In this tutorial, we will be showing you how to use assignment operators in JavaScript.
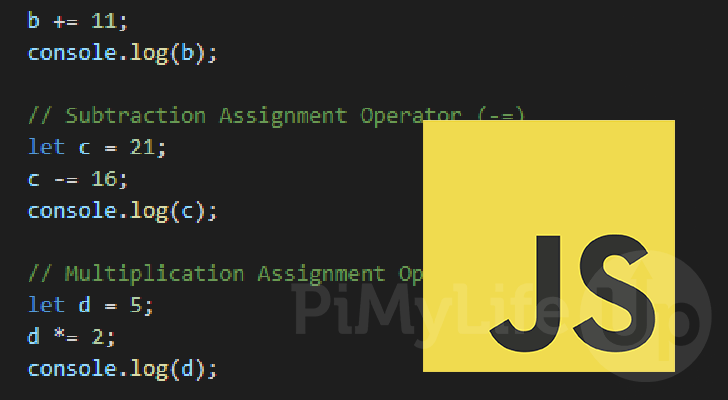
Assignment operators allow you to assign a new value to a variable easily. They take the operand on the left and assign the right operand to it.
JavaScript also has assignment operators that allow you to perform arithmetic operations during the assignment. These are super useful and allow you to write cleaner, more condensed code.
Let us start this guide by showing off the various assignment operators that JavaScript supports within its code.
Using the table below, you can see each assignment operator and what they do. You can also use this to see the equivalent code to using the assignment operator.
Operator | Name | Example | Equivalent | Result |
---|---|---|---|---|
= | Assignment | x = y | x = y | x is set to the value of y |
+= | Addition Assignment | x += y | x = x+ y | y is added to x |
-= | Subtraction Assignment | x -= y | x = x – y | y is subtracted from x |
*= | Multiplication Assignment | x *= y | x = x * y | x is multiplied by y |
**= | Exponentiation Assignment | x **= y | x = x ** y | x is raised to the power of y |
/= | Division Assignment | x /= y | x = x / y | x is divided by y |
%= | Modulus Assignment | x %= y | x = x % y | Remainder of x divided by y |
Example usage of Assignment Operators in JavaScript
In the following sections, we explore how you use each assignment operator in JavaScript.
Alongside showing you how to use them in your code, we also explain what they do. If you are new to JavaScript, it is well worth trying to remember how each of these works.
Assignment Operator in JavaScript (=
)
The assignment operator is one of the simplest to understand in JavaScript. All this operator does is assign the right-side value to the variable on the left side of the operator.
For the example below, we will start by defining a variable called “x
” and assigning it the value 15
.
We will then use the assignment operator again to change the value of this variable to 10
. The final value of “x
” is logged to the console.
let x = 15;
x = 10;
console.log(x);
Since the last value we assigned to “x
” was 10
, that is the result you will get from the above code.
10
Addition Assignment Operator (+=
)
JavaScript has a variety of operators that allow you to modify a value on assignment. This operator allows you to increase the left-side operand by adding the right-side operand.
In this example, we will start by declaring a variable with the name “x
“, and assign it the value of 10
.
We then use JavaScript’s addition assignment operator (+=
) to increase the value of our “x
” variable by 11
.
let x = 10;
x += 11;
console.log(x);
Below, you can see how this operator increased the value of our “x
” variable. The most significant advantage of this operator is that it is much cleaner to write than “x = x + 10;
“.
21
Subtraction Assignment Operator in JavaScript (-=
)
The subtraction assignment operator (-=
) allows you to decrease the left variable by the right value. The biggest advantage of this operator is that it allows you to write cleaner code.
To showcase this operator in JavaScript, we will start this example by declaring a variable called “x
” with the value 21
.
We then use the subtraction assignment operator to subtract “16
” from the value of our “x
” variable.
The updated value of the “x
” variable is then logged to the console.
let x = 21;
x -= 16;
console.log(x);
From the code above, you will end up with the following result. With this result, you can see how the value of “x
” was subtracted by 16
, and JavaScript assigned the result back to the “x
” variable.
5
Multiplication Assignment Operator (*=
)
The multiplication assignment operator in JavaScript allows you to multiple the value of a variable and assign it the result.
To use this operator, you specify your variable, followed by the operator (*=
), followed by the amount you want to multiply the number by.
To showcase this, we will start the example script by defining a variable called “x
” and assigning it the value “5
“.
We then utilize the multiplication assignment operator to multiply our “x
” variable by 2
.
let x = 5;
x *= 2;
console.log(x);
After running the example code, you should end up with the result below. With this, you can see how this operator multiplied our “x
” variable by 2
and assigned it the new value.
10
Exponentiation Assignment Operator in JavaScript (**=
)
The exponentiation assignment operator (**=
) allows you to raise the value of your variable by the right-side operand, with the resulting value being assigned back to the variable.
With the example below, we start by defining a variable called “x
” and assign it the value of 10
.
We then use this operator to raise our “x
” variables value by 3
and assign the value back to “x
“. Finally, the value in the “x
” variable is logged to the console by using the “console.log()
” function.
let x = 10;
x **= 3;
console.log(x);
After raising 10
to the power of 3
, you should end up with the following value stored in the “x
” variable.
1000
Division Assignment Operator (/=
)
To divide a variable by another value and assign that result back to the variable, you can use the division assignment operator (/=
).
The division assignment operator in JavaScript is written using a slash symbol (/
) followed by the equals symbol (=
).
Let us write a quick example in JavaScript to show you how this works. We start by declaring a variable (x
) and assigning it the value 1000
.
On the next line we divide our “x
” variable by 100
, assigning the result directly back to the “x
” variable.
let x = 1000;
x /= 100;
console.log(x);
After the example above is run, you will end up with the result below. This shows that our “x
” variable was assigned the result of 1000
divided by 100
.
10
Modulus Assignment Operator in JavaScript (%=
)
The final operator we are looking at is the modulus assignment operator (%=
). This operator allows JavaScript to get the remainder from a division operation (Modulus).
In this case, the variable on the left is divided by the right-hand operand. The remainder from this division is assigned back to the left-side variable.
To showcase the modulus assignment operator, we will write a short example. This example starts with us declaring the “x
” variable and assigning it the value 10
.
We then use JavaScript’s modulus assignment to get the remainder of x
divided by JavaScript will assign the result of this arithmetic operation back to the “x
” variable.
let x = 10;
x %= 8;
console.log(x);
By running this example, you will see how the “x
” variable is assigned the value of 10
modulus 8
(2).
2
Conclusion
In this tutorial, we went through and explained how each of the assignment operators works in JavaScript. These are a fundamental part of the JavaScript language.
Please comment below if you have any questions about how the assignment operators work.
Be sure also to check out our many other JavaScript tutorials. Alternatively, we have a wealth of other programming guides if you want to learn a new language.