In this tutorial, we will explain the basics of how variables are handled within the PHP programming language.
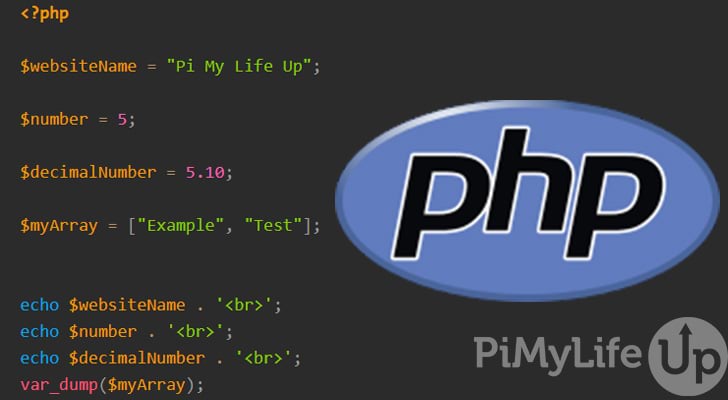
If you look at any PHP script, you are likely to find variables, so it is very important that you have a good understanding of the basics. A variable is used to store data, and in PHP, they are represented by the $
sign followed by the variable name.
Throughout this tutorial, we will take you through a range of important topics regarding variables in PHP. For example, we will touch on naming conventions, data types, variable scopes, and creating a variable variable. We keep things relatively basic, so it is great if you are just starting out with programming.
This tutorial is for PHP 8 or 8.1. If you are using a newer or older version of PHP, then this tutorial may not be entirely accurate. However, it is unlikely most of the fundamentals have changed.
Table of Contents
- Variable Names in PHP
- Predefined Variables
- Using Variables in PHP
- Variable Data Types
- Variable Scopes
- Variable Variables
- Conclusion
Variable Names in PHP
Like most programming languages, PHP has a set of rules for naming a variable. In this section, we will touch on valid variable names.
All variables in PHP start with the dollar sign ($) followed by the variable’s name. A missing $
will trigger an error when you try to run the code.
To create a valid variable name in PHP, you must start with a letter or underscore. If the variable starts with a number or any other character, it will be invalid.
After the starting character, a variable name can contain letters, numbers, or underscores.
Variable names in PHP are case-sensitive, so make sure you follow the same capitalization every time you reference a variable.
Below are a few examples of valid and invalid variable names in PHP.
//Valid PHP Variable Names
$pimylifeup;
$_pimylifeup;
$pimylifeup1;
$pi_my_life_up;
//Invalid PHP Variable Names
$0pimylifeup;
$*pimylifeup;
$pi my life up;
Predefined Variables
PHP contains a range of predefined variables you will not be able to use as names. If you try to use any of these as a variable name, you might experience unexpected behavior.
Below are some of the predefined variables that you can use within PHP.
- $GLOBALS
- $_SERVER
- $_GET
- $_POST
- $_FILES
- $_REQUEST
- $_SESSION
- $_ENV
- $_COOKIE
- $php_errormsg
- $http_response_header
- $argc
- $argv
The available predefined variables in PHP may vary depending on the version that you are using.
Using Variables in PHP
Using variables in PHP is super easy, thanks to it being a loosely typed language. Being loosely typed means you do not need to define the variable that you are declaring. So, for example, if you assign a “string” to a variable, the variable type will automatically be a string.
Being loosely typed can help make your code more flexible and reduce errors. However, on the flip side, it may sometimes produce unexpected results in cases where you expected a certain data type but got something else.
The following two sections will discuss declaring and outputting the variables in a PHP script.
Declaring Variables
Declaring a variable in PHP is super easy, you specify your variable name and assign the value you want using the =
operator.
In the example below, we assign four variables with a unique data type. Also, whenever you specify text make sure you enclose it within quotes.
<?php
$websiteName = "Pi My Life Up";
$number = 5;
$decimalNumber = 5.10;
$myArray = ["Example", "Array"];
Outputting Variables
Outputting a variable in PHP is very straightforward. Typically you will use print or echo to output the variable, but in some cases, you might need to use var_dump
or a different function.
In the example below, we output a range of different variables using echo or var_dump
(Outputting contents of an array).
<?php
$websiteName = "Pi My Life Up";
$number = 5;
$decimalNumber = 5.10;
$myArray = ["Example", "Test"];
echo $websiteName . '<br>';
echo $number . '<br>';
echo $decimalNumber . '<br>';
var_dump($myArray);
Below is the output of running the above script. Each of our variables printed to our screen successfully. Also, by using var_dump
on our array, we could see each value stored in the array, including their data type.
Pi My Life Up
5
5.1
array(2) { [0]=> string(7) "Example" [1]=> string(4) "Test" }
Variable Data Types
There is a range of different data types that you can assign to a variable. We will briefly touch on some data types you can use.
PHP does not enforce type definition when you create a variable. Instead, PHP will determine the type by the value stored in the variable. For example, if a string is assigned to $var
, the variable will be of type string. It has pros and cons, but we will cover that in another guide.
String – A string consists of characters and is defined inside either single or double-quotes.
$x = "Pi My Life Up";
Integers – Is a non-decimal number that can be anywhere between -2,147,483,648 and 2,147,483,647.
$x = 1021;
Float or Double – is a number that contains a decimal point.
$x = 10.123;
NULL – A special data type reserved for variables with no value assigned. A variable created with no value will automatically be assigned null. You can also assign null to a variable.
$x;
$y = NULL;
Boolean – This data type supports only two different states, TRUE or FALSE.
$x = TRUE;
Arrays – This data type allows you to store multiple values in a variable.
$fruit = ["Apple","Banana","Orange"];
Objects – You can store an object as a variable. An object is a significant aspect of object-oriented programming.
$apple = new Apple("Red", "Small", "Fresh");
Resources – This is not a data type but instead stores a reference to function and resources external to the PHP script. For example, a reference to a database call.
$file = fopen("test.txt","w");
Variable Scopes
Since you can declare PHP variables anywhere within a PHP script, you will need to be aware of variable scope.
There are three variable scopes within PHP: local, global, and static. We will briefly cover each of these scopes below.
Global Scope
A variable with global scope is defined outside a function and can only be accessed outside a function. Therefore, if you try using a global variable inside a function, it will be empty.
In the example below, we create a global variable $x
and use it inside and outside a function.
<?php
$x = "Apple";
function variableTest(){
echo "I am inside the function and x is " . $x;
}
variableTest();
echo "I am outside the function and x is " . $x;
As you can see in our output below, when we tried using our $x
global variable inside the function, the variable is empty since it is global.
I am inside the function and x is
I am outside the function and x is Apple
Using the global keyword
You can access a global variable inside a function by using the global keyword. Alternatively, you can use the $GLOBALS
array to access a variable.
We use the global keyword to access our $x
variable in the example below. You can also do this by using the globals array, for example $x = $GLOBALS['x']
.
<?php
$x = "Apple";
function variableTest(){
global $x;
echo "I am inside the function and x is " . $x . "<br>";
}
variableTest();
echo "I am outside the function and x is " . $x;
As you can see in our output below, we now have access to our global variable $x
inside the function.
I am inside the function and x is Apple
I am outside the function and x is Apple
Local Scope
A variable declared inside a function has local scope and cannot be accessed outside of the function.
You can reuse local scope variable names in different functions since a variable with local scope is constrained to the function it is declared in.
In the example below, we demonstrate that $y
is available inside the function but not outside of it.
<?php
function variableTest(){
$y = "Apple";
echo "I am inside the function and y is " . $y . "<br>";
}
variableTest();
echo "I am outside the function and y is " . $y;
As expected, the local variable is not available outside the function.
I am inside the function and y is Apple
I am outside the function and y is
Static Scope
Lastly, you might want to make a variable static by using the static keyword. A static variable will survive and retain its value after a function has completed. If we use the function again, the static value will still contain the value it was last assigned.
In the example below, we create a static variable $y
inside the function variableTest()
that we call four times. Each time the function is called, our static variable will increase by 2
and never reset to 0
.
<?php
function variableTest(){
static $y = 0;
echo $y . "<br>";
$y = $y + 2;
}
for($i = 0; $i < 4; $i++){
variableTest();
}
The output below shows our static variable $y
increase by 2
on every call to the function.
0
2
4
6
Variable Variables
In PHP, it is possible to have “variable” variable names. A variable variable takes the normal value of a variable and uses that as its name. You can assign values to the variable and access it by its name.
To create a variable variable, first declare a regular variable ($x
) and assign a string to it, in our case, “food“. Next, add an extra dollar sign ($$x
), and assign another value, in our case, it’s “Potato“. You can now reference our variable using $food
.
<?php
$x = "food";
$$x = "Potato";
echo $food;
$food = "Carrots";
echo $food;
In the above example, we create a variable variable with the name “food” by adding an extra dollar sign and assigning it another value “Potato“.
We also use echo to output our variable’s value before and after we update the value.
Potato
Carrots
This overview is very basic, and there is quite a bit you can do using variable variables. We will touch on this useful technique in a future tutorial.
Conclusion
Hopefully, by now, you have a decent understanding of how to use variables within the PHP programming language. We have covered most of the basics for handling variables, including scopes, data types, declarations, and more.
There is always much more to learn about PHP and other programming languages, so I recommend checking out some of our other PHP tutorials. If you are new to coding, you might find our tutorial on if else statements incredibly useful.
If we can improve this tutorial, please do not hesitate to let us know by leaving a comment below.