In this guide, I go through how and when to use include and include_once in PHP.
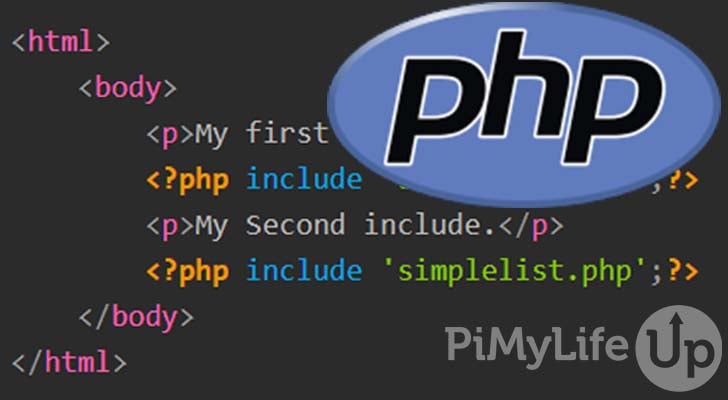
There isn’t a massive difference between include
and include_once
. The main difference is that include will add the specified file every time it is called. However, include_once
will only ever include the file once and never again.
The files are included based on the path given in the statement. If no path is specified, then it will try using the include_path
specified in PHP’s config file. Lastly, PHP will check the script’s directory and the current working directory before throwing a warning.
When using include
or include_once
, PHP will throw a warning if the specified file does not exist, but it will not stop the script execution. So, use include
only when the specified file is not essential for your program to function correctly.
It’s important to remember that when you include a file, it will inherit the variable scope of where it is called. So, for example, if you include a file inside a function, its variables will only be available inside the function where you included the file.
You should use require if you need the script to throw a fatal error and stop executing whenever the specified file does not exist. So, both include and require have unique use cases, so think carefully before using them in your code.
When to use include
You will want to use include
in situations where you need to include a file multiple times throughout a script. For example, you have some output that you want to be used in several places but do not want to repeat the same code constantly.
If you do not want the file included multiple times, you will need to use include_once
instead. I also recommend using include_once
if you are including code that might cause code duplication problems.
To demonstrate how you can include the same file multiple times using include we create a PHP file named index.php
.
Inside the PHP script we have the code below. We call a PHP file named simplelist.php
twice using the include
statement.
<html>
<body>
<p>My first include.</p>
<?php include 'simplelist.php';?>
<p>My Second include.</p>
<?php include 'simplelist.php';?>
</body>
</html>
Copy
The contents of the simplelist.php
file is below. It is a simple HTML unordered list.
The simplelist.php
file is in the same directory as the index.php
file.
<ul>
<li>1</li>
<li>2</li>
<li>3</li>
</ul>
Copy
The output below is the HTML output of the PHP files above. You can see our PHP file containing the HTML list was included twice.
<html>
<body>
<p>My first include.</p>
<ul>
<li>1</li>
<li>2</li>
<li>3</li>
</ul>
<p>My Second include.</p>
<ul>
<li>1</li>
<li>2</li>
<li>3</li>
</ul>
</body>
</html>
Copy
When to use include_once
As the name implies, PHP will not include a file more than once when you use the include_once
statement. So, it is very useful for avoiding duplication problems, redefinitions of functions, or even variables having their value reassigned.
I recommend using include_once
when you do not want a file included multiple times. It is also ideal if you are unsure if the file is already included and you do not want to include it again.
In our example we have a file named index.php
with the contents below.
We call the simplelist.php
file twice using include_once
to demonstrate how the statement works in PHP.
<html>
<body>
<p>My first include_once.</p>
<?php include_once 'simplelist.php';?>
<p>My Second include_once.</p>
<?php include_once 'simplelist.php';?>
</body>
</html>
Copy
The contents of the simplelist.php
file is below. It is a simple HTML unordered list.
<ul>
<li>1</li>
<li>2</li>
<li>3</li>
</ul>
Copy
Below is the raw HTML output of our two PHP files above. The code will render correctly in a web browser.
As you can see in the output, our file was only included once.
<html>
<body>
<p>My first include_once.</p>
<ul>
<li>1</li>
<li>2</li>
<li>3</li>
</ul>
<p>My Second include_once.</p>
</body>
</html>
Copy
Conclusion
Using include
or include_once
in your PHP code is pretty straightforward and should be easy to understand. However, if you think we can improve this guide, please do not hesitate to leave a comment at the bottom of this page.
If you are new to PHP and wish to learn more, I highly recommend checking out some of our PHP tutorials. We cover a huge range of topics and are always adding more.
is the include once per session or once per page load?
Hi Myles,
With PHP an include is only for that specific request.
Kind regards,
Emmet