In this short tutorial, we will be touching on how to write a while loop in PHP.
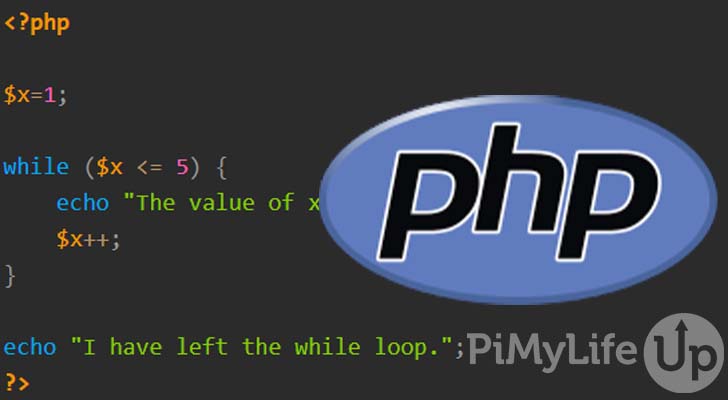
Learning how to use a while loop is extremely important if you are new to programming. Luckily, while loops have the same behavior and syntax across most programming languages. But, of course, there are always some exceptions.
A while loop will check a condition before executing the code inside the loop. This style of loop is known as an entry control loop. PHP will execute the code inside the loop if the condition is true. However, if the condition is false, it will skip past the code inside the loop and continue with executing the code after the loop.
You can nest while loops within each other but you need to be careful as multiple nested loops can cause performance problems, especially when dealing with large data sets.
An alternative to a while loop is known as a do-while loop. A do-while loop is the opposite and will execute the code inside the loop before checking the condition. This process means that the code inside the loop will always run at least once.
Table of Contents
while Loop Syntax
There are a couple of different ways you can structure a while loop. They are pretty straightforward and not much more complicated than a simple if statement.
A while loop will continually run until the condition within the brackets turns false. If the condition is never met, PHP will simply move on past the while loop without executing the code inside.
The example below uses curly brackets to enclose the code we wish to run if the condition is true. This style is most likely the one you will encounter when reading through PHP code.
while (condition) {
// Code here will be executed if the condition is met
}
Copy
You can also write a while loop without curly brackets and simply end the while loop using endwhile
. A colon will also need to be placed after the closing bracket on the condition statement.
while (condition):
// Code here will be executed if the condition is met
endwhile;
Copy
How to Use a while Loop in PHP
In this tutorial, we will touch on the basics of how to write a simple while loop in PHP. Below are a couple of basic examples of writing a while loop.
I highly suggest learning PHP operators so you can build good quality conditions for the while loop.
while Loop Using Curly Brackets
The code below is very basic but will show you the fundamentals of a while loop in PHP.
At first, we create a variable called x
and assign it the value of 1
.
In the while loop, we have a condition that states for as long x
is less or equal to 5
the result is true. For as long as the condition returns true, the while loop will run.
Inside the while loop, we echo a string that includes the value of x
. Afterward, we increment the value of x
by 1
.
<?php
$x=1;
while ($x <= 5) {
echo "The value of x is " . $x . "<br>";
$x++;
}
echo "I have left the while loop.";
?>
Copy
As you can see below, we looped until x
reached 6
and turned the condition ($x <= 5
) false.
Once the condition was false, we continued to our echo statement after the while loop.
The value of x is 1
The value of x is 2
The value of x is 3
The value of x is 4
The value of x is 5
I have left the while loop.
Copy
while Loop Using a colon and endwhile
In this example, we will not be using curly brackets but the colon and endwhile to enclose our code. However, the behavior is exactly the same as using the while loop with curly brackets.
In the code below, we first create a variable called y and assign it the value of 5
.
The while loop condition states that for as long as y
is greater than 0
, continue to the next iteration.
Our code inside the while loop will output the value of y
before subtracting 1
off the value of y
.
<?php
$y=5;
while ($y > 0):
echo "The value of y is " . $y . "<br>";
$y--;
endwhile;
echo "I have left the while loop.";
?>
Copy
In our output below, you can see the value of y
goes down on every loop until the last output of y
is 1
.
On the last attempt to loop, y
is 0
and no longer matches the requirements of the loop condition. Since the condition is false, it skips the inside code of the loop and prints out the last echo statement after our loop.
The value of y is 5
The value of y is 4
The value of y is 3
The value of y is 2
The value of y is 1
I have left the while loop.
Copy
Breaking Out of a while Loop
There may be times when you want to end a while loop prematurely. For example, you find a piece of data you were looking for and now no longer need to continue looping through the rest of the data. To do this in PHP, you simply need to use break
.
In the code below, we create a variable called z
and assign it the value of 1
.
In the while loop, we have a condition that states for as long as z
is less or equal to 5
continue to loop.
Inside the while loop, we echo the value of z
. Afterward, we increment the value of z
by 1
.
We have an extra if statement that declares if z
equals 3
, break
out of the loop.
<?php
$z=1;
while ($z <= 5)
{
if ($z == 3){
break;
}
echo "The value of z is " . $z . "<br>";
$z++;
}
echo "I have left the while loop.";
?>
Copy
As you can see in our output below, the code stopped outputting at the value of 2
. It does not output 3
as our break statement is before our echo statement.
The value of z is 1
The value of z is 2
I have left the while loop.
Copy
Using continue Inside a while Loop
You may sometimes need to skip processing a value but continue looping through the data. For example, you can use continue
to tell PHP to move onto the next iteration without executing any more of the code.
In the code below, we create a variable called w
and assign it the value of 1
.
For the while loop, we have a condition that states for as long as w
is less or equal to 5
continue to loop.
Inside the while loop, we echo the value of w
. Afterward, we increment the value of w
by 1
.
We also have an extra if statement that declares if w
equals 2
, increment w
by 1
, and continue
to the next iteration.
<?php
$w=1;
while ($w <= 5)
{
if ($w == 2){
$w++;
continue;
}
echo "The value of y is " . $w . "<br>";
$w++;
}
echo "I have left the while loop.";
?>
Copy
As you can see in our example below, the code does not output anything when w
equals 2
. However, unlike break
, it continued with the loop and printed the remaining numbers until the condition turned false.
The value of w is 1
The value of w is 3
The value of w is 4
The value of w is 5
I have left the while loop.
Copy
Infinite while Loop
You need to be careful of creating an infinite loop. For example, you may accidentally write a condition that will never turn false.
On the odd occasion, you may want to do this deliberately, for example, when you need a server to run through processes continuously. However, it is unlikely you will need to use infinite loops as a beginner, and there are often techniques that achieve the same task more efficiently.
To cause the while loop to be stuck in an infinite loop, you can use 1
or true
as the condition, as shown in the example below.
<?php
while (1) {
echo "Stuck in here forever";
}
?>
Copy
Luckily, if you do write an infinite loop by accident, PHP has a timeout of 120 seconds. After 120 seconds, PHP will terminate the script. You can change this behavior by editing the PHP ini file.
To terminate a script stuck in an infinite loop, you may need to kill the process, or if possible, you can use CTRL + C within the terminal.
Conclusion
A while loop is incredibly powerful, and you will likely find yourself using them in your next PHP project quite a bit. I hope that this tutorial has covered all the basics and that you now have a good understanding of while loops in PHP.
There are a few other types of loops that you will find extremely helpful when writing your next PHP project. For example, you may find for loops, do-while loops, or even foreach loops helpful. Each loop has its benefits, so I recommend checking out each of them.
If there is anything that we can improve on with this tutorial, let us know by leaving a comment below.
That was insightful and educative. I love to learn more