In this tutorial, we will be showing you how you can define and use constants in PHP.
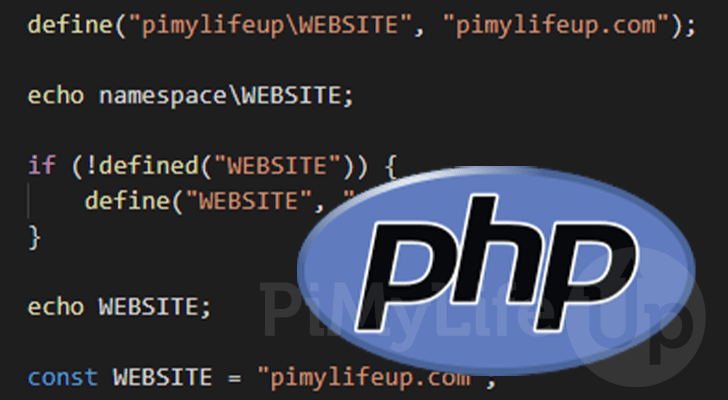
In PHP, a constant is an identifier that holds a specified value. The value stored within a constant can only be defined at runtime.
You can’t change the value stored within a constant after it has been defined. It is helpful for values you want to access throughout your PHP script and don’t want or need their value to be changed.
For example, you can use a constant within PHP to store your SQL server’s login details for access during runtime.
There are two ways that you can define a constant within PHP.
- The first method is to utilize the
define()
function. These constants are handled during runtime. - The second method is to use the “
const
” keyword. Constants defined using this are set during compile time before your code is ran.
We will explore these methods and explain some fundamental differences between them within this guide.
When naming a constant within your PHP code, you should always use uppercase letters. Additionally, a constant still follows standard variable naming rules.
A constant must start with a letter or underscore, followed by letters, numbers, or underscores. However, it would help to avoid writing constants that start with and end with two underscores. PHP’s “magic constants” typically use these.
When referencing a constant within your code, you do not use the dollar sign ($
) before its name. So, for example, you would use “FTP_DETAILS
” and not “$FTP_DETAILS
“.
Lastly, by default, constants are automatically accessible throughout your entire script, which means that PHP can access them throughout your code.
Defining Constants using PHP’s define() Function
The define()
function is one of the two ways to define a variable as being a constant within the PHP language. It is not as user-readable as the “const
” keyword but still has some advantages.
When constants are defined using this function, they are not processed until run time. Meaning you can use PHP’s conditional statements to dictate whether the constant is defined or not.
The syntax for using the define function is straightforward, with it only having two parameters that you need to worry about.
define(NAME, VALUE)
Copy
The first parameter (NAME
) is where you will set the name for your constant.
Remember that when setting the name here, you should use uppercase letters. So, for example, a valid name for a constant in PHP would be “FTP_DETAILS
“.
The second (VALUE
) parameter is where you will set the value you want to be assigned to your constant.
Basic Declaration of a Constant in PHP using define()
Let us show you how simple it is to use the define()
function to declare a constant within PHP.
We will use the name “WEBSITE
” for this constant and set the value to a string containing “pimylifeup.com
“.
You can see that when we used the define function, we passed our variable name into the first parameter, then the value we wanted to set to the second parameter.
We then utilize PHP’s inbuilt echo function to output the value that we set our constant.
<?php
define("WEBSITE", "pimylifeup.com");
echo WEBSITE;
?>
Copy
Remember that you must avoid using the dollar sign ($
) in front of its name to utilize a constant. A constant is referenced purely using its name. So for example, you would use “CONSTANT
“, instead of “$CONSTANT
“.
Constants Declared with define() are Global
Constants that are declared using the define()
function become globally available to the current PHP script. You can even use it within functions and classes.
To showcase this, let us write a simple script. This script will contain a function called foo()
that will output the value of our constant when called.
With this example, you can see how the constant can be still utilized within a function.
<?php
define("WEBSITE", "pimylifeup.com");
function foo() {
echo WEBSITE;
}
foo();
?>
Copy
Likewise, if we were to write a simple class called Bar, you can see how you can still use a PHP constant within it.
We will use the same “define()
” function as our previous example. This time we will make a simple class called “Bar
“. In this classes construction method, we will echo the value of the “WEBSITE
” constant.
<?php
define("WEBSITE", "pimylifeup.com");
class Bar
{
public function __construct() {
echo WEBSITE;
}
}
new Bar();
?>
Copy
Declaring Constants in PHP within a Different Namespace
Declaring a constant when using the define function using a different namespace isn’t the clearest code. By default, the define function will also default to the global namespace.
When declaring a constant within a particular namespace, you need to include the namespace as part of its name.
For example, if you want the constant to be a part of a namespace called “pimylifeup
” you would use “pimylifeup\NAME
“.
The example below shows you how to declare a constant using the define function under a specific namespace.
We then print the constant we created under the current namespace.
<?php
namespace pimylifeup;
define("pimylifeup\WEBSITE", "pimylifeup.com");
echo namespace\WEBSITE;
?>
Copy
Unless needed, you should use the “const
” keyword. It will automatically be declared under the current namespace.
Conditionally Declaring Constants in PHP using the Define() Function
The key advantage of using the define() function over the “const” keyword to declare a constant is when they are processed.
A constant isn’t processed until runtime using the define()
function, meaning it can be conditionally set.
Conversely, constants defined using the “const
” keyword are processed at compile time. Meaning they are defined before any code runs.
With the following example, we use the “defined()
” function to see if the constant has already been defined. If it hasn’t, we declare it.
This snippet is useful if the constant could of possibly been defined elsewhere in the code.
<?php
if (!defined("WEBSITE")) {
define("WEBSITE", "pimylifeup.com");
}
echo WEBSITE;
?>
Copy
You can Pass Arrays into the define() Function
Starting with PHP 7, it is possible to use an array as the value of a constant when using the define function.
Before the release of 7.0, you would be required to use the const keyword whenever you wanted to define an array as a constant.
All you need to do is set the value to an array. We will be writing a simple example, declaring the “FOOD
” constant with a simple array.
You access elements of a constant array just like you would with a normal array within PHP. With the example below, we will print out the third element of the array.
<?php
define("FOOD", [
'Apple',
'Carrot',
'Raspberry Pie'
];
echo FOOD[2];
?>
Copy
Using the const Keyword in PHP to define a Constant
The second way of declaring a constant in PHP is to use the const keyword. This is considered more readable than using the “define()
” function and has some behavioral differences.
When using the const keyword, a constant is set during compilation time, not run time. Unfortunately, this means you cannot use the const keyword within conditional statements.
Like using the define() function, you should write the constant name in uppercase letters or numbers.
The basic syntax for using the const keyword to define a constant in PHP is as we have shown it below.
const NAME = value;
Copy
Basic Usage of the const Keyword to Declare Constants
For this example, we will declare a new constant using PHP’s const keyword. We will name this constant “WEBSITE
” and assign it the value “pimylifeup.com
“.
Once declared, we will use the echo function to print the value of the constant to the screen.
<?php
const WEBSITE = "pimylifeup.com";
echo WEBSITE;
?>
Copy
Using const to Declare Global Constants in PHP
You can use the const keyword to declare constants globally in PHP. All you need to do is ensure that it is declared outside of a namespace or class.
With the example below, you can see that our “WEBSITE
” constant is still accessible from within the “bar()
” function.
When the function is called, it will print the value of our global constant.
<?php
const WEBSITE = "pimylifeup.com";
function bar() {
echo WEBSITE;
}
bar();
?>
Copy
You can Declare Constants within a PHP Class using the const Keyword
Unlike the define function, you can use the const keyword to declare a constant within a class.
Unless you specify it differently, the constant will be publicly accessible.
With this example, we will show you various ways to declare and access a constant within a class in PHP.
When you declare your constant within the class, you can access it by using “self::
” followed by the constant name.
Outside of the class you can reference the constant by accessing the class statically (E.G. “foo::
“) or by creating a function that outputs the value of the constant.
<?php
class foo
{
const WEBSITE = "pimylifeup.com";
function print_constant() {
echo self::WEBSITE;
}
}
echo foo::WEBSITE;
$foobar = new foo();
$foobar->print_constant();
?>
Copy
Constants Declared with the const Keyword Adopt the Current Namespace
Constants that are declared within PHP using the const keyword will adopt the currently set namespace.
This is much cleaner to use than the define function, where you must include the namespace in its declaration.
The example below will show you how easy it is to declare a constant within a namespace using the const keyword. We then print the declared value.
<?php
namespace helloworld;
const WEBSITE = "pimylifeup.com";
echo namespace\WEBSITE;
?>
Copy
Conclusion
Throughout this guide, we have shown you the various ways to declare a constant in PHP.
A constant is a variable that’s value doesn’t and cannot be changed after its initial declaration. PHP’s “define()
” function allows you to declare constants that are created during runtime.
The inbuilt const keyword allows you to declare constants that are created at compilation time. There is a small performance boost by using this over the define function.
Please comment below if you have any questions about dealing with constants in PHP.
To learn more about PHP and its various features, check out our many other PHP guides. We also have tutorials that cover other programming languages as well.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support