This tutorial will show you how to encode and decode a string in the base64 format in PHP.
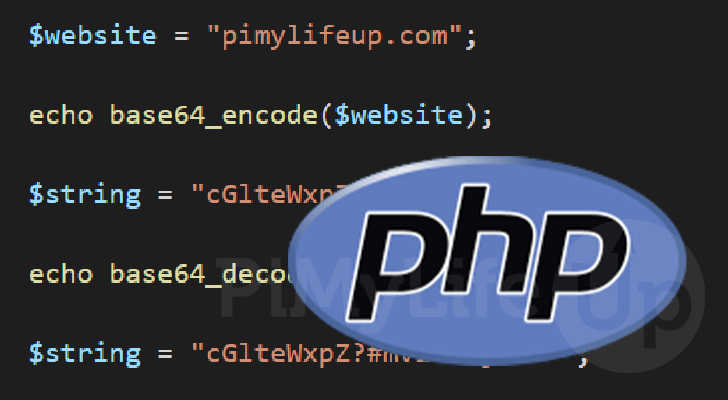
Base64 is a useful encoding type that allows you to encode a string in PHP that you can easily decode.
PHP utilizes the MIME Base64 standard for its encoding. This standard was originally designed to help send binary data in text-based protocols like emails.
Something like an image would be encoded in this format during transit and then decoded back to its original data by the receiving client.
There many other uses for Base64 encoding and decoding in PHP. For example, in a previous comment system we were using it encoded SVG data into Base64 which was then interpret by the web browser back into its original data.
Over the next couple of sections, we will show you how you can encode and decode using Base64 in PHP. In particular we will be touching on the base64_encode()
and base64_decode()
PHP functions.
Please note that Base64 should never be used for security. It is designed to be efficiently encoded and decoded on the fly and offers no protection.
The base64_encode() Function in PHP
In this section, we will be showing you how to encode a string in the Base64 format in PHP. To achieve, this you will be learning all about the base64_encode() function.
Definition of PHP’s base64_encode() Function
Let us show you how exactly the base64_encode() function is defined within PHP. Knowing how it is defined will make it easier for you to understand what sort of data you will be doing with.
This function is very straightforward as it only sports a singular parameter that expects a string.
Below you can see how base64_encode()
is defined. This definition shows the parameters expected data type and the functions return type.
base64_encode(string $string): string
Copy
This function only requires a single parameter. That parameter is the string you want to encode to the MIME Base64 standard.
PHP will return a Base64-encoded version of your string. You will note that this string will consume more data and be longer than the original data.
Due to the way Base64 works, this will consume roughly 33% more data than the original string, so be careful if you are dealing with a large source string.
Example of using base64_encode()
Now that we know the definition of the base64_encode() function in PHP, we can explore an example of how you would use this in your code.
We will keep this example relatively straightforward by passing a string straight into the function.
At the top of this example, we will create a variable called “$website
” and assign it the string “pimylifeup.com
“.
We then use PHP’s base64_encode()
function, passing in the “$website
” variable into it’s single parameter. The returned Base64 string will be output thanks to us using the echo statement.
<?php
$website = "pimylifeup.com";
echo base64_encode($website);
?>
Copy
Below, you can see how PHP encoded our “pimylifeup.com
” string in the Base64 format.
You will immediately notice that the resulting string is longer and ends with an equal sign. This is because the MIME-Base64 standard uses the equals sign for padding as the length must always be a multiple of three.
cGlteWxpZmV1cC5jb20=
The base64_decode() Function in PHP
Now that you know how to encode a string in the MIME-Base64 format in PHP let us explore how to decode the string.
PHP provides a function called base64_decode()
that will take an encoded string and decode it back into its original data.
Definition of PHP’s base64_decode() Function
Before we proceed, let us explore the definition of base64_decode()
within PHP. This definition will show you the various parameters and the return type of this function.
This function is what will take a Base64 string and convert it back to its original data.
Below you can see the definition of the function. This shows the two parameters alongside the data return type.
base64_decode(string $string, bool $strict = false): string|false
Copy
This function has two arguments that you can use. The first is required, and the second is optional.
$string
– This parameter is required and is where you will pass in your MIME Base64 encoded string.
If your string uses a different standard, you may experience issues using this function.$strict
– The strict parameter is optional and is set to “false
” by default.
By setting$strict
to “true
“, PHP will return “false
” if the Base64 string contains any character not available in the Base64 alphabet.
When set to “false
“, PHP will silently discard any invalid characters and attempt to decode the resulting string.
PHP’s base64_decode()
function will return “false
” on failure, or the decoded data. The decoded data may be binary, so be prepared to handle this within your code.
Examples of using base64_decode() in PHP
In this section, we will be showing you how to use the base64_decode()
function in PHP. We will be providing two different examples below.
The first example will show how the function is used without the optional parameter. The second example will show you how the strict option works.
Basic Usage of the base64_decode() Function
Our first example will explore the basic usage of the base64_decode()
function. So with this example, we will be ignoring the second parameter.
To start this script, we will define a PHP string called “$string
” and assign it a Base64 encoded string ("cGlteWxpZmV1cC5jb20="
). This string is the same one we encoded earlier in this guide.
We then pass our “$string
” variable straight into the base64_decode()
function. We then output the resulting string by using the echo statement in front of it.
<?php
$string = "cGlteWxpZmV1cC5jb20=";
echo base64_decode($string);
?>
Copy
After running the above example code, PHP will have decoded our Base64 string back into its original data. In our case, this will be the following text.
pimylifeup.com
Using the Strict Field in the base64_decode() Function
Now that we have seen the basic usage, let us try using the base64_decode()
function, but we will turn the strict mode on by setting the second parameter to “true
“.
We will adjust our encoded string by adding three invalid Base64 characters to show what this does. These are an exclamation mark (!
), a question mark (?
), and a hashtag (#
).
At the top of our script, we define our variable called “$base64_string
” and assign it the value “cGlteWxpZ?#mV1cC5jb20=
“.
We then will pass this into two separate function calls.
- The first will use PHP’s base64_decode() function but leave strict disabled.
This will show how PHP normally handles a Base64 string containing invalid characters. - We enable base64_decode()’s strict functionality for the second call by setting its second parameter to
true
.
Instead of attempting to strip out invalid characters, the function will fail and returnfalse
.
For both of these functions, we pass their output directly into PHP’s var_dump() function. This function will print both the value and data type that is returned.
<?php
$string = "cGlteWxpZ?#mV1cC5jb20=";
var_dump(base64_decode($string));
var_dump(base64_decode($string, true));
?>
Copy
Below you can see how PHP’s base64_decode()
function works when setting the strict parameter. When strict isn’t set or set to false, it will clean up the provided string and attempt to decode it.
However, it will simply fail and return false
when strict mode is enabled.
string(14) "pimylifeup.com"
bool(false)
Conclusion
In this tutorial, we showed you how PHP handles Base64 encoded strings. You can easily decode and encode any given string using two simple functions.
While originally designed to help send binary data in text-optimized protocols, this encoding still has its uses.
If you have any questions about the base64_encode()
and base64_decode()
functions, please comment below.
Be sure to check out our other PHP tutorials if you want to learn more. We also have many other programming guides if you want to learn a new language.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support