In this guide, we will show you all of the arithmetic operators supported by JavaScript and how to use them.
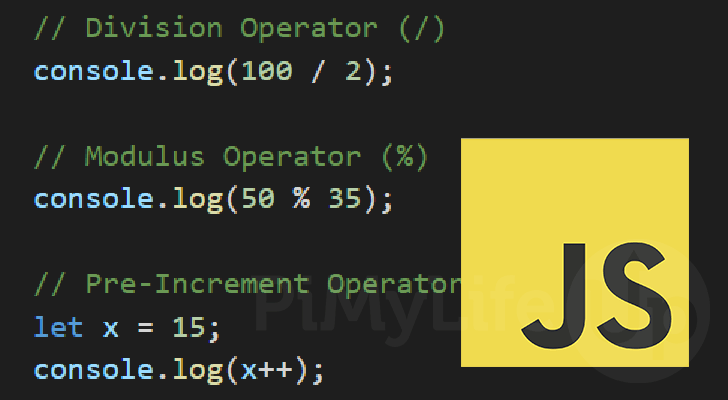
Arithmetic operators are what allow you to modify numeric values within JavaScript with ease.
These operators cover most basic math operations you would want to perform in JavaScript.
An example of some math that you can perform within JavaScript easily is addition, subtraction, multiplication, exponentiation, division, and modulus.
Over the following sections, we will show you the supported arithmetic operators, the symbols that represent these operators, and examples of how to use them within JavaScript.
Table of Contents
Supported Arithmetic Operators in JavaScript
Let us start by exploring the arithmetic operators that are supported by the JavaScript programming language.
The best way to show these supported operators is by using a table. The table below shows you all the information you need.
For example, for any chosen operator, you can see its name, an example of using that operator, and a short description of the result.
Operator | Name | Example | Result |
---|---|---|---|
+ | Addition | x + y | Adds x to y |
– | Subtraction | x – y | Subtracts y from x |
* | Multiplication | x * y | Multiplies x by y |
** | Exponentiation | x ** y | Raises x to the power of y |
/ | Division | x / y | Divides x by y |
% | Modulus (Division Remainder) | x % y | Returns remainder of x divided by y |
++x | Pre-Increment | ++x | Increments x by one, then returns the value |
x++ | Post-Increment | x++ | Returns the value, then increments x by one |
–x | Pre-Decrement | –x | Subtracts x by one, then return the value |
x– | Post-Decrement | x++ | Returns the value, then subtracts x by one |
To learn more about each of these arithmetic operators, then be sure to read on. We will give a quick example of each of these operators and explain more about what they do.
Examples of Arithmetic Operators in JavaScript
Now that you know all of the arithmetic operators supported in JavaScript, let us show an example of how each one works.
Within each of these examples, we will explain further what the operator is used for and what math operation they perform.
Addition Operator in JavaScript (+
)
JavaScript’s addition operator (+
) is straightforward to use and allows you to add two values together easily.
In our example below we simply add the number 10
to 10
and log the result to the console. All we needed to do was use the plus (+
) symbol in between our two values.
console.log(10 + 10);
After running the example below, you should end up with the following value.
20
Subtraction Operator (-
)
The subtraction operator (-
) in JavaScript is also straightforward to understand and use. This operator is used to subtract the right-hand operand from the left-hand operand.
With this example, we are subtracting 15
(right value) from 20
(left value). The result from this operation is logged to the console.
console.log(20 - 15);
This piece of JavaScript should produce the following result, with 15
being subtracted from 20
.
5
Multiplication Operator in JavaScript (*
)
The multiplication operator (*
) is the next JavaScript arithmetic operator that we are touching on. This operator allows us to multiply the left hand0operand by the right-hand operator.
To showcase this, we have the following example where we use the asterisk symbol (*
) to multiply 6
by 2
. The value returned by this operation is logged to the console.
console.log(5 * 2);
Below you can see how our number 5
was multiplied by the value 2
, giving us the value of 10
.
10
Exponentiation Operator (**
)
The exponentiation operator (**
) allows you to raise a number by another number within JavaScript quickly. To use this operator, you need to use two asterisk symbols one after another (**
).
To showcase this, in our example below, we will be raising 10
to the power of 2
. This would be the equivalent of writing “10 * 10
“. To showcase this operation’s end value, we log it to the console.
console.log(10 ** 2);
After using the JavaScript example above, you should have the following result logged to your console. From this result, you can see how using this operator JavaScript raised 10
to the power of 2
.
100
Please note that this is a newer JavaScript operator and requires support for ES2016.
Division Operator in JavaScript (/
)
The division operator in JavaScript allows you to divide a number by another. This operator is represented in JavaScript by the slash symbol (/
).
With this example, we will divide 100
by 2
and log the resulting value to the console.
console.log(100 / 2);
The result below shows how our use of the division operator divided our value of 100 by 2.
50
Modulus Operator (Division Remainder) (%
)
The modulus operator is somewhat similar to the division operator, but instead of returning the divided value, it only returns what is left over by the operation.
For example, dividing 12
by 10
will give us the remainder of “2
” since that was what was left over after JavaScript completed the division.
Let us show this operator at work within some actual JavaScript. The following example will use the percentage symbol (%
) to get the division remainder of 50
divided by 35
.
console.log(50 % 35);
Below you can see the result of this modulus operation. Since 35
only goes into 50
once, we will end up with a remainder of 15
.
15
Pre-Increment Operator in JavaScript (++x
)
The pre-increment operator (++x
) in JavaScript allows you to increase the value of a variable by one, then return the resulting value. This operator is represented by two plus symbols (++
) directly before your variable.
To showcase this, we start by declaring a variable called “x
” and assigning it the numerical value of 15
.
We then log the value of “x
” by using the “console.log()
” function.
let x = 15;
console.log(++x);
Since the value of “x
” is incremented before it is returned, you should end up with the following result.
16
Post-Increment Operator (x++
)
The post-increment operator (x++
) differs slightly from the “pre-increment” due to when the incrementing occurs. When using this operator in JavaScript, it will return the value of your variable, which will then be incremented by one.
The post-increment operator is used in JavaScript by using two plus symbols (++
) immediately after your variable.
With the example below, we start the script by declaring the “x
” and assigning it the number 16
.
We then post-increment the value of “x
” and print the resulting value returned by this operation. We also log the value of our “x” variable immediately after.
let x = 16;
console.log(x++);
console.log(x);
Below you can see how the post-increment operator works. The first log prints the original value since the post-increment operator returns the value immediately.
However, the second log has the incremented value since JavaScript updated it after the operation returned a value.
16
17
Pre-Decrement Operator In JavaScript (--x
)
The pre-decrement operator (--x
) in JavaScript allows you to decrease the value of a variable by one and then return the resulting value. You can utilize this operator by using two minus symbols (--
) immediately before your variable name.
With the example below, we start our script by declaring a variable called “x
” and assigning it the value of 17
.
We then utilize JavaScript’s pre-decrement operator on our “x
” variable and log the resulting value.
let x = 17;
console.log(--x);
The result below shows that JavaScript decremented the value of our “x
” variable by 1
.
16
Post-Decrement Operator (x--
)
JavaScript also has an arithmetic operator called the “post-decrement” operator (x--
). This operator returns the value of the variable, and then decrements the value of variable by one.
You can utilize this operator by using two minus symbols (--
) immediately after your variable name.
With our JavaScript example below, we start by declaring a variable called “x
” and assign it the value 16
.
For our first “console.log()
” call, we use the post-decrement operator to decrease the value of our “x
” variable. Since this is a “post” decrement, the returned value will be the original one, not the decreased value.
We use a second “console.log()
” to show you how the value is only updated after it has been returned.
let x = 16;
console.log(x--);
console.log(x);
The two values below show how the post-decrement operator works in JavaScript. Since the value is only updated after it has been returned, you will first get the same value back.
16
15
Conclusion
This guide shows you the various arithmetic operators supported in JavaScript and how to use them.
These operators allow you to perform math on your values within your scripts easily.
Please comment below if you have any questions about using any of the arithmetic operators in JavaScript.
Be sure to check out our many other JavaScript tutorials to learn more. We also have a wealth of other coding guides if you want to learn a new language.