In this tutorial, we will be going through and explaining how variables are handled in Python.
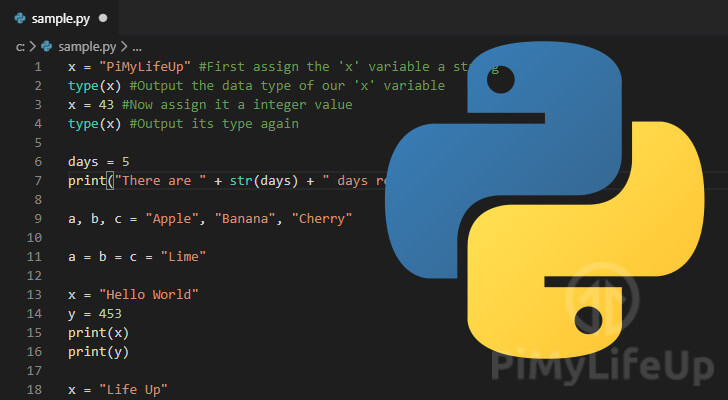
Variables are an essential part of all programming languages, and Python is no different.
You will be using variables within Python to store various bits of information, from strings to numbers.
Throughout this tutorial, we will be explaining to you several aspects of variables in the Python programming language.
One important thing to note about Python is that every variable is also considered an object, this is due to Python being completely object orientated.
Assuming you’re just starting out with Python, we highly recommend that you check out our beginner’s guide to Python.
If you would like to skip to a particular section in this tutorial, then feel free to make use of our table of contents below.
- Variable Names in Python
- Declaring Variables in Python
- Assigning Multiple Values to Multiple Variables
- Assigning a Single Value to Multiple Variables
- Outputting Variables in Python
- Combining Variables in Python
- Deleting Variables in Python
Variable Names in Python
One of the first things that you should learn is how you should be naming your variables in Python.
Like most programming languages, you can’t just write anything as your variable name.
There are a few different rules that you must follow when naming your variables in Python. These rules are quite simple and should be reasonably easy to understand.
● You can not start a Python variable with a number. You must only begin a variable name with a letter or an underscore.
For example, 0x
is an invalid variable name, but x0
and _0x
are considered valid.
● A Python variable must only contain uppercase and lowercase letters ( A–Z a–z ), digits ( 0 – 9 ), and the underscore character ( _ ).
To showcase what a valid variable name looks like in Python, we have listed some examples below.
- pimylifeup
- P1MyL1feUp
- pi_MY_life_UP
- pI_007
All of these variables are considered valid under Python’s variable name rules.
The reason for this is that the names don’t start with a number, and they only contain alphanumeric and underscore characters.
● Variable names in Python are case sensitive. Two variables with the same name but different casing are considered as two different variables.
For example, websitename
is considered a different variable to websiteName
because of the capitalization of the letter “N“.
Declaring variables in Python
Declaring variables in Python is a straightforward task as you do not have to worry about things that other languages are more strict on.
With Python, a variable is dynamically created as soon as you assign a value to it.
x = 5
y = "pimylifeup"
Copy
You do not need to worry about declaring a particular type when defining your variables.
In Python, the interpreter dynamically works out the data type when a value has been assigned to the variable.
The data type of a variable can even change after its declaration.
For example, the following shows us creating a variable called x
that contains a string, then changing it to a numeric value so that the data type switches from a string to an integer.
x = "PiMyLifeUp" #First assign the 'x' variable a string
type(x) #Output the data type of our 'x' variable
x = 43 #Now assign it a integer value
type(x) #Output its type again
Copy
Using our little example above in Python, you can see how the interpreter automatically changes the type of the variable.
We use the type()
function to output the type of the variable.
You can see how the data type changes as the variable changes by checking out the output of the code snippet above.
>>> x = "PiMyLifeUp"
>>> type(x)
<type 'str'>
>>> x = 43
>>> type(x)
<type 'int'>
Copy
Assigning Multiple Values to Multiple Variables
If you want to define values to multiple variables on one line, then you are able to in Python.
The way to do this is to list your variables separated by commas “,
” followed by the equal sign, then the values you want to assign separated by commas.
The order of your variables is the same order in which the values will be assigned.
For example, if we wanted to assign three separate values ("Apple"
, "Banana"
, "Cherry"
) to three variables (a
, b
,c
), we can do this on one line by doing the following.
a, b, c = "Apple", "Banana", "Cherry"
Copy
Please note that you should only do things like this when it doesn’t affect the readability of your code in a negative way. Remember that longer code isn’t necessarily bad code.
Assigning a Single Value to Multiple Variables
Python also allows you to assign a single value to multiple variables on one line.
This behavior could be useful if you wanted to reset multiple values to 0
for example.
To assign a value to multiple variables, you need to separate each of your variables using the equals sign (=
). The final equals sign should point to the value you want to assign to your variables.
For example, if we want to assign the value "Lime"
to our three separate variables (a
, b
, c
), then we can by doing the following.
a = b = c = "Lime"
Copy
Outputting Variables in Python
We can output the value of variables to the console in Python easily by making use of the print()
function.
This function is available in both Python 2.7 and Python 3 and is the recommended way for printing text to the console.
Below is an example of declaring two variables called x
and y
and outputting the value of both of them by using the print()
function.
x = "Hello World"
y = 453
print(x)
print(y)
Copy
If you run the sample code above, you should end up with the following two lines in your console.
Hello World
453
You can see how the value of both variables was outputted using the print()
function.
Combining Variables in Python
You can also combine variables in a print statement using the plus sign (+
) operator.
This operator will work as long as both values are of the same data type.
Concatenating Strings
When the variables involved are all strings, then the strings are all concatenated.
Here is an example of combining a variable, x
, with an inline string in a print statement.
x = "Life Up"
print("Pi My " + x)
Copy
We can also do the same thing using two variables that are both strings.
For example, if we have a variable called x
with a value of "Pi My "
, and a variable called y
with a value of "Life Up"
then we can combine them by using x + y
.
x = "Pi My "
y = "Life Up"
print(x + y)
Copy
The output you will get from both of these samples is the following. You will see that both the x
and y
variables have been concatenated.
Pi My Life Up
Adding Integers Together
If the data type of both variables is an integer or float, then the plus sign (+
) will work as a mathematical operator
Here we have an example of combining a variable x
that has a value of 32
with the number 5 within the print()
function.
x = 32
print(5 + x)
Copy
You can also do the same thing by combining two variables as long as they are both numeric. Python does not like mixing data types when combining variables.
x = 5
y = 32
print(x + y)
Copy
The result from both of these code samples should end up being the same.
37
Combining Strings and Integers
With Python, you can’t just combine an integer and a string. To connect the different data types, you need to convert the string to a number or the number to a string.
If you try to combine a string with a number, you will run into an error like below, stating that the data types cannot be joined.
TypeError: cannot concatenate 'str' and 'int' objects
There is a way we can get around this error, and that is by converting the data to the type we need.
Converting to a string
For the first part of this section, we will walk you through converting a number to a string as there is less we need to worry about.
To convert a number like an integer or float to a string in Python, we can make use of the str()
function.
This Python function automatically converts any other Python data type into a string.
A number, for example, would go from being the number 5
to a string that contains the number 5 like so "5"
.
For example, if we wanted to print out the text “There are 5 days remaining“, with 5
being an integer stored in a variable called days
, we need to wrap the days
variable in the str()
function.
You can see how the str()
function works in practice with the little code snippet below.
days = 5
print("There are " + str(days) + " days remaining")
Copy
If you were to go ahead and run this code snippet, you should see the string be outputted without any errors.
There are 5 days remaining
There are also other methods for converting numbers to a string, but we will delve further into that on our guide on Strings in Python.
Converting to a number
Of course, that is only good if you want to deal with strings, there are also times where you will want to convert a string to a number.
Using Python, we can convert variables from a string to an integer using the int()
function, and to a float by using the float()
function.
For example, let’s say that we have a variable called wage
that has the value "10"
assigned to it. Now, we want to add the number 15
to this number so we will store that in our bonus
variable.
To be able to add the number to the string, we will need to wrap our wage
variable in our int()
function.
Below we have written up a little code sample showing how you can do this.
wage = "10"
bonus = 15
print(int(wage) + bonus)
Copy
From this snippet, you should get the following result, with the string "10"
converted to a number and added to the bonus
variable.
25
With this result, we can see that our wage
variables value has been converted to int and then added to our bonus variable successfully.
The same method will also work for floating numbers stored in a string such as "12.5"
. The only change you need to make is to switch the int()
function with the float()
function.
We will take a deeper dive into numbers in Python in a later tutorial.
Deleting Variables in Python
If you ever want to delete a variable in Python, there is a couple of ways to do this.
There are a few different reasons why you may want to delete the contents of a variable. One of the main reasons you would want to delete a variable is to help free up memory faster.
The first way is to wait for Python’s garbage collector to take care of the variable. Python automatically keeps track of how many references an object has. Once the reference count reaches zero, the garbage collector automatically frees up the used memory.
Of course, this may not be the most efficient way of deleting variables. You are also able to do this yourself by making use of the del
keyword.
The keyword is reasonably simple to use. You just need to use del
followed by the variable you want to delete. Like so del x
Once a variable has been deleted, Python will automatically free up its used memory when the garbage collector fires next.
You can see the delete keyword in action in our following code snippet.
x = "PiMyLIfeUp"
print(x)
del(x)
print(x)
Copy
When you run this code snippet you should run into an error stating that the variable name x
is not defined.
>>> x = "PiMyLIfeUp"
>>> print(x)
PiMyLIfeUp
>>>
>>> del(x)
>>>
>>> print(x)
Traceback (most recent call last):
File "", line 1, in
NameError: name 'x' is not defined
Copy
It’s important to note that even when a variable is deleted, its memory will still be used until the Python garbage collector cleans up the memory. Python offers no guarantee on when memory will be cleared.
Hopefully, at this point, you will now have a good understanding of how variables work in Python.
You should also have an idea of how to use variables in your scripts and also be able to combine and print out their values.
In our next guide we will be explaining to you the difference between local and global variables in Python.
If you have run into any issues with this tutorial on Python Variables then feel free to drop a comment below.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support