In this tutorial, we take you through how to use the rand()
function in the PHP programming language.
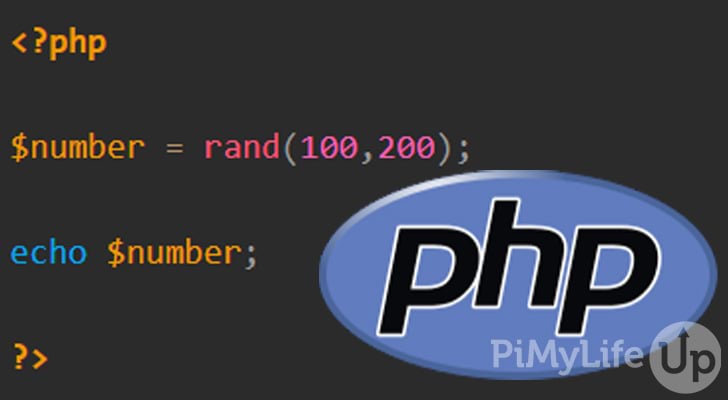
If you require a random value generated within your PHP script, then the rand()
function is perfect. It is super easy to use and understand.
This tutorial will show you how to use the function with arguments and without arguments. We will also touch on how you can generate a random float.
Since PHP 7.1 the rand()
function uses the same random number generator as the mt_rand()
function. Using the same backend as mt_rand()
means the random numbers generated are from a Mersenne Twister random number generator. The generator produces random numbers much faster than the average libc rand function.
It is not recommended you use this function if you require cryptographically secure values. Alternatives, such as random_bytes()
, openssl_random_pseudo_bytes()
, or random_int()
may be used for cryptographic purposes.
This tutorial assumes you are using PHP 7.4 or higher. However, we recommend that you upgrade to the latest PHP version available.
Syntax of the PHP rand() Function
The syntax of the rand function is pretty straightforward, as you can see below. We will discuss the two optional arguments and the return value below.
rand(int $min, int $max): int
The two arguments, min
and max
will need to be type integer. Also, the return value will be an integer.
Specifying values for min
and max
will limit the random value generated to the values you specify. For example, if you want a random number between 100 and 200 (inclusive), you would write the random function like rand(100, 200)
.
Both arguments are optional, and without them, PHP will return a random value between 0
and getrandmax()
. If you specify arguments, you will need to enter both. If you only enter one, you will receive an error.
It is important to mention that there is another random function you can use within PHP, and that function is mt_rand()
.
The mt_rand()
function is essentially the same as rand()
in PHP versions 7.1 and higher. The only difference is that mt_rand()
does not allow max
to be smaller than min
.
mt_rand(int $min, int $max): int
Examples of the rand() Function
We will take you through two examples of using the rand()
function in PHP. In one example, we will use no parameters, and in the other, we will use two parameters. We will also cover how you can use rand()
to generate random float numbers.
Using no Arguments
In the example below, we do not use any arguments when using the PHP rand function. Therefore, our number may range from 0
to the value determined by getrandmax()
.
<?php
$number = rand();
echo $number;
?>
In the output below, you can see the number printed when we first ran the script. Every time you run the script, you will get a different number.
2112124673
Using Arguments
This example will use 100 as the minimum argument and 200 as the maximum argument. Using these two values as arguments means our random number will be between 100 and 200 (inclusive).
<?php
$number = rand(100,200);
echo $number;
?>
On our first run, the random number returned was 190. Every time we run the PHP script, PHP will return another random number between 100 and 200.
190
Generate a Random Float using rand()
If you want a float rather than an integer, you can achieve this by dividing the result of the PHP rand function. In the example below, we divide our result by 1000 to get a random float rather than an integer.
<?php
$number = rand(1000,2000)/1000;
echo $number;
?>
Our result is 1.105, which means rand()
produced an integer of 1105, which we then divided by 1000. Of course, you can tinker with the math to achieve the values you want for your program.
1.105
Conclusion
I hope this short guide has taught you the basics of using the rand function in PHP to generate a random number. This function is extremely useful when you need a random number generated.
There are many more functions in PHP that you might find extremely useful for programming your next script. For example, you might need to generate an md5 hash or check if a value is numeric. I highly recommend checking out our many PHP tutorials if you want to learn more.
If we can improve this tutorial, please do not hesitate to let us know by leaving a comment below.