In this guide, we will take you through the basics of using the PHP $_POST global variable for handling incoming POST data.
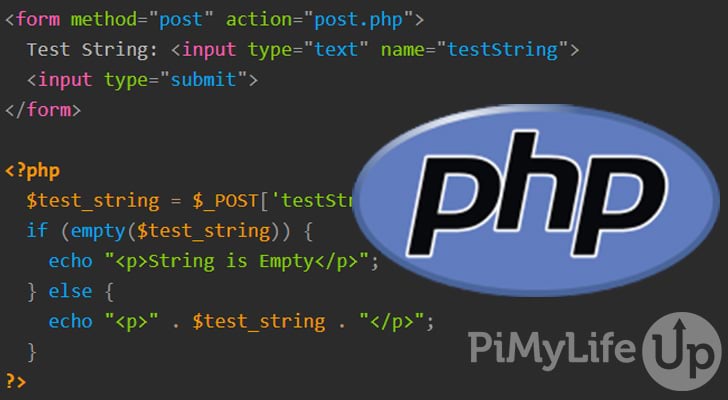
The $_POST super global (superglobal) variable within PHP is an associative array of variables containing data sent via an HTTP POST request. Using this variable, you can access data sent to your PHP script from a HTML form. You can also send form data via JavaScript.
An alternative to $_POST is the $_GET super global variable. The $_GET variable allows you to access data sent via a GET request. The parameters for a GET request are included in the URL, for example, https://example.com/post.php?test=example
. The “test
” text is the parameter name and “example
“, is the value.
This tutorial will go through the basics of the HTTP POST method and how you can use the PHP $_POST global variable to process a POST request.
What is the HTTP POST Method
The POST method sends data to a server using the Hypertext Transfer Protocol (HTTP). POST and GET are the two most common types of HTTP methods.
There are several key points on POST requests which I will list below.
- You can use POST requests for sending sensitive data (HTTPS is recommended).
- A POST request will never be cached.
- POST requests are not stored in your browser history and cannot be bookmarked.
- There is no restriction to the data length with a POST request.
You can view the HTTP request type by looking for the method header within the request.
:authority: development.local
:method: POST
:path: /post.php
:scheme: https
accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.9
accept-encoding: gzip, deflate, br
accept-language: en-US,en;q=0.9
Example of using $_POST in PHP
To receive a POST request in PHP, we will need to use the $_POST
super global variable. Since it is a global variable, you can use the $_POST
variable in any scope.
The $_POST
super global variable is an associative array of variables containing POST request data sent to the script. Using this variable is very straightforward, as we will quickly explain below.
The script further down this page demonstrates how you can use the $_POST
super global variable to receive and process HTTP POST requests. We will go through each part of the script below.
Firstly, we create an HTML form that uses the HTTP POST method for sending data. In the form action attribute, we specify our post.php
file as the URL to process the form data submission.
<form method="post" action="post.php">
Inside our form, we have an input that accepts text and will be accessible under the name “testString
” within the $_POST
super global variable. Ensure that each input has a name attribute if you want it available within $_POST
, as using id will not work.
Next, we have an input of the type “submit“, allowing you to submit the form.
Test String: <input type="text" name="testString">
<input type="submit">
Below the HTML is our PHP script. First, we create a variable $test_string
and assign it the testString
value from our form using the $_POST
super global variable.
$test_string = $_POST['testString'];
Lastly, we do a simple if else statement where we echo the string if it is not blank. Otherwise, we print a message claiming our string is empty.
if (empty($test_string)) {
echo "<p>String is Empty</p>";
} else {
echo "<p>" . $test_string . "</p>";
}
Below is the full PHP script, which will work if you want to test it yourself.
<form method="post" action="post.php">
Test String: <input type="text" name="testString">
<input type="submit">
</form>
<?php
$test_string = $_POST['testString'];
if (empty($test_string)) {
echo "<p>String is Empty</p>";
} else {
echo "<p>" . $test_string . "</p>";
}
?>
The screenshot below demonstrates how our basic form looks within a web browser. On submission, the form sends a POST request to post.php
, which processes the string and outputs the text underneath the form.
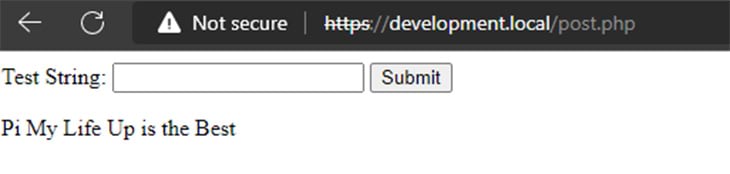
Conclusion
I hope you now have a decent understanding of HTTP POST requests and how you can view them using the PHP $_POST super global variable. If you plan on doing a lot of server communication, you must understand both of these concepts.
We have plenty more PHP tutorials that you might find useful if you want to learn more. For example, we have tutorials that touch on handling variables in PHP, writing if-else statements, and more.
Please let us know if you notice a mistake or if an important topic is missing from this guide.