This tutorial will show you how to use the crypt() function in PHP.
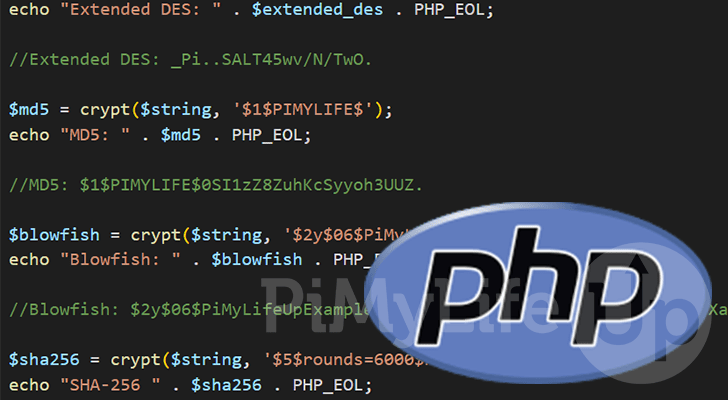
The crypt() function in PHP allows you to generate a hash of the specified string using a variety of hashing algorithms.
Some of this function’s supported hashes include blowfish, SHA-256, and MD5.
If you are planning on using this to encrypt passwords, we recommend that you use the password_hash()
function instead. This function is a wrapper for PHP’s crypt() function but uses strong encryption and a strong salt by default.
Over the following few sections, we will show you how to use the crypt() function to generate each of the supported hashes for a chosen string. Since this is controlled through its “salt” parameter, this isn’t the most straightforward function to utilize.
Syntax of PHP’s crypt() Function
Let us start by exploring the syntax of the crypt function within PHP. The syntax shows us how this function is written and will give you a basic idea of to utilize it.
With this syntax, you can see that the crypt() function has two parameters and returns a string which will be your hash.
crypt(string $string, string $salt): string
Copy
$string
– This parameter is where you will specify the string you want to be hashed by PHP.
Depending on the hash type, this string may be truncated during the hash generation, meaning it won’t consider the whole string.$salt
– You will use the salt to specify the hashing method as well as the “salt” that is applied to the hash.
A salt is a way of changing the result of a hash. You will only be able to generate the same hash with the same salt. This is to prevent someone from just generating a ton of hashes for possible passwords and getting matches very quickly.
The crypt() function will return the hashed version of your passed-in string. The length of this string depends heavily on what salt you pass into the crypt function.
Supported Hash methods for PHP
As mentioned earlier, the crypt function in PHP has support for several different hashing methods. The hashing method is specified through the salt parameter using a specific set of characters.
We will go over each supported hash type and how they are specified in the following sections. You should research which one best suits your needs.
CRYPT_STD_DES
The first hash we will be looking at is the Standard DES-based hash. This hash is specified using a 2-character salt.
Valid characters for this type of hash is “.
“, “/
” “0-9
” “A-Z
” and "a-z
“.
For example, PHP would consider the following a valid salt for the “CRYPT_STD_DES
” hash.
pi
CRYPT_EXT_DES
An extended version of the DES-based hash has a few benefits, including the support for a longer salt.
The salt for this hashing method must be nine characters long and start with the underscore (_
) character.
The algorithm uses the first four characters after the underscore as the iteration count. Each character is 6-bits, with the first character being the least significant.
The next four characters are the salt that is applied to your hash
These values are encoded from 0 to 64 using the characters “.
/
0-9
A-Z
a-z
“. The period (.
) will be 0, and the lowercase z
will be “64
“.
Below is an example of setting a “CRYPT_EXT_DES
” hash using the salt parameter of PHP’s crypt() functions.
_Pi..SALT
CRYPT_MD5
Another hash supported by PHP’s crypt() function is MD5. While now considered a relatively weak hash, it still has its usage outside of security.
You can also use the md5() function in PHP to achieve the same thing as utilizing this hash type.
To specify the MD5 hash with this function, you need to start your salt with “$1$
” (hash identifier) followed by eight characters (salt), and finally end the string with another dollar sign symbol ($
).
The following is an example of setting an MD5 salt with the crypt() function in PHP.
$1$PIMYLIFE$
CRYPT_BLOWFISH
The crypt() function also supports the bcrypt version of the blowfish cipher that was designed as a solid alternative to the aging DES standard. If you encrypt data like passwords, this is the algorithm you will likely want to use in PHP.
Thanks to the design of the algorithm, you can progressively make the hash stronger over time by increasing the number of iterations. It strengthens the hash as it takes more processing power to generate a hash slowing down the process. Unfortunately, a fast algorithm is not suitable for encryption.
To use the blowfish encryption standard in PHP, you need to start your salt with “$2y$
“. While you can also use “$2x$
” and “$2a$
“, they both will utilize a weaker hashing method.
After the hash identifier, you need to specify the cost of the hash using a two-digit parameter This cost must range between “04
” and “31
“. The higher the number, the more processing power it will take to generate a hash.
Finally, the hash is completed with a 22-character hash. This hash must only use characters from the following “.
“, “/
“, “0-9
“, “A-Z
“, and “a-z
“.
The following is an example of a valid salt for the blowfish standard on PHP with the crypt() function.
$2y$06$PiMyLifeUpExampleSalt1
CRYPT_SHA256
Using PHP’s crypt() function also allows you to generate a SHA-256 hash of your specified string.
To generate the hash using PHP, you need to start your salt with “$5$
“. This tells the crypt() function that you want to use the SHA-256 hash.
Next, you can specify how many rounds of hashing should be executed. These rounds are similar to blowfish’s cost option. If you want to, you need to write “rounds=N$
” after the hash specifier. N
is the number of rounds to execute.
Blowfish has a minimum of 1,000
rounds and a maximum of 999,999,999
. Any value that is outside of this range will be rounded to the nearest value.
If no number is specified, then PHP will, by default, use 5,000
rounds.
Lastly, you need to specify a sixteen-character salt followed by the dollar sign symbol ($
).
Below are two examples of using the SHA-256 hash with PHP’s crypt() function. In the top example, we use the rounds option, and in the second one, we don’t.
$5$rounds=6000$PiMyLifeUpExampl$
$5$PiMyLifeUpExampl$
CRYPT_SHA512
The SHA-512 hash is a longer version of the SHA-256 hash. Since it is essentially an extended version of the hash, its usage is very similar.
The only difference is how you tell PHP’s crypt() function that you want to generate an SHA-512 hash.
To generate an SHA-512 hash, you will need to start your salt with “$6$
“.
Following the hash identifier, you can then specify the number of rounds you want used to generate the hash. Like SHA-256, this is written using “rounds=N$
” where “N
” is the number of rounds.
Finally, you can write your 16-character salt followed by the dollar sign symbol ($
).
Two examples of a valid SHA-256 hash are what we have written below. One uses the rounds parameter. One does not.
$6$rounds=6000$PiMyLifeUpExampl$
$6$PiMyLifeUpExampl$
Using the crypt() Function in PHP
This section will show you how to use the crypt() function within PHP. You will use the various salts we learned previously and see how they work in actual code.
For this example, we will write a short PHP script that will generate a hash using each of the crypt() functions supported hashes.
1. Let us start this script by declaring a variable called “$string
“, which we will assign the PHP string "PiMyLifeUp"
.
Throughout the rest of this script, we will use this one string for each of our different hashing types. By doing this, you can see how each of PHP’s crypt() function’s different hashes look, and how they are used.
$string = "PiMyLifeUp";
Copy
2. With our first example, we will be using the PHP crypt() function to produce a standard DES hash of our string.
We do this by first passing “$string
” into the first parameter of the crypt() function. Next, we pass in a simple two-character for the salt. In our example, this will be “pi
“.
Finally, we use PHP’s echo keyword to output the text “Standard DES” followed by the hash we just generated.
$standard_des = crypt($string, "pi");
echo "Standard DES: " . $standard_des . PHP_EOL;
Copy
3. For our next example, we will generate an extended DES hash of our “$string
” variable.
This time when we specify the salt we will be using “_Pi..SALT
“. This salt uses the format expected by the crypt() function.
The result is output using the echo keyword and will be identified by the “Extended DES
” label.
$extended_des = crypt($string, "_Pi..SALT");
echo "Extended DES: " . $extended_des . PHP_EOL;
Copy
4. Let us now show you how you can use the crypt() function in PHP to generate an MD5 hash of a string.
We first pass in our “$string
” variable to the crypt function. Next, we pass in the salt. We start this salt with “$1$
” to tell PHP to generate an MD5 hash. After the hash identifier, we then specify an eight-character salt.
Like the previous example, we print out the generated MD5 hash by using echo.
$md5 = crypt($string, "$1$PIMYLIFE$");
echo "MD5: " . $md5 . PHP_EOL;
Copy
5. Generating a blowfish cipher is as easy as the other hashes. You just need to pass in the correctly formatted salt.
To showcase this we use the salt “$2y$06$PiMyLifeUpExampleSalt1
“. By starting this salt with “$2y$
“, PHP can tell that we want to generate a blowfish hash. Following this, we specify that we want to run “06
” iterations of the hash.
Finally, to finish off our salt, we include a 22-character salt.
$blowfish = crypt($string, "$2y$06$PiMyLifeUpExampleSalt1");
echo "Blowfish: " . $blowfish . PHP_EOL;
Copy
6. Next, we will showcase how you can use the crypt() function in PHP to generate an SHA-256 hash of a specified string.
PHP identifies a salt for SHA-256 by the presence of “$5$
” at the start of the salt. You can then optionally specify how many rounds the hash should run before completing. The more rounds, the longer the hash takes to complete.
We then output the resulting hash to the screen by utilizing the “echo
” keyword.
$sha256 = crypt($string, "$5$rounds=6000$PiMyLifeUpExampl$");
echo "SHA-256 " . $sha256 . PHP_EOL;
Copy
7. Our final example shows you the final hash type that PHP’s crypt() function supports. That hash type is SHA-512.
To tell the crypt() function to generate an SHA-512 hash, you need to start your salt with “$6$
“. Optionally, you can set the number of rounds to generate the hash. Finally you end the string with a 16 character salt.
$sha512 = crypt($string, "$6$rounds=6000$PiMyLifeUpExampl$");
echo "SHA-512 " . $sha512 . PHP_EOL;
Copy
8. The final version of your code should end up looking like what we have shown below.
<?php
$string = "PiMyLifeUp";
$standard_des = crypt($string, 'pi');
echo "Standard DES: " . $standard_des . PHP_EOL;
$extended_des = crypt($string, '_Pi..SALT');
echo "Extended DES: " . $extended_des . PHP_EOL;
$md5 = crypt($string, '$1$PIMYLIFE$');
echo "MD5: " . $md5 . PHP_EOL;
$blowfish = crypt($string, '$2y$06$PiMyLifeUpExampleSalt1');
echo "Blowfish: " . $blowfish . PHP_EOL;
$sha256 = crypt($string, '$5$rounds=6000$PiMyLifeUpExampl$');
echo "SHA-256 " . $sha256 . PHP_EOL;
$sha512 = crypt($string, '$6$rounds=6000$PiMyLifeUpExampl$');
echo "SHA-512 " . $sha512 . PHP_EOL;
?>
Copy
9. After running the code above, you should end up with the following result.
With this result, you can see the different hashes generated for our “PiMyLIfeUp
” string.
Standard DES: pip8SglU2x.0M
Extended DES: _Pi..SALT45wv/N/TwO.
MD5: $1$PIMYLIFE$0SI1zZ8ZuhKcSyyoh3UUZ.
Blowfish: $2y$06$PiMyLifeUpExampleSaltufxceQbVRwrEHQD50rsQd41MN0rX4Xaq
SHA-256 $5$rounds=6000$PiMyLifeUpExampl$RJtEAkxuB/qAQWAoLgoODNNL.g8/CNpKUrECCgStaA4
SHA-512 $6$rounds=6000$PiMyLifeUpExampl$jT9tvq71uXwD82zzwMgUtU85IOUTvkv6p1rCCzlQzCvNq18P0q/7KYbr3cfQdggL3dJ0FgR8.jD9mQUSM/7l31
Conclusion
Hopefully, at this point, you will now have a good understanding of how you can use the crypt() function in PHP.
This function allows you to generate hashes of any string you pass in. While not the most simple function to use, it is very powerful. Additionally, it has a support for various useful hashing algorithms.
Please comment below if you have issues using the crypt() function.
We also have a wealth of other PHP tutorials if you want to explore this language further. If you want to learn a new programming language, check out our many other coding guides.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support