Syntax in the PHP Language
The Nowdoc string syntax in PHP is very similar to Heredoc. In a way, it can be considered very similar to using single quotes.
With a Nowdoc string, no variable or escaped character sequence is evaluated. This functionality is useful when you are writing a plain string and do not need to include
To write a Nowdoc string, you must first start it with three less than signs (<<<
), followed by an identifier wrapped in single quotes ('IDENTIFIER'
) and then a new line.
Additionally, this string must also end with the identifier at the start of a new line. The final identifier does not have to be enclosed in single quotes.
<<<'IDENTIFIER'
This is an example
of a valid heredoc
string in PHP
IDENTIFIER;
Copy
Example of Using Nowdoc Strings in PHP
Since the Nowdoc string syntax in PHP is similar to Heredoc, we will only be going over some quick examples.
These examples will focus on how PHP will not expand values held within a Nowdoc string.
Basic Usage of the Nowdoc Format
Writing a simple single-line string isn’t the best usage of the Nowdoc string format in PHP. However, it will give you a good understanding of the basic syntax.
We will store a string with the text “Hello World
” within the variable. For our Nowdoc identifier, we will simply use “'IDENTIFIER'
” for this example.
<?php
$string = <<<'IDENTIFIER'
Hello World
IDENTIFIER;
echo $string;
?>
Copy
A Multi-Line String using Nowdoc in PHP
The Nowdoc format works best with readability when dealing with multiple lines of text.
As a Nowdoc string will not expand elements, you must embed newlines within the string itself.
Below is an example of how you would write a multi-line Nowdoc string in PHP. You can see from this example that PHP will not expand the new line character.
<?php
$string = <<<'IDENTIFIER'
Hello World
Embed new Lines into your string
As Nowdoc will not expand \n
IDENTIFIER;
echo $string;
?>
Copy
Nowdoc Strings Don’t Expand Values
This example will show you the main difference between a Nowdoc and a Heredoc string in PHP.
With the Nowdoc syntax, variables and escaped characters will not be expanded when PHP parses the string. This is useful when you want to use characters without worrying about them being expanded.
For this example, we will show you how to use a variable called “$variable
” and two escaped characters (\t
and \n
) without PHP expanding them.
<?php
$variable = "I won't be expanded";
$string = <<<'IDENTIFIER'
Hello World
PHP wont expand a $variable
\tOr Escaped Characters\n
When Using Nowdoc.
IDENTIFIER;
echo $string;
?>
Copy
Conclusion
Hopefully, by this point in the tutorial, you will now understand how to define strings in PHP.
Each of the various string definitions has its usage. Choose which way feels most readable to you and achieves the task easily.
For example, if you wanted to write a single line string that will expand variables, you will likely use double-quoted strings.
If you have any questions about strings in PHP, please feel free to comment below.
Additionally, to learn more about the programming language, check out our various PHP guides.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support