In this tutorial, you will be learning about logical operators in JavaScript.
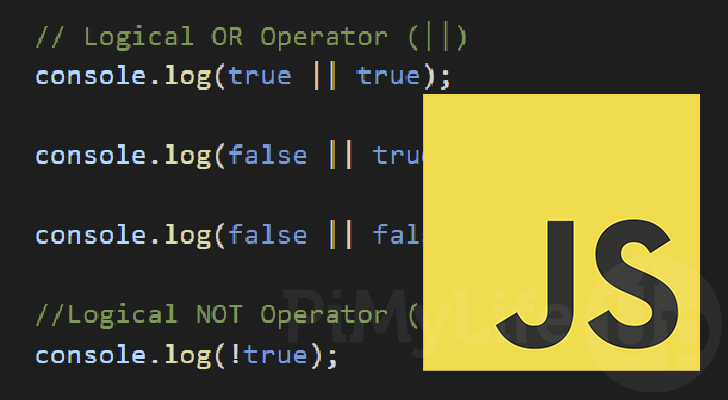
In JavaScript a logical operator performs operations based on the logical output of two operands. A logical result is a value that is either true
or false
.
Using the logical operator, you can easily perform operations based on whether two operands are true
or false
. There is also a logical operator in JavaScript that returns the inverse, so false
when true
, and true
when false
.
Learning these logical operators are a crucial part of JavaScript and are often combined with the many other JS operators.
These operators also make up a vital part of conditional if statements in JavaScript, helping you easily chain together multiple operations and saving you from having to nest multiple if statements.
Below we have included a table showcasing each logical operator supported within JavaScript. Using this table, you should get a good understanding of what each operator does.
Operator | Name | Example | Result |
---|---|---|---|
&& | AND | x && y | true if both x and y are true |
|| | OR | x || y | true if x or y are true |
! | NOT | !x | true if x is false |
Of course, the table only shows you so much. If you feel like you need to learn more about logical operators, be sure to continue reading.
We will cover each of these operators showing you their possible results and how to use them.
Examples of Logical Operators in JavaScript
In this section, we will explore each logical operator that JavaScript supports. From the following sections, you should learn how each of these operators are used, and what they are used for.
We have also included a truth table for each value so you can see the possible results you will get depending on the values fed to the operator.
AND Operator (&&
) in JavaScript
The “AND” logical operator (&&
) in JavaScript allows you to compare two operands to see if they are both true
.
If both values are true
, then the operator will return true
. If either value is false
, then the operator will return false
.
The logical AND operator is written first with the left operand, then the two ampersand symbols (&&
), and finally the right operand. The left operand will be compared to the right operand.
a && b
Using the truth table below, you can observe the possible results from the logical operator in JavaScript. This table shows the cases in which the “AND” operator would return false
or true
.
Using this you can see that it will only ever return true
if both operands are true
.
a | b | a && b |
---|---|---|
false | false | false |
false | true | false |
true | false | false |
true | true | true |
When JavaScript evaluates the AND operator, it will check the left operand first before checking the right operand. It will only check the right operand if the left operand is true
.
This can be helpful to know when using “AND” to compare the results from two functions. Using the more lightweight function as the first call can help save some CPU time.
Example of the AND Operator
Let us show you two quick examples to showcase how the AND operator works in JavaScript. By using “console.log()
“, we can easily see the values returned by this logical operator.
- The first usage of the “AND” logical operator will return
true
.
This returns true because the left and right operands are “true
“. - The second expression will return
false
.
This is because one of the operands is “false
“. As mentioned earlier, the “AND” operator requires both operands to betrue
.
console.log(true && true);
console.log (false && true);
The results below show how JavaScript evaluated the AND (&&
) logical operator in both of our examples.
true
false
Logical OR Operator (||
) in JavaScript
Using the OR operator (||
) in JavaScript, you can compare two operands and return true
if either the left or right operand is true
.
The logical OR operator is written in JavaScript by first specifying the left operand, followed by the two pipe symbols (||
), and finally the right operand.
a || b
To understand the logical output of the OR operator, you can view the table below. The table below shows what the or operator (||
) will return depending its operands’ value.
In this table, the left operand will be called “a
“, and the right operand will be called “b
“.
This table shows that the OR operator returns true
as long as one operand is true
.
a | b | a || b |
---|---|---|
false | false | false |
false | true | true |
true | false | true |
true | true | true |
When JavaScript executes the OR logical operator it will first check the left operand to see if it is true
. After that, it will only check the right operator if the left operand is false
.
Like with the AND operator, this behavior can be useful to know for optimizing your JavaScript code.
If you have a function that returns fast, you might want to utilize it as the left operand, allowing the OR operator to return quickly if the value is true
.
Example of the OR (||
) Operator
In the example below, we have three different usages of the OR operator. We will quickly explain the result of each of these expressions and why.
- With the first example, JavaScript will return
true
.
The or operator is satisfied by the left operand being true. While the right operand is alsotrue
, JavaScript won’t check it. - The next example will also return
true
.
While the left operand isfalse
, the right operand istrue
, satisfying the requirements of the logical OR operator. - The final example returns
false
since both the left and right operands are false.
console.log(true || true);
console.log(false || true);
console.log(false || false);
Thanks to us using the “console.log()
” function, you can see how JavaScript evaluated each use of the OR logical operator.
true
true
false
Logical NOT Operator (!
) in JavaScript
JavaScript’s logical NOT operator (!
) allows you to check if a value is not true
. You can almost consider this operator to return the inverse of whatever logic was returned.
One thing to note is that this logical operator only works on a single operand at a time. If you want to get the inverse of multiple operands, you must use this operator on each of them.
The NOT logical operator is written in JavaScript using an exclamation mark (!
), followed by your operand.
!a
The table below shows how the NOT operator returns true
when the operand is false
. It will also return false
when the operand is true
.
a | ! a |
---|---|
false | true |
true | false |
Example of the Logical NOT Operator (!
) in JavaScript
To showcase this, let us write a short example showing what is returned by the NOT operator.
- The first example will return false since we use the logical NOT operator on a “true” value.
- With the second example, the NOT operator will return
true
. This is because we are using it on a “false
” value.
console.log(!true);
console.log(!false);
Below you can see the results of our NOT expressions logged to the console.
false
true
Conclusion
Throughout this guide, we have shown you the various logical operators supported by JavaScript.
You should now know how to use the logical NOT, OR, and AND operators within your code.
Please comment below if you have any questions about logical operators.
If you want to learn more about JavaScript, we have a variety of other tutorials. We also have a wealth of tutorials if you want to learn a new programming language.