In this guide, I go through how to use a switch statement in PHP.
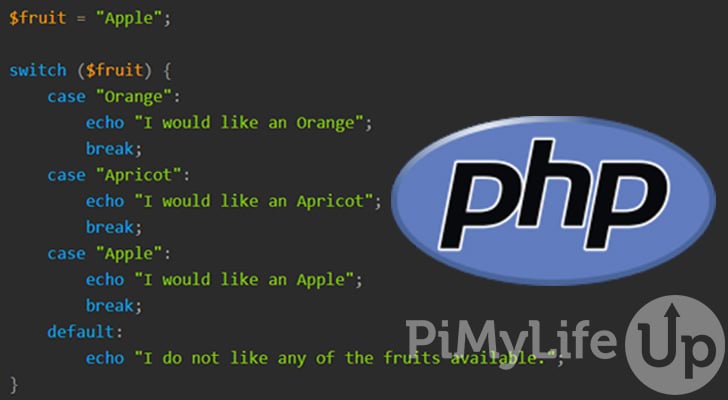
Using a switch statement is an excellent alternative to using a series of if and elseif statements. We explain in this tutorial why you might want to pick one over the other.
There are quite a few unique features of a switch statement that you will need to know if you plan to use them. For example, switch statements execute line by line. If you do not use a break statement, a switch will execute all the code after a match until it reaches the end of the switch. We will explain this in more detail further on.
You can nest switch statements inside each other, but it is not recommended as it can make code very hard to read and understand.
This tutorial will touch on how to write a switch statement in PHP and cover some of the basics that you need to know. We also touch on comparing switch statements to if-else statements.
Table of Contents
switch Statement Syntax
The syntax of a switch statement is pretty straightforward, but you will need to know a few things about how they operate.
A switch accepts an expression that will only be evaluated once. In most cases, the expression is a variable that PHP will compare to the case statements within the switch structure. Once a match is found, the code block associated with the case statement will be executed.
A switch can have many case statements in PHP, but it is good practice to keep them to a minimum as lots of case statements can make the code hard to read.
The break statements are optional, but without them, PHP will execute all the code after a match until it hits a break statement or the end of the switch block.
You can specify a default statement at the end of a switch that will only execute when the expression does not match any case statements. The default statement is optional and can be left out of the switch if you do not require it.
After each case statement, you can use a semicolon instead of a regular colon. However, we recommend using a colon as it helps make the code more readable.
switch (i) {
case match1:
//code to be executed if match;
break;
case match2:
//code to be executed if match;
break;
case match3:
//code to be executed if match;
break;
...
default:
code to be executed if i does not match with any case;
}
Alternatively, you can write a switch statement using switch(i):
and end it with endswitch
. The example below demonstrates the alternative syntax for a switch in PHP.
switch (i) :
case match1:
//code to be executed if match;
break;
case match2:
//code to be executed if match;
break;
case match3:
//code to be executed if match;
break;
...
default:
code to be executed if i does not match with any case;
endswitch;
switch vs. if-else
This section will compare the differences between a switch and a series of if and elseif statements.
A PHP switch statement is evaluated only once, whereas a series of if and elseif statements are evaluated every time they are specified. This slight difference can impact the expected behavior of your code, so keep this in mind. In terms of performance, there is very little performance difference between using a switch and a series of if statements.
The most significant benefit of using a switch is readability. A switch can be much easier to read and understand than a series of if-else and elseif statements. However, readability can still come down to personal preference.
Knowing when to use a switch statement over a series of if-else and elseif statements mostly comes down to preference. Over time you will learn when to use one over the other. Typically for complicated conditions, I use an if-else series. For simple comparisons, I use a switch.
Below is a switch and a series of if-else and elseif statements. Both will result in the same output.
<?php
$fruit = "Apple";
if ($fruit == "Orange") {
echo "I would like an Orange";
} elseif ($fruit == "Apricot") {
echo "I would like an Apricot";
} elseif ($fruit == "Apple") {
echo "I would like an Apple";
}
else {
echo "I do not like any of the fruits available.";
}
switch ($fruit) {
case "Orange":
echo "I would like an Orange";
break;
case "Apricot":
echo "I would like an Apricot";
break;
case "Apple":
echo "I would like an Apple";
break;
default:
echo "I do not like any of the fruits available.";
}
?>
How to Use a switch Statement in PHP
It is up to the developer when to use a switch statement in their PHP coding. Typically, it is good to use a switch when you have multiple comparisons of the same expression with different values. A well-laid-out switch is much easier to understand than a complicated if-else and elseif structure.
Below we will go through several different ways to write a switch statement.
Writing a Basic switch Statement
In this example, we create a basic switch statement that compares a string against several different case statements within the switch. I will explain the process of the code below.
We first create a variable named $fruit
to store our string “Apple“. Next is our switch, where we specify our variable as the expression. The expression will be evaluated once.
PHP will now compare the value of the expression against each value in our case statements. Our string matches the third case statement containing the “Apple” string.
PHP will execute the block of code under our matching case statement. The code block contains an echo statement and a break statement. The echo statement will output the string, and the break statement will force an exit from the switch.
<?php
$fruit = "Apple";
switch ($fruit) {
case "Orange":
echo "I would like an Orange";
break;
case "Apricot":
echo "I would like an Apricot";
break;
case "Apple":
echo "I would like an Apple";
break;
default:
echo "I do not like any of the fruits available.";
}
?>
The output below shows that our $fruit
variable matched the “Apple” case statement.
I would like an Apple
Default Statement
In our example below, we have a switch that uses integers rather than strings. The variable ($num
) we will be using in the expression has the value of 0
and will not match up with any of our case statements within the switch. As a result, the default code block will run.
<?php
$num = 0;
switch ($num) {
case 1:
echo "The number is 1.";
break;
case 4:
echo "The number is 4.";
break;
case 2:
echo "The number is 2.";
break;
default:
echo "I do not have a matching number.";
}
?>
Since our $num
variable does not match any case statements, the default code block will run, and you will get the output below. If you do not have a default statement, nothing will be outputted.
I do not have a matching number.
Combining Case Statements
If you have the same result for multiple case statements, you can combine them and reduce code repetition. In our example below, we have the same output for three different cases, lemon, line, and orange.
<?php
$fruit = "Lime";
switch ($fruit) {
case "Lemon":
case "Lime":
case "Orange":
echo "I like citrus.";
break;
case "Apricot":
echo "I like Apricot";
break;
case "Apple":
echo "I like Apple";
break;
default:
echo "I do not like any of the fruits available.";
}
?>
Our code above resulted in the following output because our variable matches one of the first three case statements that share the same code block. We have an echo inside the code block that prints out the following text.
I like citrus.
Using break Statements
You can use break statements to control how the switch statement handles matches. For example, if a break does not exist, the switch will execute every code block from the first match to the end.
In the following example, our string will match the first case statement. However, there are no break statements until our case statement checks for “Orange“. Since there are no break statements, PHP will execute the first three echo statements.
<?php
$fruit = "Lemon";
switch ($fruit) {
case "Lemon":
echo "I like Lemon.";
case "Lime":
echo "I like Lime.";
case "Orange":
echo "I like Orange.";
break;
case "Apricot":
echo "I like Apricot";
break;
case "Apple":
echo "I like Apple";
break;
default:
echo "I do not like any of the fruits available.";
}
?>
The output below demonstrates how the switch handles case statement code blocks with no break statement. It is very important to keep this in mind when writing your switch statements in PHP.
I like Lemon.
I like Lime.
I like Orange.
Nesting Switch Statements
The last topic I want to touch on is nesting switch statements. You can nest switch statements, but it is not recommended as it can get messy and hard to read fast.
Below is an example of two nested switch statements in PHP.
<?php
$fruit = "Lemon";
$vegetable = "Carrot";
switch ($fruit) {
case "Lemon":
echo "I like Lemon and ";
switch ($vegetable) {
case "Broccoli":
echo "I like Broccoli.";
break;
case "Lettuce":
echo "I like Lettuce.";
break;
case "Carrot":
echo "I like Carrot.";
break;
default:
echo "I do not like any of the vegetables available.";
}
break;
case "Lime":
echo "I like Lime and ";
switch ($vegetable) {
case "Potato":
echo "I like Potato.";
break;
case "Cauliflower":
echo "I like Cauliflower.";
break;
case "Parsnip":
echo "I like Parsnip.";
break;
default:
echo "I do not like any of the vegetables available.";
}
break;
default:
echo "I do not like any of the fruits available.";
}
?>
The above code has a variable ($fruit
) that matches with the first case statement (“Lemon“) in our outer switch. It also has a second variable ($vegetable
) that matches with the last case statement (“Carrot“) in our first nested switch. The resulting output is below.
I like Lemon and I like Carrot.
Conclusion
I hope this guide has shown you all the basics of using a switch statement in PHP. It is an extremally helpful way to control code execution and help make code readable for other developers.
If you want to learn more about programming in PHP, I recommend checking out our ever-growing range of PHP tutorials. We cover topics such as for loops, while loops, if-else, and more. The tutorials are perfect for both beginner and advanced developers.
If you notice we can improve this tutorial, please do not hesitate to let us know by leaving a comment below.