In this tutorial, we will be showing you how to use if, else, and elif conditional statements in Python.
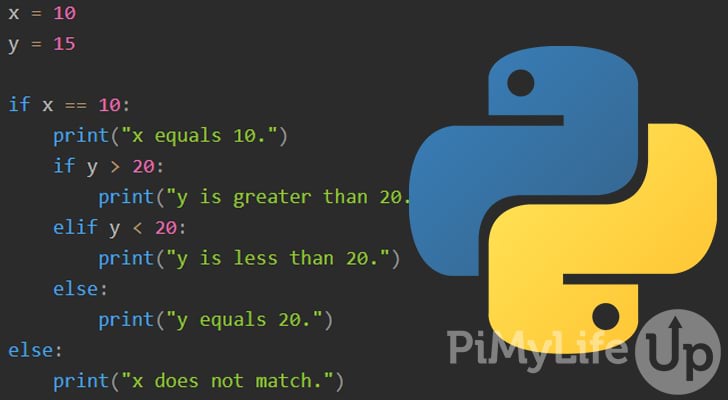
Conditional statements are a programming fundamental that you will need to know if you wish to program software using Python or any other programming language.
By using conditional statements, you can control the execution of code. The statement conditions will need to evaluate to be true or false. Depending on the result, you can skip or execute specific code blocks.
This tutorial will go through the many ways you can structure conditional statements. We will go through how to correctly write if, if else, if elif and, if elif else statements. We will also touch on nesting if statements.
To build a good expression for a conditional statement, you will need to understand how to use Python operators. Therefore, I highly recommend taking the time to learn about the different operators you can use.
If you want a shorthand way to write a conditional statement, I recommend learning more about ternary operators or conditional expressions. However, I strongly recommend that you learn the basics first.
Python does not have built-in support for switch statements, so you need to use if elif logic. However, in Python 3.10.0 and later, there is support for match and case statements, which are very similar to a switch.
Table of Contents
- The if Statement
- The if else Statement
- The if elif Statement
- The if elif else Statement
- Nested if Statements
- Conclusion
The if Statement in Python
The most basic Python conditional statement is the if statement. By using the if statement, you will be able to check to see if a condition evaluates to true or false. If the condition is true, the indented code will run. Otherwise, it will skip past it.
As we mentioned earlier, the code block for an if statement will need to be indented. You can use a single space or four spaces as long as the spacing is consistent for the indentation. If you do not use the correct indentation, an error will occur.
Below is an example of how you can structure an if statement in Python.
if condition:
#Code here will be executed if the condition is met
Example of Using the if Statement
In our example below, we have a simple Python script that uses an if statement to check the value of x. Our if statement condition is that x must equal 15. If the condition evaluates to true, the block of code will run. Otherwise, it will not run.
x = 15
if x == 15:
print("My x value is 15.")
Since x
equals 15
, the code block containing our print function will run, resulting in the output below.
python ifelse.py
My x value is 15.
The if else Statement in Python
You can add an else statement after an if statement if you want a specific code block to run if the condition of our if statement is not met.
So, if our if statement condition evaluates to false, the else code block will execute. However, if the condition evaluates to true, the if statement code block will run and our else block will be skipped.
if condition:
#Code here will be executed if the condition is met
else:
#If none of the previous conditions were met, run this code
Example of Using an if else Statement
This example will demonstrate how an if else statement works within Python.
Our if statement checks whether the value of x is equal to 15. If the condition evaluates to be true, it will run the if statement code block. Otherwise, it falls to the else code block.
x = 12
if x == 15:
print("My x value is 15.")
else:
print("x does not match")
As our x value does not equal 15, the condition will be false, so our else code block will be executed instead. As you can tell in our output below, the else statement code block ran.
python ifelse.py
x does not match
The if elif Statement in Python
If you would rather your else statement have a condition, you can use an elif statement instead. The elif statement is the same as an “else if” statement found in other programming languages.
An elif statement will check its condition when the previous if statement’s condition is false. You can have multiple elif statements after a single if statement. Python will check each condition in order until a condition returns true.
if condition:
#Code here will be executed if the condition is met
elif condition:
#Code here will be executed if the elif condition is met and if condition is not met
Example of Using the if elif Statement
In this example, we demonstrate how an elif statement works.
At first, our if statement checks whether x equals 15. If it doesn’t, it moves onto the elif condition. Our elif condition will return true if x equals 12.
x = 12
if x == 15:
print("My x value is 15.")
elif x == 12:
print("My x value is 12.")
Since our x value does not meet the if condition but meets the elif condition, the following was outputted when we executed the Python script.
python ifelse.py
My x value is 12.
The if elif else Statement in Python
You can use a combination of all three of the examples above. However, there are a few conditions, such as an if statement must always come first.
You can have many elif statements, but the first elif must come after an if statement. Lastly, an else statement must come last as it will only run when other conditions are not met.
The example below demonstrates how you would structure an if, elif, and else statement.
if condition:
#Code here will be executed if the condition is met
elif condition:
#Code here will be executed if the elif condition is met and if condition is not met
else:
#If none of the previous conditions were met, run this code
Example of Using the if elif else Statement
The code example below demonstrates how a combination of an if, elif, and else statement will work.
Our first if statement checks if x equals 15. If it doesn’t, Python moves onto the elif statement.
The elif statement checks if x equals 12. If it doesn’t, the code will fall into our else statement.
x = 10
if x == 15:
print("My x value is 15.")
elif x == 12:
print("My x value is 12.")
else:
print("x does not match")
Our x value is 10, so it does not match the conditions in both our if and elif statements. Since x doesn’t match either condition, the else code block is executed. You should get the following output if you run the above Python script.
python ifelse.py
x does not match
Nested if Statements in Python
A nested if statement is as simple as placing an if statement inside another if statement. By doing this, you can create a complex logic flow for decision making.
It is good practice to keep the depth of nesting to a minimum, as the more levels you have, the harder the code can be to understand.
The example syntax below demonstrates a nested if statement.
if condition:
#Code here will be executed if the condition is met
if condition:
#Code here will be executed if the condition is met
else:
#If none of the previous conditions were met, run this code
Example of Using a Nested if Statement
Our example code below demonstrates a nested if statement. We use declare two variables, x and y with int values.
The outer if statement checks if x equals 10. If it does, we print out our first line and then reach our nested if statement.
Our nested if statement checks to see if y is greater than 20. If it isn’t, it moves to our elif condition.
The elif condition checks to see if y is less than 20. If that condition is not met, it moves into our nested else code block, which prints out a string.
x = 10
y = 15
if x == 10:
print("x equals 10.")
if y > 20:
print("y is greater than 20.")
elif y < 20:
print("y is less than 20.")
else:
print("y equals 20.")
else:
print("x does not match.")
The output below is the result of running the above Python script. Our x value matches with the outer if statement, but our y value didn’t match the nested if or elif condition, so the else code block was executed.
python ifelse.py
x equals 10.
y is less than 20.
Conclusion
I hope that this tutorial has taught you all the basics of using if, else, and elif statements to control the code execution in Python. Understanding if else statements is a programming fundamental, so I recommend practicing using these in a basic Python script.
We have many more Python tutorials that I recommend looking at if you wish to learn more. For example, our tutorials on Python while loops or Python for loops will be useful for controlling the execution of code.
Please let us know if you notice a mistake or if an important topic is missing from this guide.