In this guide, we will touch on the different data types that you can use in the PHP programming language.
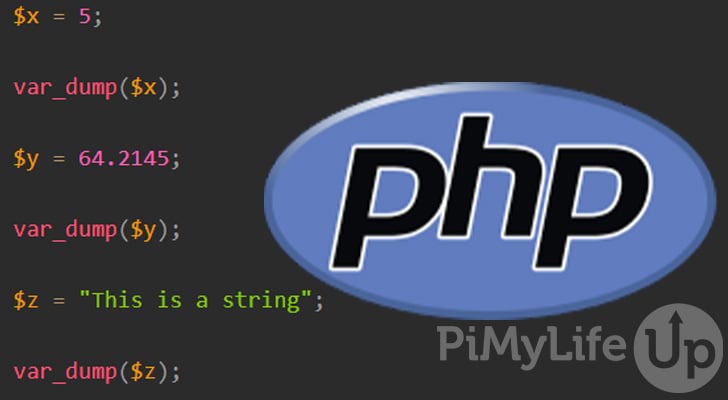
It is important to understand the PHP data types available to you as you will likely need to use most of them at least once. Most data types are similar to most other programming languages, but you will find some unique characteristics in PHP.
Below we will touch on different data types that PHP supports such as integers, floats, strings, Booleans, arrays, objects, resources, and NULL. Please let us know if we have missed anything or if a particular topic requires more explanation.
This guide assumes you are using the latest version of PHP. There may be some differences in functionality and definitions if you are using an older version of PHP.
Table of Contents
PHP Scalar Types
A scalar type refers to values that are a single item rather than a collection or composite. In PHP, a scalar variable can contain an int, float, string, or bool.
Integer
Integers are whole numbers and can be anywhere between -2,147,483,648 and 2,147,483,647. Since an integer is a whole number, you cannot use a decimal point.
You can specify a PHP integer in hexadecimal (base 16), decimal (base 10), octal (base 8), or binary (base 2) notation.
Below is an example of assigning an integer to a variable in PHP.
<?php
$x = 5;
var_dump($x);
The output of the above script is below.
int(5)
Float
A float is also known as a floating-point number and is a number that contains a decimal point. A fractional number will be incredibly useful when performing math equations where a whole integer is not accurate enough.
The float type includes the double type. You may come across references to double in older tutorials and manuals.
Below is an example of assigning a float to a variable in PHP.
<?php
$y = 64.2145;
var_dump($y);
Below is the output of var_dump
.
float(64.2145)
String
A PHP string is a sequence of characters that you can store within a single variable. The maximum length of a string is 2147483647 bytes or 2 GB.
You can specify a string by enclosing text within single or double quotes.
Below is an example of assigning a string to a variable within PHP.
<?php
$x = "This is a string";
var_dump($x);
Below is the output from the above script.
string(16) "This is a string"
Boolean
A Boolean has two possible values, TRUE
or FALSE
. You will often use a Boolean type within a conditional statement. The code below demonstrates using Booleans within the PHP programming language.
<?php
$x = true;
$y = false;
if($x == true){
echo "The x value is true";
}
Below is the output from the above script.
The x value is true
PHP Compound Types
A compound data type can hold multiple values, such as integers, floats, strings, and more. The two examples of a compound type in PHP are arrays and objects.
Array
An array in PHP is very similar to an array in other programming languages. For example, you can store multiple values in an array.
Arrays in PHP are ordered maps where each value is tied to a key. If you do not specify a key, a key is set automatically starting from 0 and incrementing by 1 for each new value.
The example below creates an array and stores it in the $fruits
variable. Then, we use the var_dump
function to print the data type and all the values within the array.
<?php
$fruits = ["apple", "banana", "watermelon";
var_dump($fruits);
The output from the above script is below.
array(3) { [0]=> string(5) "apple" [1]=> string(6) "banana" [2]=> string(10) "watermelon" }
Object
In object-orientated programming, classes and objects are two main concepts you will need to understand. A class is a template for an object and can contain constants, properties, and methods.
When you assign a class to a variable using the new keyword, it will create an object. An object is an instance of the class. The PHP object will inherit all the class’s properties, methods, and behaviors.
Each time you create a new object, it is independent of other objects created from the same class.
Below is a very basic example of a class and object. First, we assign our class to the $obj
variable by using the new keyword, which will create an object.
<?php
class exampleClass
{
function write_example()
{
echo "Example";
}
}
$obj = new exampleClass;
var_dump($obj);
Below will confirm that our variable contains an object.
object(foo)#1 (0) { }
PHP Special Types
There are two data types in PHP that do not fit into the scalar or compound category and are thus referred to as special types. The two special data types in PHP are resource and NULL.
Resource
The PHP resource special type is used to hold references to resources and functions that are external to the PHP script. For example, connections to a database or references to a file will be considered a resource type.
The example below demonstrates a resource type by using the fopen
function.
<?php
$file = fopen("example.txt", "r");
var_dump($file);
As you can see below, our file reference is the special resource type.
resource(2) of type (stream)
NULL
The NULL special data type is used whenever a variable has no value assigned to it. A user can assign a variable the value of NULL. Otherwise, it is automatically assigned when a variable is created with no value.
This special data type can only have one value, NULL.
Below is an example of the NULL value in use.
<?php
$x = NULL;
$y;
var_dump($x);
var_dump($y);
The script above should print out NULL values for both of the variables.
NULL NULL
Conclusion
I hope by now you have a decent understanding of the different data types that are available within PHP. I highly recommend reading more information on each data type, so you know how to use them correctly.
We have plenty more tutorials on the PHP programming language that covers a wide range of topics. If you are new to PHP, I recommend checking out some basics such as variables, constants, or if-else statements.
If we can improve this tutorial, please do not hesitate to let us know by leaving a comment below.