This guide will show you how it is possible to create a directory in PHP by using the mkdir function.
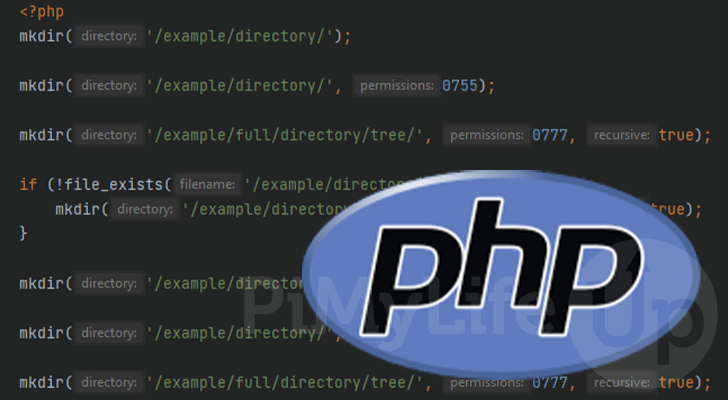
The mkdir function in PHP is a simple yet powerful function that allows you to create a directory wherever the running agent has permission.
At its most straightforward usage, you can create a single directory in the specified path. However, you can even use this function to create a whole directory tree by enabling its “recursive
” functionality.
It is a core part of PHP and is helpful for various cases where you need to create folders on the fly. For example, you might want to create directories based on the current date when handling uploads within PHP.
You may be interested in what each of these parameters does, so let us quickly go over them. We will show you a fair chunk of these in the later sections.
Definition of the mkdir Function in PHP
Before we proceed further, we should first touch on how the mkdir function is defined in PHP.
Below you can see how exactly the mkdir function is defined within PHP. It sports four parameters, but only the first one is required to utilize its functionality.
This function will return true
, if the directory was successfully created and false
if it failed.
mkdir(
string $directory,
int $mode = 0777,
bool $recursive = false,
?resource $context = null
)
Copy
You may be interested in what each of these parameters does, so let us quickly go over them. We will give examples of how each parameter is used later in this guide.
The Directory Parameter
The “directory
” parameter is required and is used to specify the directory path that you want to be created by this function.
The mkdir function in PHP has full support for both relative (./newdir/
) and absolute paths (/var/www/php/newdir
).
However, it is best to use absolute paths here as the current working directory might not be what you think.
If you want to create a directory relative to the current PHP file, you will want to append your directory with “__DIR__
“. This constant contains the current work directory.
For example, using the relative path example before, we would write “__DIR__ . '/newdir/'
“.
The mode Parameter
Using the “mode
” parameter, you can set the permissions that should be assigned to the folder when it is created.
By default, PHP will create the directory with the permissions “0777
” the widest possible access. The owner, group, and everyone else will have full access to this directory.
The mode value should typically be defined as an octal, meaning you should use the following format.
- The first number must always be a 0.
- The second number specifies the permissions for the owner.
- The third number sets the permission for the group that owns the directory.
- Finally, the fourth number sets the permissions for anyone else.
The value for each of these permission groups is calculated by adding the following numbers.
- 1 = Execute Permissions
- 2 = Write Permission
- 4 = Read Permission
For example, if we wanted our group owner only to have read and write permissions, we would use “6
” for that group.
You can learn more about how Linux handles permissions by checking out our chmod command guide.
Please note that if you are on a Windows-based system, this option will not do anything.
The recursive Parameter
PHP’s mkdir function will only attempt to create the last segment in a path by default.
For example with the path “/var/www/example/
“, PHP will only create the ending “/example/
” directory. If the previous directories don’t exist, then the function will fail.
You can set the “recursive
” parameter to true
to get around this. When set to true
, PHP will run through each part of the path, creating them if they don’t exist already.
The context Parameter
The context parameter is optional and allows you to control the file handle that PHP’s mkdir function will utilize.
For most cases, you will not need to utilize this parameter. It is only useful when you need to control the file stream.
Basic Usage of the mkdir Function in PHP
Let us start with the most basic way to use the mkdir function within PHP.
The simplest way to use mkdir is to pass in the directory that you want to create. The function will assume that the default values should be used for all other options.
With the following example we will be creating the directory “/example/directory/
“.
<?php
mkdir('/example/directory/');
?>
Copy
As recursive is set to false by default, the “/example/
” part of this directory must already exist. Otherwise, mkdir will return false
, and fail to create the directory.
Likewise, as we aren’t setting the “mode
” parameter, the default permissions of “0777
” are set when this folder is created.
Setting Permissions on Folder Creation with mkdir on PHP
The mkdir function in PHP allows you to set the permissions on the folders that it creates by utilizing its mode option.
You will still need to specify the directory you want to create for the first parameter. For our example below, we use the directory “/example/directory/
“.
We can set the permissions to be assigned to this new folder with the second parameter. This needs to be an octal value.
- The first number must remain
0
as this value is an octal. - The second number is the user privileges. In our example below, we set this to
7
.
Using 7 gives the user execute (1
), write (2
), and read (4
) privileges. - With the third number, we set the permissions for the group that owns the file.
With our example, we will set this number to5
. This gives the group execute (1
) and read (4
) permissions. - The last and fourth number allows you to set the permission for all other users.
Like with the group permission, we also set this to 5 for our example.
<?php
mkdir('/example/directory/', 0755);
?>
Copy
When this bit of PHP is executed, it will use the mkdir function to create the directory “/example/directory/
“, with the permission “0755
“.
Creating Parent Directories using PHP’s mkdir Function
The final parameter that we will be showing you how to use is the recursive one. This is one of the most useful functions of PHP’s mkdir function, as it allows you to create a whole directory branch in a single function call.
To use this, we must define the directory and mode first. We will be using “/example/full/directory/tree/
” for our directory, and for the mode, we will be using the default “0777
“.
Now all we need to do is set the third (recursive) parameter to true.
<?php
mkdir('/example/full/directory/tree/', 0777, true);
?>
Copy
With “true
” passed into the recursive parameter, mkdir will ensure that each part of the directory is created.
Create a Folder if it Doesn’t Exist Using mkdir in PHP
While PHP’s mkdir function helps create a directory, it doesn’t perform any checks to see if that directory exists before firing.
When used on a directory that already exists, the mkdir function will throw a warning indicating that it already exists.
To avoid this warning from occurring within the code, we can utilize PHP’s “file_exists
” function. Using this function, we can check if the directory exists before proceeding.
Below we have a simple PHP if conditional statement using the file_exists()
function to check if the directory does not (!
) exist.
Only if the file file_exists
function returns false
will our mkdir
function call be ran and our directory, “/example/directory/tree/
” be created.
<?php
if (!file_exists('/example/directory/tree/')) {
mkdir('/example/directory/tree/', 0777, true);
}
?>
Copy
Conclusion
By the end of this tutorial, we hope that you now understand how the mkdir function works in PHP.
This function is helpful as it allows you to create directories within PHP. It even allows you to set permissions on the new folders and recursively create any directories in the provided path.
Of course, the user that PHP is running under will need permission to create folders, so make sure you have your permissions set correctly.
Please comment below if you have any questions about using the mkdir function.
Be sure to check out our many other PHP tutorials, and our other coding guides.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support