In this tutorial, we will be showing you how you can use the str_replace function within PHP.
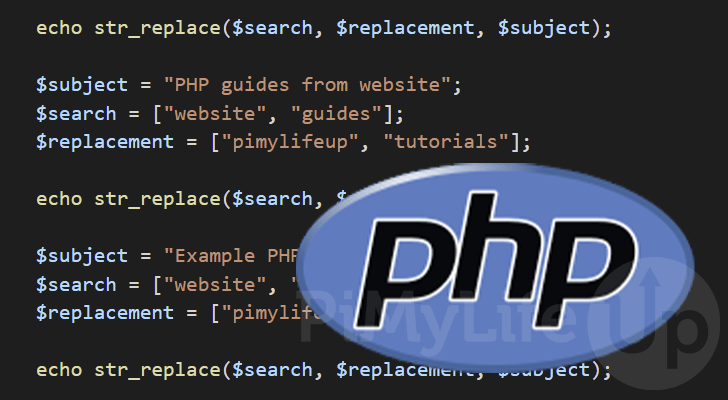
The str_replace function is one of PHP’s many inbuilt functions that help you deal with strings.
The function allows you to easily replace all occurrences of a specified string with another string. You can even use multiple replacements.
This function is useful when you only want to replace a particular part of a string and not the entire string. It can even take a PHP array to find multiple strings with multiple replacements.
We will show you how you can use the str_replace
function in PHP using various examples throughout the following sections.
Table of Contents
- Syntax of PHP’s str_replace Function
- Simple Usage of str_replace Within PHP
- Using Multiple Replacements on a String with str_replace
- Retrieving the Number of Replacements PHP’s str_replace Performs
- Using PHP’s str_replace on Multiple Subjects
- Conclusion
Syntax of PHP’s str_replace Function
Below you can see the syntax for the str_replace function in PHP. It has four parameters, only one of which is optional.
It can return either your modified PHP string, or an array of modified strings depending what is passed into the “$search
” parameter.
str_replace(
array|string $search,
array|string $replace,
array|string $subject,
int &count = null
): string|array
Copy
Let us quickly go over the four different parameters that control the behavior of PHP’s str_replace function.
$search
– This parameter is where you specify the string that you want to replace. Sometimes this parameter is described as the “needle”.
If you have multiple strings you want to replace, then you can pass them in as an array.$replace
– With this parameter, you will set the string with which you want to replace your search parameter.
When used as purely a string, PHP will replace all matched elements with it.
Passing an array into this parameter, you can designate multiple replacements. The order must match the array you used in the “$search
” parameter.
If there isn’t a matching array element, an empty string will be used as the replacement.$subject
– The “subject
” parameter is the string that you want to perform the search and replacement on.
If you specify an array, PHP will perform the search and replacement on each element. This will also make thestr_replace
function return an array.$count
– The final parameter is entirely optional and allows you to retrieve how many replacements were performed
To get the count, you need to pass in a variable. PHP will set the number of replacements to this variable.
We will walk you through some PHP examples that explore how you can utilize these parameters.
Simple Usage of str_replace Within PHP
Let us explore the most basic usage of the str_replace function within PHP.
Below is the most straightforward syntax for using the str_replace
function. All three of the required parameters are shown in this syntax.
We will only pass in strings for each of these parameters and not arrays.
str_replace($search, $replace, $subject);
Copy
Example of a simple usage of str_replace
For this example, let us start by creating a string called “$subject
” and assign it the value “PHP Tutorials at website.com
“. This string will be our “subject
” or “haystack
“.
In our next step, we create a variable named “$search
“, this will be the string we are looking for within our “$subject
” variable. For this example, our needle will be “website
“.
Finally, our last variable will be called “$replacement
” and have the value “pimylifeup
” assigned to it.
With all of our variables declared, we pass each of them into PHP’s str_replace function. Remember to use the order “search
“, “replacement
“, “subject
“.
We use the PHP’s echo function to show us the result of our string replacement.
<?php
$subject = "PHP Tutorials at website.com";
$search = "website";
$replacement = "pimylifeup";
echo str_replace($search, $replacement, $subject);
?>
Copy
After running this piece of PHP code, you will have the following output. You can see how the str_replace function replaced the “website
” part of our string with “pimylifeup
“.
PHP tutorials at pimylifeup.com
Copy
Using Multiple Replacements on a String with PHP’s str_replace
The str_replace function in PHP also allows you to replace multiple strings at once by passing an array of strings into the search parameter (["search1", "search2"]
).
You can specify a replacement for each of the search strings by passing in an array (["replacement1", "replacement2"]
).
When using an array of replacements, the order is essential. For example, “search1
“, will be replaced with “replacement1
“.
Alternatively, you can pass in a string, and PHP will replace all elements with that text ("replacement"
).
To give you a better idea of how all of this works, let us show you a couple of examples.
Example of Multiple Searches and a Single Replacement
For our first example, let us write a quick PHP script that uses str_replace to search and replace multiple strings with a single one.
We start by creating a variable called “$subject
“, to which we will assign the string “PHP tutorials from website
“. This string is what we will be searching for and replacing strings in.
Our next step is to create a variable called “$search
“. We will store an array containing the strings we rant replaced with this variable.
The first element of this array will be "website"
, and the second element "tutorials"
. These strings will be replaced when the “str_replace
” function is run.
The last variable we create in this tutorial is called “$replacement
“, and will be assigned the value “pimylifeup
“. This variable contains our replacement string. PHP will replace all found elements with this value as we aren’t using an array.
Finally, the three variables we just created are passed into the “str_replace()
” function within our PHP script. Remember the order “search”, “replacement”, “subject”.
<?php
$subject = "PHP tutorials from website";
$search = ["website", "tutorials"];
$replacement = "pimylifeup";
echo str_replace($search, $replacement, $subject);
?>
Copy
After running this code, you will end up with the following output. You can see that the str_replace function has run through each of the elements in our “$search
” array and replaced them with the string stored in “$replacement
“.
PHP pimylifeup from pimylifeup
Copy
Example of Multiple Searches with Multiple Replacements
This example shows you how str_replace handles both multiple searches and multiple replacements.
Let us start by declaring the value we will be using in the “$subject
” variable. The string we will be running the str_replace
function on will be “PHP guides from website
“.
For our search variable, we will be specifying two strings within an array. These two elements will be “website
” and “guides
“.
Our next variable will be called “$replacement
” and will also be an array. Within this array, we will specify the replacement text. Remember that the order must match the array you pass into the “$search
” parameter.
For this example we will be replacing “website
” (1st array element), with “pimylifeup
“, and “guides
” (2nd array element) with “tutorials
” by using the array “["pimylifeup", "tutorials"]
“
Our final step is to pass each of these variables into the “str_replace
” function and output the resulting value by using the echo keyword.
<?php
$subject = "PHP guides from website";
$search = ["website", "guides"];
$replacement = ["pimylifeup", "tutorials"];
echo str_replace($search, $replacement, $subject);
?>
Copy
Below is the expected output from running the above PHP example. From this example, you should see how str_replace handles multiple searches and multiple replacements.
Always remember that the order of your elements in the “$searches
” and “$replacements
” arrays matters.
PHP tutorials from pimylifeup
Copy
Example of str_replace in PHP with Mismatched Search / Replacement Arrays
Now that we have shown you standard usages of the str_replace function in PHP let us explore what happens when your “replacements” array is smaller than your “search” array.
Start with a string that you want to perform replacements on. For our case, this will be “Example PHP guides from website.com
“, and we will store it in a variable called “$subject
“.
Next, we create an array to store the strings we search for in our subject. In this case, we will be replacing the words “website
” and “guides
“.
Finally, we will create an array with a single element for our replacements. This replacement element will be “pimylifeup
” and match our “website
” search element.
Since the replacement array is smaller than the search array, PHP will remove each non-matching element from the string. It will act as if you set the replacement for that element to an empty string like ""
.
We then pass all of these variables into the “str_replace()
” function and output the result.
<?php
$subject = "Example PHP guides from website.com";
$search = ["website", "guides"];
$replacement = ["pimylifeup"];
echo str_replace($search, $replacement, $subject);
?>
Copy
From this example, you will end up with the following string. You can see that str_replace replaced our first search text with “pimylifeup
“, and our second was stripped from the string as we specified no replacement.
Example php from pimylifeup.com
Copy
Retrieving the Number of Replacements PHP’s str_replace Performs
Using the “$count
” parameter you can determine how many replacements PHP’s str_replace function performs on the given subject.
For this parameter, you will need to pass in a variable. PHP will set the count to the variable passed into this parameter when it finishes executing
str_replace($search, $replace, $subject, $count);
Copy
By default, PHP’s str_replace function will replace as many occurrences of a string that it finds.
Example of Using the Count Parameter
For this example, let us pass a variable called “$count
” into PHP’s str_replace function. This variable is where PHP will set the count.
For our “$subject
” variable, we will assign the string “Example PHP website from website.com
“. This string is our haystack and what we will be running replacements on.
We then create another variable with the name “$search
” and assign the variable the value “website
“.
For our replacement, we create a variable called “$replacement
” and set the value “pimylifeup
“.
We then pass in our variables into the “str_replace
” function, using the order “$search
“, “$replacement
“, "$subject
“, “$count
“. The result of this string replacement is printed to the output.
Finally, we echo the value now stored in our “$count
” variable. This variable will now hold the number of replacements performed within the string.
<?php
$subject = "Example PHP website from website.com";
$search = "website";
$replacement = "pimylifeup";
$count = '';
echo str_replace($search, $replacement, $subject, $count);
echo $count;
?>
Copy
From this PHP example, you should end up with a result similar to what we have shown below.
You will have the modified string and the number of replacements made. In this example, the count should be printed as “2
“.
Example PHP pimylifeup from pimylifeup.com
2
Copy
Using PHP’s str_replace on Multiple Subjects
The str_replace function in PHP can also handle an array of subjects. This is useful when you want to iterate over multiple strings using the same replacements.
When used like this, the str_replace function will return the modified strings as an array. The returned array will maintain the same order as the one passed in.
Example of using the str_replace Function on Multiple Strings
For this PHP example, let us start with an array of subject strings. We call this array “$subjects
” and declare the various example strings we used in previous examples within it.
We then declare our “$search
” variable with the text “website
“. This is our needle and is present in all four of our subjects.
The “$replacement
” variable used to store our replacement text will be set to “pimylifeup
“.
All three of these variables are passed into the “str_replace
” function. As str_replace will now return an array, we will use the “print_r()
” function to print all elements.
Alternatively you could use PHP’s “var_dump” function instead to see the results of the array.
<?php
$subjects = [
"PHP Tutorials at website.com",
"PHP tutorials from website",
"Example PHP guides from website.com",
"PHP guides from website"
];
$search = "website";
$replacement = "pimylifeup";
print_r(str_replace($search, $replacement, $subjects));
Copy
After running this piece of PHP code, you should get a result similar to what we have shown below. You can see how subject strings were all modified, with the result being returned as an array.
Array
(
[0] => PHP Tutorials at pimylifeup.com
[1] => PHP tutorials from pimylifeup
[2] => Example PHP guides from pimylifeup.com
[3] => PHP guides from pimylifeup
)
Copy
Conclusion
Throughout this tutorial, we have shown you the various ways to use the str_replace function within PHP.
It is the go-to function when you need to perform simple replacements on a string. It is capable of handling multiple subjects as well as multiple replacement strings.
If you have any questions about using the str_replace function, please comment below.
To learn more about PHP and its various built-in functions please check out our PHP tutorials. We also have other coding guides if there is another programming language you are interested in.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support