In this tutorial, you will learn how to use the basename() function in PHP.
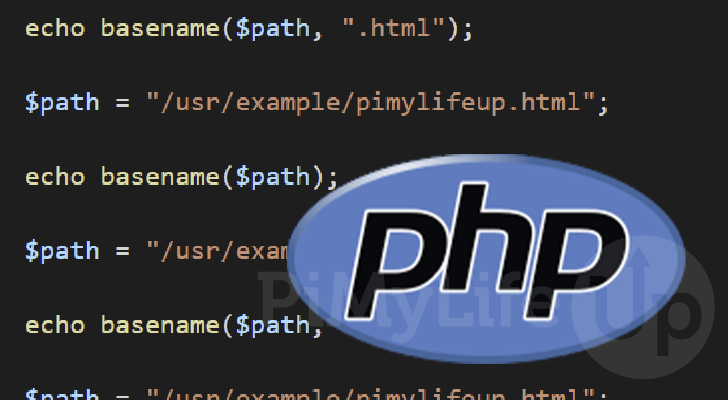
PHP’s basename()
function allows you to retrieve the trailing name component from a passed-in string. The returned trailing component is what is called the “base name”.
The string you pass into this function must contain either a path to a file or a directory.
For example, if you had a string containing the path “/etc/example/pimylifeup.txt
“, this function will return “pimylifeup.txt
“. Alternatively, if you had the path “/etc/example/
“, the base name would be “example”.
PHP’s basename() function is straightforward to utilize, as you will see in the following sections.
Definition of PHP’s basename() Function
Before we show some examples of how you utilize the basename() function within PHP, let us explore how it is defined.
The basename() function accepts two parameters, both of which need to be a string. We will explore what each of these parameters is used for shortly.
Additionally, PHP will return a string containing the base name based on the values fed in through its parameters.
Below you can see how the basename() function is defined within PHP. This definition shows you each parameter and its required data types. Additionally it also shows you what the function will return
basename(string $path, string $suffix = ""): string
With the definition of the function in mind, let us explore what the two parameters are used for.
$path
– The path parameter is where you will define the path you want to get the base name from.
This parameter must be a string and should be a valid directory or filename.$suffix
– Using the suffix parameter, you can strip out any text that is located at the end of the retrieved base name.
For example, setting a suffix of “.png
” with a path of “/example/pimylifeup.png
” will return “pimylifeup
“.
There are a couple of things that you must note when using the function that we will quickly go over.
- First, the basename() function is not aware of path components provided by the filesystem. For example, components such as “
..
” and “.
” are not handled. - Second, this function does not understand whether the actual path exists on the current filesystem. It operates naively on the string that is provided to it.
- Finally, the basename() function in PHP is local-aware. If you provide a multibyte character path, the matching locale must be set utilizing the setlocale() function.
If the locale does not match what is provided in the string, then this function’s behavior is undefined.
Using the basename() Function in PHP
Now that we have walked through how the basename() function is defined within PHP let us put it to use.
In the following examples, we will show you can use this function and the data you expect to get back from it.
Basic usage of basename()
Let us start by exploring the most basic usage of PHP’s basename() function. That basic usage is only to use the first parameter.
The first parameter, as mentioned earlier, is where we will be specifying the path.
For this example, we will utilize the path “/usr/example/pimylifeup.html
“, which we will assign to a variable called “$path
“.
We then pass this variable into the “basename()
” function, using PHP’s echo statement to print the resulting string.
<?php
$path = "/usr/example/pimylifeup.html";
echo basename($path);
?>
After running the above example, you should end up with the following string. You can see PHP printed only the trailing part of the path.
pimylifeup.html
Stripping out of the Suffix from PHP’s basename() Function’s Result
Now let us explore the second parameter of basename(). This parameter allows you to strip the suffix from the string returned by PHP’s basename() function.
This is useful when you want something like a file extension stripped from the result.
To showcase this, let us take the same path that we used in our previous example (“/usr/example/pimylifeup.html
“) and assign it to a variable called “$path
“.
This path is then passed into the first parameter of our basename() function call.
However, for the second parameter, we will be using the string “.html
“. This string is what will be stripped from the resulting basename.
<?php
$path = "/usr/example/pimylifeup.html";
echo basename($path, ".html");
?>
The example above will print the following result. From this result, you can see that specifying the suffix stripped it from the resulting basename.
pimylifeup
Conclusion
Within this guide, we have shown you how you can use the basename() function in PHP.
The basename() function is helpful for quickly getting the trailing component of a path. This is useful when you either only want the ending directory or filename.
Please comment below if you have any questions about using this function within PHP.
To learn more, check out our many other PHP tutorials. Alternatively, we have many other programming guides if you want to learn a new language.