In this tutorial, we will show you how you can redirect a user using the PHP programming language.
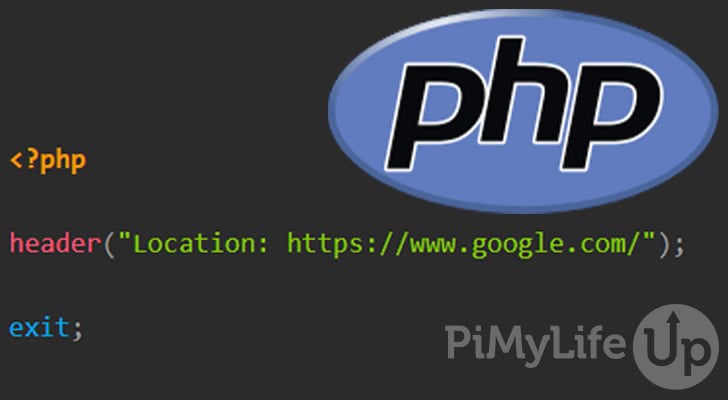
Using a PHP script to redirect users has been popular for many years. This guide shows you how you can achieve this using two different methods.
There are many reasons why you may want to redirect a user. For example, you may need to point them to a new version of an article that’s no longer available at a specific URL. You may also need to use a redirect if you are changing domains or you want to redirect users from HTTP to HTTPS.
Throughout this tutorial, we make use of the PHP header function. In addition, we have a full tutorial covering the header function that I recommend checking out if you need more information on how it works.
PHP Redirect Using the Header Function
There are two ways that you can redirect a user using PHP. In this tutorial, both methods that we show you will use the header function. We recommend using the location header as it is widely supported and provides a better user experience.
You must specify the header function before any output, as output will cause headers to lock up, resulting in an error.
Redirect using the Location Header
Using the location header is one of the most popular ways to redirect a user using PHP. There is no delay when using location, so the web browser will redirect the user as soon as they receive the header.
The location header is straightforward and should be specified like the example below. [URL] is the URL that you want to redirect the user to.
Location: [URL]
In the example below, we specify our header as a parameter in the PHP header function. Afterward, we terminate the PHP script by using the exit function.
<?php
header("Location: https://www.google.com/");
exit;
As soon as the user receives the location header, they are redirected to the specified URL along with the HTTP status code of 302 temporary redirect.

Changing the Status Code
By default, the status code of the redirect will be 302 temporary redirect. However, you can change this by specifying the status code you wish to be used. For example, you may want to use 301 (permanent redirect) or 303 (other).
Below is the syntax of the header function. We go into more detail about this function in our header tutorial, but to quickly recap, we will briefly touch on each of the parameters.
- Header is where we specify our header.
- Replace is where we specify TRUE or FALSE to replace any existing headers with the same name.
- http_response_code is the response code we wish to use.
header(header, replace, http_response_code)
The example below specifies the header that uses location and our destination URL. The second parameter is TRUE, as we wish to replace any existing header with the same name. Lastly, we want a permanent redirect, so we specify 301.
<?php
header("Location: https://www.google.com/", TRUE, 301);
exit;
As you can see below, our redirect used the status code we specified in the header function.

Redirect using the Refresh Header
If you wish to have a delay before redirecting the user, you can use the “refresh” header instead. The header “refresh” is not part of the HTTP standard but is supported by most major browsers.
The refresh method instructs the browser to refresh the web page after a certain time. We can change the page that will load when it refreshes by specifying a URL. Using this method, you can redirect a user after a certain amount of time.
You should write the header like our example below. [TIME] is the number of seconds you wish to wait before refreshing. [URL] is the URL of the location you wish to redirect the user towards.
refresh:[TIME];url=[URL]
In our example below, we wait for 5 seconds before redirecting to Google’s homepage. We use echo to output a string that the browser will display while the user is waiting to be redirected.
<?php
header( "refresh:5;url=https://www.google.com" );
echo 'You will be redirected in about 5 secs. If not, please click <a href="https://www.google.com">here</a>.';
When the user first loads the initial web page, the status code is 200 success. After the delay, the browser will redirect the user to the destination webpage.

Conclusion
I hope that you now have a good understanding of how you can redirect a user using the header function in PHP. Both methods have their use cases, but I recommend sticking to the location method for the best compatibility.
There is much more to learn about PHP and its functions, so I recommend checking out our wide range of PHP tutorials. For example, you may find it useful to learn more about headers or generating an MD5 hash.
If we can improve this tutorial, please do not hesitate to let us know by leaving a comment below.