In this tutorial, we will go through everything you need to know to write a valid for loop in Python.
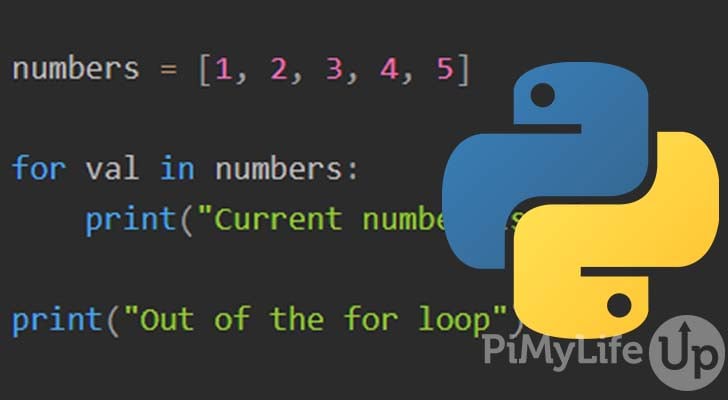
In programming, for loops are an essential part of controlling code execution. Therefore, it is highly recommended that you can read, write, and understand how for loops operate in all programming languages.
Python handles for loops slightly differently from other languages and instead treats them like a foreach loop. In the loop statement, you must specify an object such as a list. Next, Python will iterate through the object until it reaches the end.
Like while loops, you can nest for loops inside each other, the inner loop will execute on every iteration of the outer loop. This flow will be useful for handling certain data sets and situations.
Table of Contents
for Loop Syntax
A for loop in Python is a little different from other programming languages because it iterates through data. The standard for loop in Python is more like foreach. You will need to use an object such as a string, list, tuple, or dictionary. Alternatively, you can also use the range function.
The for loop will iterate through a data set until it reaches the end. You can use statements such as continue or break if you wish to skip an iteration or break out of the loop entirely.
Another way you can use a for loop is with the range function. The range function allows you to iterate through a sequence of numbers.
The syntax is pretty straightforward, and like all Python code blocks, everything inside the block will need to be tabbed by at least a space. Since Python is whitespace sensitive, any inconsistencies in tabbing will likely result in an error.
for iterator in sequence:
#Code here will be executed if the condition is met.
Copy
How to Use a for Loop in Python
In this tutorial, we will take you through several different ways you can use a for loop in Python.
If you ever need to process large data sets, you will likely need to use either a for loop or a while loop. So, it is essential that you have a good understanding of how loops work in Python.
I recommend checking out our Python getting started guide if you a new to the Python programming language. We cover most of the basics you need to know to get started.
Writing a Standard for Loop
In this example, we will be writing a standard for loop that simply iterates through a list. This method is likely how you will structure most of your for loops in Python.
In our script below, we first declare a list of numbers. Unfortunately, Python doesn’t support arrays, but a list is somewhat similar.
Next, we have our for
statement in which we declare a variable val
. Each iteration will update the val
variable to the current item in our list “numbers”. The loop will repeat until it reaches the last item in our list.
Inside our loop block, we have a print statement that prints the current value of val.
Lastly, we print a line stating we have finished and left the loop.
numbers = [1, 2, 3, 4, 5]
for val in numbers:
print("Current number is: ", val)
print("Out of the for loop")
Copy
Our output below shows that the loop starts from the first element in our list and stops once it reaches the last element. Once it reaches the last element, it exits the loop and prints the text “out of the for loop”.
Current number is: 1
Current number is: 2
Current number is: 3
Current number is: 4
Current number is: 5
Out of the for loop
Writing a for Loop using Range
You can use a for loop with the range function instead of an object. The overall structure of the for loop does not change, but you instead use range in the statement.
The range Function
The range function returns a sequence of numbers. By default, it starts at 0
and increments by 1
. It will continue to increment until it reaches a specified number
range(start, stop, step)
Copy
- Start is an optional parameter and specifies the starting position. The default value is
0
. - Stop is a required parameter and specifies the stopping position.
- Step is an optional parameter and specifies how much to increment on each iteration. The default value is
1
.
Example of a for loop using range
In this for loop example, we will be using the range function with a starting value of 0
and a stopping value of 5
. The val
variable will hold the current value of the range function.
Inside our for loop, we print the value of val
so we can see how the range function iterates until it hits our stopping value of 5
.
Lastly, we print that we have left the loop.
for val in range(0, 5):
print("Current number is: ", val)
print("Out of the for loop")
Copy
Below is the output of the code above. As you can see, the loop iterated through the range until it reached the stopping value of 5
.
Current number is: 0
Current number is: 1
Current number is: 2
Current number is: 3
Current number is: 4
Out of the for loop
Using else with the for Loop
You can use an else
keyword with a for loop in Python. Once the for loop has been completed, the else
block will run.
If you break out of the for
loop using the break
statement, the else
block will not be executed.
In our example below, we have a standard for loop but with the addition of the else statement.
numbers = [1, 2, 3, 4, 5]
for val in numbers:
print("Current number is: ", val)
else:
print("In the else code block.")
Copy
The output below demonstrates the else
code block executing after the for loop has iterated through the entire data set.
Current number is: 1
Current number is: 2
Current number is: 3
Current number is: 4
Current number is: 5
In the else code block.
Breaking Out of a for Loop Prematurely
There will likely be times when you require to exit the loop early. For example, if you hit a set of data or a specific requirement, you can break out of the loop and continue executing the rest of the code. All you need to do is use the break
statement inside the for loop.
The example below will have the for loop exit early once the val
variable equals 3
.
numbers = [1, 2, 3, 4, 5]
for val in numbers:
if val == 3:
break
print("Current number is: ", val)
Copy
The output only reaches two as the break statement ended the loop before it got to our print line.
Current number is: 1
Current number is: 2
Using continue Inside a for Loop
You may sometimes want to stop processing and skip to the next loop iteration. You can achieve this task by simply using the continue
statement.
In our example below, we use the continue keyword whenever the val
variable equals 3
. Of course, you can write more complex conditions, but we will keep it simple for this example.
numbers = [1, 2, 3, 4, 5]
for val in numbers:
if val == 3:
continue
print("Current number is: ", val)
Copy
In our output below, 3
has been skipped, and the code continued to execute until it reached the last number in our list.
Current number is: 1
Current number is: 2
Current number is: 4
Current number is: 5
Conclusion
It is essential to understand a for loop in Python, as you will likely need to use them quite a bit. Luckily they are an easy concept to grasp and follow roughly the same logic as for loops in other programming languages. I hope that this guide has shown you all the basics of writing a for loop in Python.
The while loop is another loop that I recommend learning if you plan on programming in Python. They are extremely useful as you can control them using a conditional statement. I highly recommend learning about them if you are new to programming.
If there is a topic that needs more explaining or we have got something wrong, please do not hesitate to leave a comment below.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support