In this guide, I go through how and when to use require and require_once in PHP.
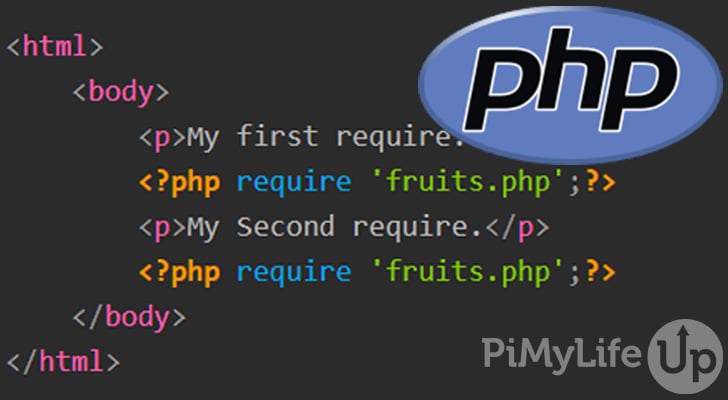
You will likely come across both require
and require_once
in PHP scripts. These two keywords are roughly the same but have a key difference that separates them.
When you use require
, PHP will include the specified file every time it is called. In comparison, require_once
will include the specified file once and ignore the file if it is mentioned again in the same script.
If the script is not compulsory for the script to run, you should consider using include or include_once instead. Using include will only throw a warning if the requested file does not exist. If you need the script to fail if the file does not exist, require
or require_once
are the perfect choice.
The files are requested based on the path specified in the statement. If there is no path, it will try using the include_path
specified in the PHP config file. Finally, PHP will check the script’s directory and the current working directory as the last attempt. If PHP cannot find the file in the above locations, it will fail and throw an error.
For require
or require_once
, PHP will trigger a fatal error if the specified file does not exist. The fatal error will stop the script execution preventing the code from progressing. Therefore using require is perfect if you include files essential to the script’s execution.
Where you use the require statement will determine the variable scope. For example, using require inside a function will have the file’s variables only available within the function.
When to use require
You will want to use require
whenever you may need to include a script multiple times throughout your code. For example, there may be cases where you need specific variables or output in different places.
If you want to avoid duplication issues and would rather not have the file included multiple times, you can use require_once. The require_once
statement will ensure that the file is included only once in the same script.
Our example below will show you how require behaves when you use it in two different places.
The file below is named index.php
and requires another file named fruits.php
.
<html>
<body>
<p>My first require.</p>
<?php require 'fruits.php';?>
<p>My Second require.</p>
<?php require 'fruits.php';?>
</body>
</html>
Below is the contents for the fruits.php
file which is required for the code above to run. The fruits.php
file should be in the same directory as the index.php
.
<ul>
<li>Apple</li>
<li>Banana</li>
<li>Orange</li>
</ul>
When you run the index.php
file you should get output that is exactly the same as our example below. You can see that our fruits.php
file outputs twice.
<html>
<body>
<p>My first require.</p>
<ul>
<li>Apple</li>
<li>Banana</li>
<li>Orange</li>
</ul>
<p>My Second require.</p>
<ul>
<li>Apple</li>
<li>Banana</li>
<li>Orange</li>
</ul>
</body>
</html>
When to use require_once
When you use require_once
, PHP will only ever include the requested file once in the entire script. Therefore, using require_once is hugely important if you want to avoid duplication issues, accidental variable reassignment, redefinition of functions, and more.
You should use require_once
when you do not need the file’s contents included multiple times. It is also helpful if you are working on a script and are unsure if the file has already been included and you do not want it included twice.
The code below is for our index.php
file where we use require_once
twice to include the fruits.php
file. This example will demonstrate how require_once
behaves when used within the same script.
<html>
<body>
<p>My first require_once.</p>
<?php require_once 'fruits.php';?>
<p>My Second require_once.</p>
<?php require_once 'fruits.php';?>
</body>
</html>
Below is our fruits.php
file and is located within the same directory as the index.php
file.
<ul>
<li>Apple</li>
<li>Banana</li>
<li>Orange</li>
</ul>
The output of the index.php
file should produce something similar to our example below. As you can see, the second require_once
did not output our fruits.php
file as we had already included it earlier.
<html>
<body>
<p>My first require_once.</p>
<ul>
<li>Apple</li>
<li>Banana</li>
<li>Orange</li>
</ul>
<p>My Second require_once.</p>
</body>
</html>
Using Require with a Missing File
When you use require with a file that does not exist it will result in a fatal error. In our example below we have a similar setup to the above examples but misspell our first file.
<html>
<body>
<p>My first require.</p>
<?php require 'fruitss.php';?>
<p>My Second require.</p>
<?php require 'fruits.php';?>
</body>
</html>
Our example below shows that PHP threw a fatal error and stopped the script from executing further. You can use this output to diagnose the issue further if required.
<html>
<body>
<p>My first require.</p>
<br />
<b>Warning</b>: require(fruitss.php): Failed to open stream: No such file or directory in <b>/home/development.local/public_html/index.php</b> on line <b>9</b><br />
<br />
<b>Fatal error</b>: Uncaught Error: Failed opening required 'fruitss.php' (include_path='.:/usr/share/php') in /home/development.local/public_html/index.php:9
Stack trace:
#0 {main}
thrown in <b>/home/development.local/public_html/index.php</b> on line <b>9</b><br />
Conclusion
I hope by now you have a decent idea of when to use require
or require_once
in your PHP code. Both are incredibly handy for including important files in your script. I also recommend looking at include or include_once if you want to include files that aren’t essential to your script.
We are always adding more PHP tutorials, so be sure to check them out if you want to learn more about coding in PHP.
If you think we can improve this guide, please do not hesitate to leave a comment at the bottom of this page.