We will go into the various operators that you can use within the PHP language within this guide.
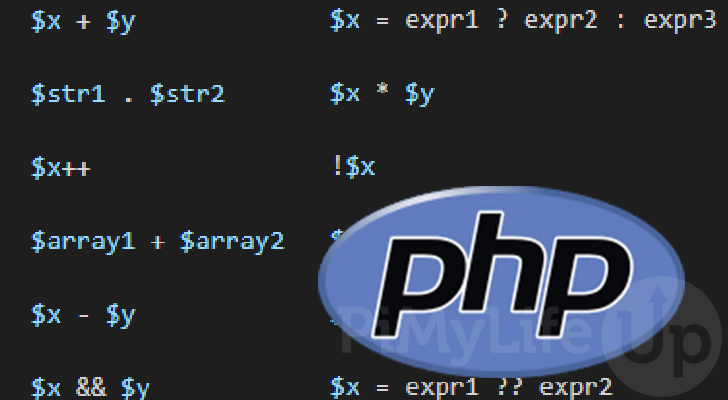
An operator in PHP is used to perform operations on variables. For example, PHP can use operators to take one or more values and create a new resulting value.
Operators are a crucial part of PHP and one of the topics that you should familiarize yourself with. These help you manipulate values and are essential to writing PHP scripts.
Within our guide, we will be grouping the various operators by what they are used for. For example, the addition operator (+
) is categorized as an arithmetic operator.
Over the following few sections, we will show you all of the operators you can use in PHP.
Table of Contents
- Categorizing PHP Operators
- Arithmetic Operators
- Assignment Operators
- Comparison Operators
- Increment/Decrement Operators
- Logical Operators
- String Operators
- Array Operators
- Conditional Assignment Operators
- Conclusion
Categorizing PHP Operators
There are a few ways to group the operators that PHP supports. Two of these ways are to either group by their usage or group by the number of values they can operate on.
The way that we will be using within our guide is based on the usage of the operators. Below is a list of all of the usage categories.
- Arithmetic Operators
- Assignment Operators
- Comparison Operators
- Increment / Decrement Operators
- Logical Operators
- String Operators
- Array Operators
- Conditional Assignment Operators
You can also group operators into three groups, each representing the number of values they can operate on.
- The first are unary operators. These only operate on a single value.
An example of a unary operator is the logical not operator (!
) or the increment operator (++
). - Secondly are binary operators. These are the type of operators you will use most when using the PHP language. These operators take two values to create a new value.
You might already be familiar with two of these operators, the arithmetic plus (+
) and minus operators (-
). - Finally, there are ternary operators. These are operators which take three values.
The only operator in PHP that takes three values is the operator that shares the same name as the group, the ternary operator (?:
).
Arithmetic Operators in the PHP Language
Arithmetic operators in PHP allow you to perform mathematic operations such as addition, subtraction, multiplication, and more.
For example using the plus (+
) symbol you can add the numeric value of variables together.
Below you can see a list of all of the arithmetic operators that are supported by default in PHP.
Operator | Name | Example | Result |
---|---|---|---|
+ | Addition | $x + $y | Sum of $x and $y |
- | Subtraction | $x - $y | Difference between $x and $y |
* | Multiplication | $x * $y | Product of $x and $y |
/ | Division | $x / $y | Quotient of $x and $y |
% | Modulus | $x % $y | Remainder of the division of $x by $y |
** | Exponentiation | $x ** $y | Raises $x to the $y th Power |
Addition (+
) Operator
The first arithmetic PHP operator that we will explore is the addition operator (+
). Using this, we can add the numeric value of two variables together.
Using the addition operator, the value on the left side will have the value of the right side added to it. This operation will return the sum of these two values.
$x + $y
Subtraction (-
) Operator
The subtraction operator (-
) allows you to subtract the numeric value of one variable from another.
With this PHP operator, the value on the left side will have the value on the right side subtracted from it.
$x - $y
Multiplication (*
) Operator
The third PHP arithmetic operator that we will be touching on is the multiplication (*
) operator. The asterisk symbol within PHP represents this operator.
With this operator, the value on the left side will be multiplied by the variable on the right side.
$x * $y
Division (/
) Operator
The division operator (/
) allows you to divide one numeric value by another numeric value easily. To use this operator within PHP, you need to use the forward-slash symbol (/
).
Using this operator, the value on the left side will be divided by the value on the right.
$x / $y
Modulus (%
) Operator
The fifth PHP arithmetic operator that we will be touching on is the modulus operator (%
). This operator allows you to get the remainder after division.
This operator will divide the value on the left side of the operator by the value on the right side. It will return the remainder from this operation.
$x % $y
Exponentiation (**
) Operator
The final PHP arithmetic operator that we will explore is the exponentiation (**
) operator.
This operator allows you to multiply the value on the left side by the power of the value on the right side.
$x ** $y
PHP Assignment Operators
In this section, we will be showing you how to use the assignment operators within PHP.
Assignment operators are used to set the value of a variable to a different value. They even offer shorthand ways to perform math operations on variables.
Below is a table outlining the various assignment operators supported within the PHP language. In addition, we have included an example of how these operators are used, including their long form equivalents.
Operator | Name | Example | Long Equivalent | Description |
---|---|---|---|---|
= | Assignment | $x = $y | $x = $y | $x is set to the value of $y |
+= | Addition | $x += $y | $x = $x + $y | $y will be added to $x |
-= | Subtraction | $x -= $y | $x = $x - $y | $x is subtracted by $y |
*= | Multiplication | $x *= $y | $x = $x * $y | $x will be multiplied by $y |
/= | Division | $x /= $y | $x = $x / $y | $x will be divided by $y |
%= | Modulus | $x %= $y | $x = $x % $y | $x is set to the remainder of $x divided by $y |
**= | Exponentiation | $x **= $y | $x = $x ** $y | $x is raised to the power of $y th |
Assignment (=
) Operator
The most straightforward operator in the PHP language is the assignment (=
) operator. This operators allows you to set the value of a variable to a new value.
The variable referenced on the left side of the operator will be set to the value on the right side.
$x = $y
Addition Assignment (+=
) Operator
In PHP, you can use arithmetic to modify values during the assignment process. The first of these that we will be touching on is the addition assignment (+=
) operator. This operator is represented by the plus and equal sign symbols.
The variable on the left side will have the value of the expression on the right side added to it. PHP will assign the resulting value to the variable on the left.
$x += $y
If you were to write this in long-form, you would have to write the following.
$x = $x + $y
Subtraction Assignment (-=
) Operator
PHP’s subtraction assignment (-=
) operator allows you to subtract a value from a variable and assign it immediately. This operator saves you from writing out a longer line of code to subtract a value from a defined variable.
The variable on the left side will have the value on the right side subtracted from it. The variable will then be assigned the resulting value of the operation.
$x -= $y
The long-form equivalent of this operation would be to use the following.
$x = $x - $y
Multiplication Assignment (*=
) Operator
The multiplication assignment (*=
) operator allows you to quickly multiply the value of a variable by another, assigning the new value in the process.
Using this operator in PHP, the variable on the left will be multiplied by the value on the right side. PHP will assign the resulting value to the variable on the left.
$x *= $y
The longer form of writing this operator would be to use the following.
$x = $x * $y
Division Assignment (/=
) Operator
The division assignment (/=
) operator allows you to divide a variable’s value by another and immediately assign that result value.
It saves you from repeatedly referencing the same variable within the same line.
The variable on the left side will be divided by the value of the right-side expression. Then, the result of this arithmetic is assigned to the left side variable.
$x /= $y
A long-form of writing this is to reference the variable’s name again and use the division operator.
$x = $x / $y
Modulus Assignment (%=
) Operator
The modulus assignment (%=
) operator allows you to get the remainder from a division operation and assign it to variable.
With this operator, the variable on the left side will be divided by the value on the right side. Then, the remainder from this operation will be assigned to the left side variable.
$x %= $y
If you were to write this out in a long format, you could write the same thing as shown below.
$x = $x % $y
Exponentiation Assignment (**=
) Operator
The last assignment operator that we will be looking into is the exponentiation assignment (**=
) operator,
This operator allows you to raise the variable on the left side to the power of the variable on the right side. PHP will save the result to the left-side variable.
$x **= $y
The long-form version of this assignment operator is shown below. This form explicitly uses the exponentiation operator to raise “$x
” by “$y
“.
$x = $x ** $y
Comparison Operators in PHP
Comparison operators are a critical part of the PHP language and are used to compare two values. There are many of these operators, and each has its use case.
The result for all but one of these operators will always be True or false. These are the operators you will use alongside conditional statements in PHP, such as the if statement.
One of the essentialthings you should learn from comparison operators in PHP is the difference between “==
” and “===
“. If you are familiar with JavaScript, you may already know the difference.
Below you can check out the table outlining the various comparison operators supported within the PHP language. If you would like to learn more about any of these operators, read on.
Operator | Name | Examples | Result |
---|---|---|---|
== | Equals | $x == $y | True if $x is equal to $y , types can be different |
=== | Identical | $x === $y | True if $x is equal to $y and they are of the same type. |
!= | Not Equal | $x != $y | True if $x is not equal to $y , types can be different |
<> | Not Equal | $x <> $y | True if $x is not equal to $y , types can be different |
!== | Not Identical | $x !== $y | True if $x is not equal to $y or if the types are different |
< | Less Than | $x < $y | True if $x is less than $y |
> | Greater Than | $x > $y | True if $x is greater than $y |
<= | Less than or Equal To | $x <= $y | True if $x is Less than or Equal to $y |
>= | Greater Than or Equal To | $x >= $y | True if $x is Greater than or Equal to $y |
<=> | Spaceship | $x <=> $y | Compares $x to $y .Returns an int with one of the three values: -1 – If $x is less than $y 0 – If $x is equal to $y .1 – If $x is greater than $y . |
Equals (==
) Comparison Operator
The first of the PHP comparison operators that we will be diving into is the equals (==
) comparison.
This operator simply checks whether the value on the left side is equal to the value on the right side. If they are the same, it will return true
, if not then false
.
$x == $y
The equals comparison operator will even perform type juggling if the variables are not of the same type.
Type juggling means that a string like “1
” will be considered the same as the number 1
. It does this by attempting to convert the string to a number.
If you want to force PHP not to use this behavior, you need to use the identical comparison operator instead.
Identical (===
) Comparison Operator
The identical (===
) operator in PHP allows you to perform strict comparisons between two variables.
Unlike the equals operator, this comparison requires both the value and the type to match. If they match, the operator will return true
. If either the value or type doesn’t match, PHP will return false
.
$x === $y
There are many reasons why you will want to use the identical comparison (==
) operator in PHP.
One of the significant reasons is to prevent type coercion. For example, there are many cases where you won’t want a string to match against a number and vice versa.
Not Equal (!=
or <>
) Comparison Operator
In PHP, there are two not equal comparison operators that you can use within your code. However, both of these operators behave the same, so you can use them interchangeably. These, not equal operators are “!=
” and “<>
“.
Using this comparison operator, you can check if two variables don’t contain the same value. It will return true
when the values aren’t the same and false
when they are the same. It is the opposite of the equals operator.
The main way to utilize the not equal operator (!=
) in PHP is as shown below.
$x != $y
However, you can also write the not equal operator (<>
) as shown below. This variation of the operator is rarely used within PHP.
$x <> $y
Like the equals operator, PHP will perform type juggling to see if two values match. For example, if you compare an integer and a string, PHP will convert the string to an integer.
So the integer 1
and the string "1"
will return false
when using the not equals (!=
) operator.
Not Identical (!==
) Comparison Operator
The not identical operator is similar to the not equals operator. The difference is that the not identical operator will also return false if the variable types do not match.
This operator works by comparing the value on the left to the value on the right. It checks to see if the values are not the same or are not the same type.
If the variables are not the same value and type, it will return true
otherwise, it will return false
.
$x !== $y
Less Than (<
) Comparison Operator
Within PHP, you can check whether a numerical value is less than another value. You need to use the less than (<
) comparison operator to do this.
This operator works by checking if the value on the left is less than the value on the right.
$x < $y
If the value on the left is less than the value on the right, the operator will return true
.
If the left value is greater than the right value, the operator will return false
.
If both values are the same value, this operator will return false
.
Greater Than (>
) Comparison Operator
Within this section we will be looking at the greater than ( >
) comparison operator in PHP.
Using this operator, you can see if the value on the left is greater than the value on the right.
$x > $y
If the left value is greater than the right value, the operator will return true
.
If the left value is less than the right value, the “greater than” operator will return false
.
If both values are equal, the operator will still return false
.
Less Than or Equal To (<=
) Comparison Operator
The PHP language allows you to combine both the less than and equal operators. This allows you to check if a value is less than or equal to another value.
Usually, the less than the operator would only return true if the values are less than it. If they match, it will return false.
This operator lets you check if the left value is less than or equal to the right value.
$x <= $y
If the value on the left is less than the right side value, the operator will return true
.
If the left-side value is greater than the right-side value, then the “less than or equal to” operator will return false
.
If the values on both sides are equal, the operator will return true
.
Greater Than or Equal To (>=
) Comparison Operator
The “greater than or equal to” comparison operator builds upon the normal “greater than
” operator. This operator can check to see if a value is greater than or equal to a value.
This operator is useful when you want to perform an action even if the values match.
Using this operator, you can check if the value on the left is greater than or equal to the value on the right.
$x >= $y
If the value on the left is greater than the value on the right, this operator will return true
.
If the left value is less than the right side value, the operator will return false
.
If both values are equal, then the operator will return true
.
Spaceship (<=>
) Comparison Operator
The last of the comparison operators in PHP is the spaceship operator (<=>
), also known as the combined comparison operator. This is one of the newest operators and was introduced with PHP 7.
What makes this operator unique is that it performs three different comparison checks. Those checks are equals to (=
), less than (<
), or greater than (>
).
The spaceship operator will check if the value on the left is less than, equal to, or greater than the value on the right.
$x <=> $y
Instead of returning true
or false
, the spaceship operator returns an integer with three possible values. The value of the integer tells you what comparison matched your variables.
-1
– If the value on the left is less than the value on the right0
– If both values are equal.1
– If the left value is greater than the right value.
Increment/Decrement Operators in the PHP Language
Increment and decrement operators in PHP allow you to easily increase or decrease the value of a variable by one.
PHP supports both pre and post incrementing a value. While both increase or decrease the value of a variable, the way they return that value is different.
Our table below shows you the various increment/decrement operators that are supported within the PHP language.
Operator | Name | Result |
---|---|---|
++$x | Pre-Increment | Increments $x by one, then returns the variables value |
$x++ | Post-Increment | Returns $x , then increments the variables value by one |
--$x | Pre-Decrement | Decrements $x by one, then returns the variable’s value |
$x-- | Pre-Increment | Returns $x , then decrements the variable’s value by one |
Pre operators will increase/decrease the value of a variable then return its value.
Post operators will return the variable’s value then increase/decrease the value.
Pre-Increment (++$x
) Operator
The pre-increment operator works in PHP by increasing the value stored within the variable. Once the value has been updated, it will return the value.
You need to use two plus symbols (++
), followed by your variable or expression.
++$x
Post-Increment ($x++
) Operator
The PHP language also supports post-incrementing a value. What this means is that the value will first be returned, then the value itself will increased.
This means the returned value is the initial value of the variable, not the incremented value.
Using this operator in PHP is as simple as using two plus symbols (++
) after the variable or expression.
$x++
Pre-Decrement (--$x
) Operator
The pre-decrement operator in PHP allows you to decrement the value of a variable by one. As a “pre” operator, the value will be decremented, then returned with the new value.
To use this operator, you only need to start it with two minus symbols (--
), as shown in the example below.
--$x
Post-Decrement ($x--
) Operator
In PHP, you can use the post-decrement operator to get a variables value and then decrement it.
Using post-decrement, PHP will return the value of your chosen variable or expression first. Then, after the value is returned, PHP will then decrease the value by one.
You can use the post-decrement operator by using two minus symbols (--
) in front of your variable or expression.
$x--
PHP Logical Operators
In this section we will be exploring the various local operators that you can use within the PHP language.
Typically you would see these operators used within conditional statements as they allow you to compare Boolean values. However, these operators do have additional functionalities.
PHP will attempt to convert values to either “true
” or “false
” when using logical operators. For example, PHP will evaluate non-zero numbers as “true
“, but zero will be considered “false
“.
Below is a table of all of the logical operators that are supported within the PHP language.
Operator | Name | Example | Result |
---|---|---|---|
and | And | $x and $y | True if both $x and $y are true |
&& | And | $x && $y | True if both $x and $y are true |
or | Or | $x or $y | True if either $x or $y is true |
|| | Or | $x or $y | True if either $x or $y is true |
xor | Xor | $x xor $y | True if either $x or $y is true, not both |
! | Not | !$x | True if $x is not true |
And (and
or &&
) Logical Operator
The PHP “and
” operator allows you to compare two values to see whether they are both true
. You can write this operator either as “&&
” or “and
“.
The difference between these two operators that perform the same action is the order in which PHP will perform that operation.
With the PHP and operator, if either value is not true
, it will return false
.
If both values are considered true
, the operator will return true
.
$x && $y
You can also write the “and” logical operator without the symbols by using the “and
” keyword in its place.
$x and $y
Or (or
or ||
) Logical Operator
In PHP, the or
(||
) operator allows you to compare two values, checking if either one of those values is true.
If the left side of the or
operator is true
, then PHP will not check the value on the right side. This is because only one side needs to prove true for the or
operator to return true
.
Like the and
operator, the or
operator also has two different ways to write it. You can either use two vertical lines (||
) or the “or
” keyword. Both behave the same but have different precedences.
$x || $y
On top of using the two vertical lines (||
), you can also use the “or
” keyword in its place.
$x or $y
xor (xor
) Logical Operator
The xor
logical operator in PHP goes by the name “exclusive or“. The difference between a normal “or
” and an “exclusive or” (xor
) is quite important.
With the “xor
” operator, only one side can be true
for the operator to return true
.
If the left and right values are true
, the “xor
” operator will return false
.
$x xor $y
Unlike the or
operator, xor
will always evaluate both the left and right expressions.
Not (!
) Logical Operator
The NOT (!
) logical operator in PHP works by negating the Boolean value of an expression. Essentially this operator works by returning an inverse of the Boolean the expression resolved to.
This operator works differently as it works purely on a single variable or expression. However, you can easily use it in conjunction with the other logical operators.
If the variable/expression is true
, the not operator will return false
.
Likewise, if the expression is false
, the not operator will return true
.
!$x
PHP String Operators
The PHP language offers two specific operators that you can use to combine strings. Within PHP, you can not use the addition operator to combine strings. Instead, you must use the dot symbol (.
).
The dot (.
) symbol allows you to perform concatenation of strings. This is the method of joining one string to the end of another string.
Below you can view a table showing the the two string specific operators supported by the PHP language.
Operator | Name | Example | Result |
---|---|---|---|
. | Concatenation | $str1 . $str2 | Concatenates $str1 and $str2 |
.= | Concatenation Assignment | $str1 .= $str2 | Appends $str2 to $str1 |
Concatenation (.
) String Operator
The first of these string operators is the concatenation (.
) symbol. You can use this symbol to join one string to another string.
PHP will join the string on the right to the end of the string on the left using the concatenation operator.
$str1 . $str2
Concatenation Assignment (.=
) String Operator
The second operator allows you to combine the concatenation (.
) operator with the assignment operator (=
).
Using this combined operator (.=
), you can join the string on the right to the end of the string on the left. PHP will assign the concatenated string to the left variable.
$str1 .= $str2
The longer way of writing this string operator would be the following.
$str1 = $str1 . $str2
Array Operators in PHP
PHP has an assortment of operators that are used to deal with arrays. While these operators look the same, they behave slightly differently when dealing with arrays.
The biggest difference is how these operators have to consider the element order, type, and value.
The table below will give you an overview of all the operators that work on arrays in PHP.
Operator | Name | Example | Result |
---|---|---|---|
+ | Union | $x + $y | Union of $x and $y |
== | Equality | $x == $y | True if $x and $y are equal.The type and order of elements can be different. |
=== | Identity | $x === $y | True if $x and $y are identical.The type and order of elements must match |
!= | Inequality | $x != $y | True if $x and $y are not equal |
<> | Inequality | $x <> $y | True if $x and $y are not equal |
!== | Non-Identity | $x !== $y | True if $x and $y are not identical |
Union (+
) Array Operator
In PHP the union operator (+
) allows you to join two arrays together, combining the values of one array onto the end of another.
PHP will append the array from the right to the end of the left side array using the union operator (+
).
If the array has conflicting keys, the values on the left array will be preferred, dropping the values on the right side.
$array1 + $array2
Equality (==
) Array Operator
The equality array operator (==
) allows you to compare two arrays and check if they have the same keys and values.
If the keys and values in the two arrays match, this operator will return true
. If they don’t match, it will return false
.
$array1 == $array2
This operator will perform type juggling to match keys or values within the array. Additionally, it will not compare to see if array values are in the same order.
Identity (===
) Array Operator
In PHP, the identity array operator (===
) is the strict alternative to the equality operator. Using this operator, you can compare two arrays to see if they are identical.
An array is considered identical when values, keys, data types, and array order match. Therefore, when all of these conditions are met, the identity operator will return true
. If just one condition doesn’t match, the operator will return false
.
$array1 === $array2
Inequality (!=
or <>
) Array Operator
The inequality array operator (!=
or <>
) is used to compare two arrays and check if their values don’t match. This is the opposite of the equality operator.
This operator works by checking whether any key or value doesn’t match up in either array.
If the arrays don’t match, the inequality operator will return true
. If they do match, the operator will return false
.
$array1 != $array2
You can also write the inequality operator by using the less-than and greater-than (<>
) symbols together.
$array1 <> $array2
The inequality array operator will perform type juggling when it’s looking for a mismatch.
Non-Identity (!==
) Array Operator
The final array operator is called non-identity (!==
). This operator is the more strict alternative to the inequality operator.
This operator will compare two arrays, checking to see if the values, keys, order, and data types don’t match up.
If any of these don’t match up, the non-identity operator will return true
. On the other hand, if the arrays are a perfect match, the operator will return false
.
$array1 !== $array2
PHP Conditional Assignment Operators
The conditional assignment operators are possibly one of the more complicated PHP operators. However, they are also incredibly powerful and allow you to write much cleaner code.
Below is a table including the currently supported conditional assignment operators within PHP.
Operator | Name | Example | Result |
---|---|---|---|
?: | Ternary | $x = expr1 ? expr2 : expr3 | Returns $x Value is expr2 if expr1 is trueValue is expr3 if expr1 is false. |
?? | Null Coalescing | $x = expr1 ?? expr2 | Returns $x Value is expr1 if expr1 exists and is not NULL Value is expr2 if expr1 doesn’t exist or is NULL |
Ternary (?:
) Conditional Assignment Operator
The ternary operator (?:
) in PHP allows you to easily set a value based on whether a specified expression is true. Moreover, it allows you to simplify an if conditional statement down to a single line.
As this is a more complicated operator, let us start with the typical syntax of using the ternary operator.
$x = expr1 ? expr2 : expr3
With the ternary operator, PHP will check the first expression (expr1
) to see if it is “true
” or “false
“.
If it is true
, the value following the question mark (?
) will be returned (expr2
).
If the expression is false
, the value after the semi-colon (:
) will be returned instead (expr3
).
Long-Form Alternative to the Ternary Operator
If you were to write this out using the traditional conditional statement format, you would have the following code instead.
if (expr1) {
$x = expr2;
} else {
$x = expr3
}
Using the ternary operator, you can see that we have condensed this simple if statement into a single line.
Null Coalescing (??
) Conditional Assignment Operator
The final conditional assignment operator that we will be looking at is PHP’s null coalescing operator (??
). This operator allows you to clean up your code further by reducing repetition.
This operator checks if the left variable exists and is not null. If these two conditions are met, the first expression is returned.
Alternatively, if the left expression doesn’t exist or is null, PHP will return the expression on the right instead.
$x = expr1 ?? expr2
Above, you can see how simple this syntax is to use. In addition it reduces the need to call the “isset()
” function as the null coalescing operator handles it.
Long-Form Alternatives to the Null Coalescing Operator
There are two longer ways of writing code that behaves the same that we will quickly go over.
The first way is to use the ternary operator (?:
) and the “isset()
” function.
You can see that you have a double-up call to “expr1
” this way. The syntactic sugar of the null coalescing operator allows you to cut this function call out.
$x = isset(expr1) ? expr1 : expr2
Alternatively, you can write out the same thing using conditional statements.
if (isset(expr1)) {
x = expr1;
} else {
x = exptr2;
}
Conclusion
Hopefully, by this point in the guide, you will understand the various operators supported by the PHP language.
Many operators are supported within the language, so don’t expect to understand them all at once.
If you have any questions about operators in PHP, please leave a comment below.
Additionally, check out our numerous PHP tutorials or check our other coding guides.