In this tutorial, we will discuss how to write a do-while loop in PHP.
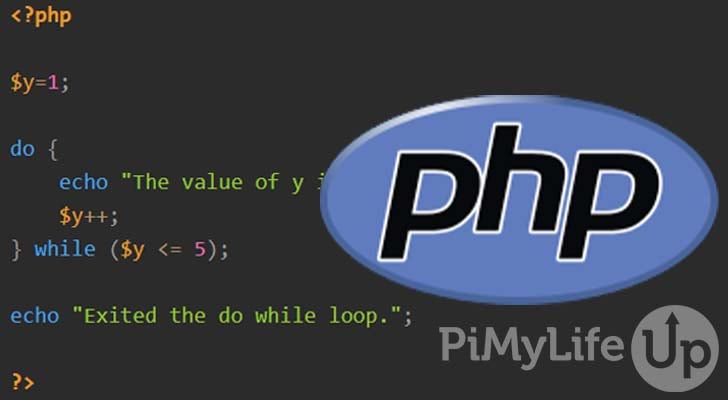
The main difference between a do-while loop and a while loop is the location of the condition expression. For a do while
loop, the condition is at the end of the loop rather than the start. The loop will always run at least once as the condition is at the end.
To explain further, PHP will enter a do while
loop without checking any conditions. It will run through the code block until it reaches the end. Afterward, it will check a condition, and if it returns true, it will go back to the start. Otherwise, if the condition returns false, it will leave the loop and proceed to execute the rest of the code.
There will be times when using a do-while loop makes more sense than a while loop. However, you will find yourself making use of a plain while loop most of the time.
You can nest do-while loops but plan your code carefully to avoid performance issues. For example, heavily nested loops may become slow when dealing with large data sets. There may be better solutions for solving the problem.
Table of Contents
do-while Loop Syntax
The syntax for a do while
loop is pretty straightforward and is somewhat similar to a regular while loop. The code block starts with “do“, followed by the curly brackets. At the end of the block, we have “while” followed by our loop condition.
A do-while loop will run at least once before checking the condition within the brackets at the end of the code block. If the condition equates to true, the code will loop back to the start. Otherwise, it will exit the code block.
The example below shows how to structure a do-while loop in PHP.
do {
// Code here will be executed at least once
} while (condition);
How to Use a do-while Loop in PHP
In this tutorial, we will cover the basics of writing a do-while loop in PHP. We also cover some of the other methods you might use alongside a loop, such as continue or break statements.
It is important to have a good understanding of PHP operators to build conditions for use in loops, if-else statements, and more.
Writing a Standard do-while Loop
Our first example will show you how a basic do-while loop works in PHP. I will quickly explain the code below.
Firstly, we create a variable named y
and give it the value of 1
.
Next, we create our do-while loop by starting with do
and a curly bracket. Inside our loop, we echo the current value of y
and then increment y
by 1
. Lastly, we close our loop with a curly bracket followed by while
and the condition expression. The condition states that y
must be less than or equal to 5
.
Lastly, we echo
a string that lets us know that we are no longer in the loop code block.
<?php
$y=1;
do {
echo "The value of y is " . $y . "<br>";
$y++;
} while ($y <= 5);
echo "Exited the do while loop.";
?>
The output below shows that our loop iterated until y
equaled 5
. Afterward, PHP exits the loop and prints out our last line of text.
The value of y is 1
The value of y is 2
The value of y is 3
The value of y is 4
The value of y is 5
Exited the do while loop.
Breaking Out of a do-while Loop
There will be times when you may want to exit out of a loop early if a certain set of conditions are met. Simply use the break
statement to exit out of the loop.
The code below shows you how to break out of the do-while loop early.
<?php
$z=1;
do {
if ($z == 3){
echo "z is equal to 3, exit early" . "<br>";
break;
}
echo "The value of z is " . $z . "<br>";
$z++;
} while ($z <= 5);
echo "Exited the do while loop.";
?>
In the output below, you can see that the loop ended early once z
was equal to 3
.
The value of z is 1
The value of z is 2
z is equal to 3, exit early
Exited the do while loop.
Using continue Inside a do-while Loop
You can use the continue statement whenever you want to skip an iteration. This technique is handy if you do not want to process certain data whenever a condition is met. However, if you want to end the loop completely, you need to use break.
In our example below, we skip the iteration whenever x
equals 3
.
<?php
$x=1;
do {
if ($x == 3){
$x++;
continue;
}
echo "The value of x is " . $x . "<br>";
$x++;
} while ($x <= 5);
echo "Exited the do while loop.";
?>
As you can see in our output below, we did not print any data when the value of x
was equal to 3
. This code is a very basic example, and you can do more complex conditions on when to run the continue statement.
The value of x is 1
The value of x is 2
The value of x is 4
The value of x is 5
Exited the do while loop.
Conclusion
The do-while loop in PHP is incredibly useful, but you will find that it is not as commonly used as a regular while loop. With that said, it is incredibly important that you understand how these loops work as you may need to use them in the future.
There are other types of loops that you can use in the PHP programming language. I highly recommend that you take the time to learn more about for loops, regular while loops, and foreach loops. All these different types of loops have pros and cons, so be sure to learn about them.
If you notice we can improve this tutorial, please do not hesitate to let us know by leaving a comment below.