In this tutorial, we will take you through the basics of the PHP statements echo and print. Both are widely in use, so it is important to understand how to use these statements.
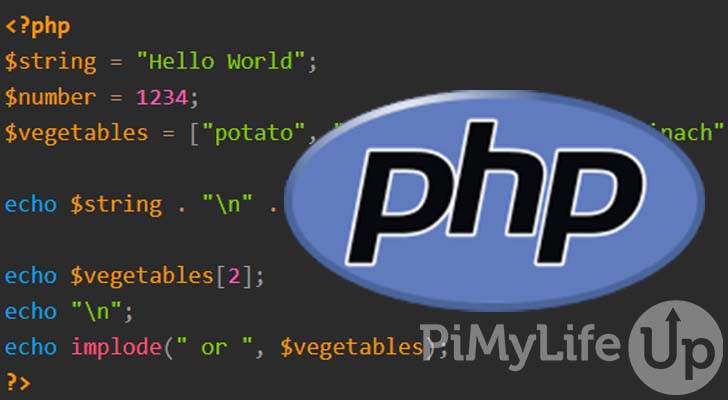
In PHP, you will use echo to output one or more expressions. It’s important to understand that echo is not a function but is a language construct. The arguments echo accepts are a list of expressions separated by commas. Unlike other language constructs, echo does not have a return value.
Like echo, print is also a language construct and not a function. You can only specify a single argument for print, and it will always return a value of 1.
You will be surprised to hear that print and echo are almost identical. The biggest differences are that print does not accept multiple arguments, returns a value, cannot be shorthanded, and is slower.
Table of Contents
Using the PHP echo Statement
The echo
statement is arguably used much more often than print in PHP scripts. Below we touch on several ways to use echo to achieve certain tasks. Of course, there is usually more that you can do, but we will touch on the basics.
Syntax of PHP echo
The syntax of echo is super simple, as you can see below. You will notice that it does not return any value, which is unusual for a language construct.
echo $expressions: void
You can also use echo with a shortcut syntax. Below is an example of how you can use the shortcut.
Hello <?="world"?>
We will go through a few examples of how you can use the PHP echo statement below.
Single String Argument
Below is an example of using a single string argument with echo. We include examples of it with and without parentheses. It is the most basic usage of echo and an easy way to display a string.
You will notice echo
will work with and without parentheses. However, it is recommended that you do not use parentheses as they can lead to confusion because parentheses are not part of the syntax but instead part of the expression. It may also lead users to think it’s a function when it is not.
<?php
echo "Hello World";
echo("Hello World");
?>
As you can see below, our example PHP script outputs “hello world” without any spacing. Everything is outputted on a single line because echo does not add new lines or spaces. You will need to add new lines into the expressions themselves. For example, echo "Hello World" , "\n"
or echo "Hello World" , "<br>"
.
Hello WorldHello World
Using HTML Elements
A string can contain HTML elements and it will be rendered correctly in a browser. For example, the code below will output a very basic web page.
<?php
echo "<h1>Basic Webpage.</h1>";
echo "<p>An example paragraph.</p>"
?>
In a browser, you should get a styled header followed by a short paragraph of text.
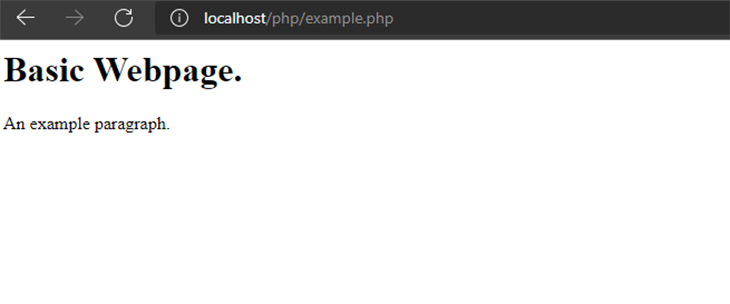
Multiple String Arguments
You can provide multiple arguments for an echo statement. Each argument is separated by a comma.
<?php
echo "Hello ", "World,", " I", " have ", "multiple", " arguments.";
echo "\n", "I am on a new line";
?>
The output of the above PHP code will contain the following text.
Hello World, I have multiple arguments.
I am on a new line
Concatenated Strings
As an alternative to specifying multiple arguments, you can simply concatenate the string instead. To concatenate in PHP, you simply use a .
between each string.
<?php
echo "Hello " . "World," . " I" . " was " . "multiple" . " strings.";
echo "\n" . "I am on a new line";
?>
The above code will output text similar to our previous example, where we specified separate arguments.
Hello World, I was multiple strings.
I am on a new line
Outputting Variables
Outputting variables using echo is very straightforward, but there are a few things you will need to be aware of before proceeding.
Some objects and data structures may not output correctly without additional functions or the correct pointer. For example, for an array data type, you may need to specify the element you wish to use, or you may want to use a function, such as implode.
Non-string types are automatically converted into string types. You may get a warning when this conversion occurs on data types such as arrays.
Below is an example of using a string, integer, and an array.
<?php
$string = "Hello World";
$number = 1234;
$vegetables = ["potato", "carrot", "parsnip", "spinach"];
echo $string . "\n" . $number . "\n";
echo $vegetables[2];
echo "\n";
echo implode(" or ", $vegetables);
?>
The above PHP code will result in the example output below.
Hello World
1234
parsnip
potato or carrot or parsnip or spinach
Non-String Types
Using echo will convert non-string types into a string type, even when strict_types=1
is declared. Below is a simple math problem, but you will see how it will complete the math problem and turn the result into a string.
<?php
echo 1 + 2;
?>
As you can see, the result is 3 and not “1 + 2“.
3
Using the PHP print Statement
Even though print is not as widely used as echo, you will likely still encounter many PHP scripts that use it. Below we go through a few topics that cover the basic usage of print.
Syntax of PHP print
The syntax of print is essentially the same as echo, as you can see below. There is one exception, print will return an integer.
print $expression: int
Next, we will touch on a few different examples of using print
in your PHP scripts.
Single Argument
One of the biggest differences between echo and print is that print will only support a single argument.
Much like echo, print supports parentheses. However, despite supporting parentheses, you will unlikely see them used much with print. Using parentheses can make print look like a regular function, but the parentheses are part of an expression, not the print syntax.
Below is an example of how you can use print in PHP.
<?php
print "Foo Bar";
print ("Foo Bar");
?>
The code above will result in the following output. It is all one line as we did not add any new lines, and print does not add new lines automatically.
Foo BarFoo Bar
Concatenated Strings
You can only specify a single argument using print, so you may want to concatenate a string instead. To concatenate a string in PHP, you simply use the .
between each string you wish to join.
<?php
print "Hello World," . " I am using print.";
print "\n" . "I am on a new line";
?>
The above code will result in the following output. As you can see, our output is split across two lines thanks to using the new line character \n
.
Hello World, I am using print.
I am on a new line
Outputting Variables
Using variables with print in PHP is the same as with echo. For example, objects and data structures such as arrays need to be pointing to the correct element you want to use; otherwise, it may result in an error or incorrect data.
Any non-string types are automatically converted to strings which may result in an error or incorrect data being displayed.
Below is an example of how to display variables such as a string, integer, and an array.
<?php
$string = "Hello World";
$number = 5678;
$fruit = ["apple", "banana", "grapes", "pear"];
print $string . "\n" . $number . "\n";
print $fruit[2];
print "\n";
print implode(" or ", $fruit);
?>
Below is the output from the example code above.
Hello World
5678
grapes
apple or banana or grapes or pear
Non-String Types
When you use print
to output data, any non-string types will be automatically converted to a string type. The conversion will occur even if strict_types=1
is declared.
Below is an example of how print
will treat a simple math problem. PHP will complete the math equation before being converted into a string.
<?php
print 11 + 42;
?>
The output of the above PHP code will display the following answer.
53
Conclusion
I hope that I have covered all the PHP echo and print statements basics. Both of these should come in handy when writing your next PHP script.
If you want to learn more about PHP and the huge number of tasks you can achieve with it, be sure to check out the ever-growing list of PHP tutorials. Also, do not be afraid to let us know if you have a topic that you would like us to cover.
Please let us know if there is an error or not enough information on the topics we have covered by leaving a comment below.