This tutorial will teach you how to use the empty() function in PHP.
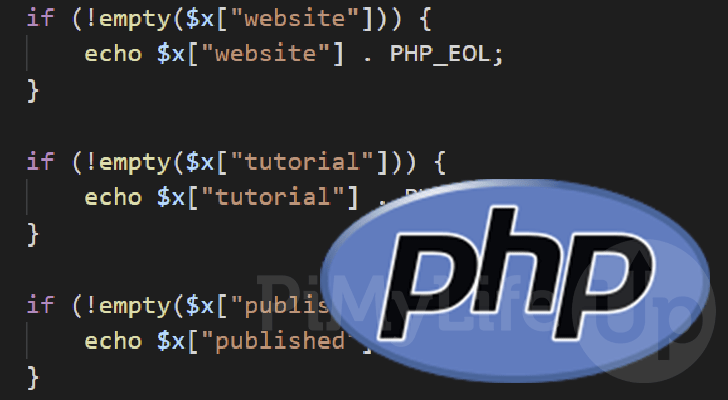
In PHP the empty() function allows you to check whether a variable is empty / has no value. This function behaves slightly differently from PHP’s isset() function which is used to check if a variable is set.
The empty() function will consider your value empty if the variable doesn’t exist or is assigned a value that is zero, false, or considered “empty” by PHP.
For example, if your variable is defined as a string but doesn’t contain any actual characters such as ""
then it will be considered “empty” by this function.
This function is useful for ensuring that you actually have a usable value in your variable.
Syntax of the empty() Function in PHP
This first section will explore the syntax of the empty() function within PHP. The syntax tells you a few things about the function, such as what data it expects and what it will return.
From the syntax you can see that the empty() function takes a single parameter. Therefore, this parameter will be the variable you want to check if it is “empty” or not.
Also, this same syntax shows that PHP will return a bool value (true
or false
).
empty(mixed $var): bool
The empty() function’s single parameter allows you to pass in a variable you want to be checked. This variable can be of any data type and does not even need to exist.
If the variable does not exist or has a false value, the empty() function will return true
.
Alternatively, if the variable exists and has a valid value, the function will return false
.
Truth Table for the empty() Function
With the table below, you can easily see situations in which PHP’s empty() function will return true
or false
.
It is much more sensitive than the isset() function as it considers the variable’s value and not just whether it is set.
Expression | empty() Return |
---|---|
$x = “”; | true |
$x = []; | true |
$x = true; | false |
$x = null; | true |
$x is undefined | true |
Read on further to see examples of the empty() function used within PHP.
Using PHP’s empty() Function in your Code
This section will show you a couple of examples of how you can use the empty() function within PHP.
It is a reasonably simplistic function but one you will find useful in a wide variety of use cases.
Basic Usage of the empty() Function
Let us start with a simple usage of the empty() function within PHP.
With this example, we will create two variables. The first variable called “$x
” will be assigned the value 0
, which is an empty value.
The second variable will be called “$y
“, and we will give it the PHP string value "PiMyLifeUp"
.
For both variables we will use PHP’s empty() function to check whether they are empty. Finally the result is dumped to the output thanks to us using the “var_dump()
” function.
The advantage of using the var_dump() function is that it will tell us both the return variable and the data type returned.
<?php
$x = 0;
var_dump(empty($x));
$y = "PiMyLifeUp";
var_dump(empty($y));
?>
Below you can see how the empty() function worked on our two variables. You can see the “$x
” variable was considered “empty”, and the “$y
” variable was not.
bool(true)
bool(false)
Using empty() on an Array Element
Another great use of the empty() function in PHP is to check array elements in an associative array.
This is especially useful as you can check whether an array element exists and if it contains a non-empty value.
With this example, we start by creating an associative array named “$x
” and assigning it various values. Our “published
” and “views
” keys will both contain empty values.
Next, we will use the empty() function within multiple if statements. We check whether a key in our array is not empty with each conditional statement.
By using the logical not operator (!
), we are inverting the result of the function, meaning the if statement will only trigger if the value is not empty.
If the value is set, we use PHP’s echo statement to print the value to the screen.
<?php
$x = [
"website" => "pimylifeup",
"tutorial" => "empty() Function in PHP",
"published" => false,
"views" => 0
];
if (!empty($x["website"])) {
echo $x["website"] . PHP_EOL;
}
if (!empty($x["tutorial"])) {
echo $x["tutorial"] . PHP_EOL;
}
if (!empty($x["published"])) {
echo $x["published"] . PHP_EOL;
}
if (!empty($x["views"])) {
echo $x["views"] . PHP_EOL;
}
?>
Below is the output you should get from the above example. Since both our “published
” and “views
” keys are considered empty, they will not be printed,
pimylifeup
empty() Function in PHP
Using empty() on every Empty Value
This final example is simply here to show the cases in which the empty() function will return true
in PHP.
So let us start this example by defining several variables. From this example, you will notice one edge case. The possible empty values are added into an “empty_values
” array.
We use a foreach loop to go over each value in this array. Next, we use var_dump()
to print the value and its type. We then use echo to print a statement based on the result of the ternary operator.
If the value is “empty()”, the text “is empty
” will be printed. Otherwise, the text “is not empty
” will be printed.
Finally, we use the PHP_EOL
constant to add a couple of new lines to the bottom of each result.
<?php
$empty_values = [
0, // Empty Integer
0.0, // Empty Float
"", // Empty String
"0", // 0 As string is empty
NULL, // Empty Value
[], // Empty Array
false, // False Value
];
foreach ($empty_values as $value) {
var_dump($value);
echo empty($value) ? "\tis Empty" : "\tis Not Empty";
echo PHP_EOL . PHP_EOL;
}
After running the above example, you should see the following result output to the page. page. There is one peculiar result here: the string "0"
is also considered empty.
int(0)
is Empty
float(0)
is Empty
string(0) ""
is Empty
string(1) "0"
is Empty
NULL
is Empty
array(0) {
}
is Empty
bool(false)
is Empty
PHP considers the string "0"
to be empty because when type juggling occurs, it turns the value into 0, which is a false value.
If you need PHP not to consider that value empty, you will need to add an additional check to ensure the value is not equal to the string "0"
, as shown below.
<?php
$x = "0";
if (empty($x) && $x !== "0") {
//Run some code here
}
?>
Conclusion
This guide showed you how to use the empty() function in PHP.
This function is simple to use but is incredibly useful. It allows you to ensure that the variable you are dealing with both exists and has a valid value.
Please comment below if you have any issues using the empty() function.
We also have a wealth of other PHP tutorials if you want to learn more about this language. Be sure also to check out our many other programming tutorials.