In this tutorial, we will go through everything you need to know for writing a while loop in Python.
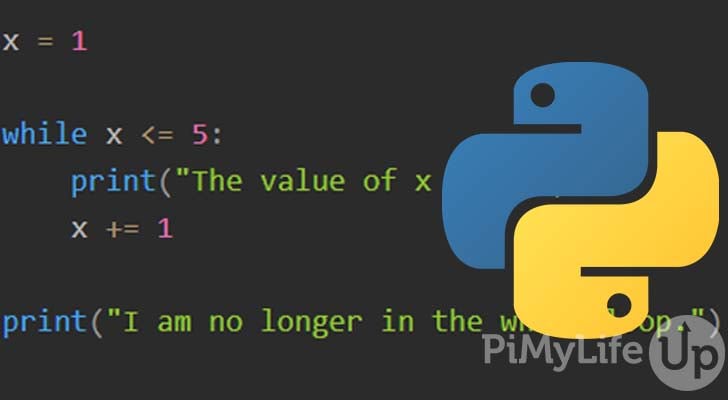
You will likely use while loops quite a bit, so it is a topic that I highly recommend learning if you are new to programming. Luckily, the core functionality of a while loop is the same across most programming languages. However, it is the syntax that may vary slightly.
Before executing the code inside the while loop, a condition must be met. Python will skip the code inside the while loop if the condition is not met. In addition, Python allows you to use an else statement with a while loop.
You can nest while loops within each other, but be careful with how many times you do this as it can lead to performance issues. There are typically less wasteful solutions than using multiple nested while loops, so make sure you plan your code well.
Table of Contents
while Loop Syntax
The syntax of a while loop in Python is slightly different from other languages, but it is mainly due to the way Python works. However, the behavior of the while loop is the same as in other programming languages.
A while loop will loop through the code until a condition turns false. Python will never execute the code inside the loop if the condition is not met at least once. Of course, it is also possible that the condition never turns false, and the code will be stuck in an infinite loop.
In our syntax example below, you can see how to structure a while loop. After the colon, all code you want included in the loop must be tabbed by at least a space. Python is whitespace sensitive, so ensure that your indention matches; otherwise, you may get errors or the code not executing correctly.
while condition:
#Code here will be executed if the condition is met.
How to Use a while Loop in Python
We will show you how to use a while loop in a Python program throughout the topics below. A while loop is one of the most basic ways you can control the flow of code, so you must understand how they work if you plan on programming any sort of Python application.
If you are entirely new to coding in Python, I suggest checking out our getting started guide for Python coding. It will take you through a lot of the basics, such as picking a good IDE (integrated development environment)
Writing a Standard while Loop
In our example below, we show you the fundamentals of writing a while loop in Python.
Firstly, we create a variable named x
and assign it the value of 1
.
For the while loop, we have a condition that says if x
is less or equal to 5
, proceed to execute the code inside the loop. As long as the condition remains true
, the code will continue to loop.
Inside the while loop, we print the value of x
to the terminal and then increment x
by 1
.
Lastly, we output that we are no longer in the while loop.
x = 1
while x <= 5:
print("The value of x is", x)
x += 1
print("I am no longer in the while loop.")
As you can see in our example output below, once x
equals 6
, the loop condition turns false
, and we exit the loop.
The value of x is 1
The value of x is 2
The value of x is 3
The value of x is 4
The value of x is 5
I am no longer in the while loop.
Using else with the while Loop
In this example, we do roughly the same as in the previous topic, but we add an else
statement after the while loop.
The else statement will run once the loop condition turns false. However, if you use break
or return
, the else code block will not execute.
The else block will run if the while condition is false. So in our example below, it will run once x
is greater than 5
.
x = 1
while x <= 5:
print("The value of x is", x)
x += 1
else:
print("I no longer meet the loop condition and now in the else block")
print("The final value of x is", x)
print("I am no longer in the while loop or else statement.")
In our output, you can see x
counts to the number 5
, then outputs the two print statements in our else
block. Lastly, it outputs that we have exited the while loop and the else block.
The value of x is 1
The value of x is 2
The value of x is 3
The value of x is 4
The value of x is 5
I no longer meet the loop condition and now in the else block
The final value of x is 6
I am no longer in the while loop or else statement.
Breaking Out of a while Loop Prematurely
There will be plenty of times when you want to end the loop prematurely. For example, to avoid pointless processing, you can break out of the loop once you have found the required piece of data. You can simply use the break
statement in Python to achieve this task.
In our example below, we have an extra if statement which states if y
equals 3
, break out of the loop.
y = 1
while y <= 5:
if y == 3:
break
print("The value of y is", y)
y += 1
print("I am no longer in the while loop statement.")
As you can see below, our loop outputs twice before meeting the if statement’s condition and therefore breaking out of the loop.
The value of y is 1
The value of y is 2
I am no longer in the while loop statement.
Using continue Inside a while Loop
There may be times you want to skip executing the current iteration of the while loop if it meets certain conditions.
In our example below, we iterate through our loop until z
equals 3
. Next, it hits the continue
statement and exits the current iteration early. Lastly, it starts the loop again, but only if the condition remains true.
z = 1
while z <= 4:
if z == 3:
z += 1
continue
print("The value of y is", z)
z += 1
print("I am no longer in the while loop statement.")
Our output below shows that the loop skipped printing the value when y
equaled 3
. This example is very basic, and you should find continue
very useful for many different applications.
The value of y is 1
The value of y is 2
The value of y is 4
I am no longer in the while loop statement.
Infinite while Loop
It is possible to create an infinite loop if you have a condition that will never be met. For example, you may accidentally write a condition that is always true or you may do it on purpose. Luckily, canceling a Python program stuck in an infinite loop is easy.
You may want to use an infinite loop when you need to process data continuously. Of course, you can always use a break statement if you need to exit the loop prematurely.
Below is an example of a while loop that will loop forever as the condition is set to True
.
z = 1
while True:
print("The value of y is", z)
z += 1
To terminate a Python script in an infinite loop, you will either need to CTRL + C in the terminal or kill the process manually.
Conclusion
Understanding a while loop is very important for programming in Python and most other languages. You will likely come across loops in most programs that you view and write yourself. I hope that this guide has helped you understand how to use a while loop in Python effectively.
Of course, there is one other type of loop that you can use in Python, and that is a for
loop. A for
loop is very useful for iterating through data such as lists, tuples, dictionaries, sets, or even strings. Therefore, I highly recommend learning how to use a for loop as it is essential for building good Python programs.
Please let us know if there is a topic that we can improve on within this tutorial by leaving a comment below.