In this tutorial, we will show you how to write a “do while” loop in JavaScript.
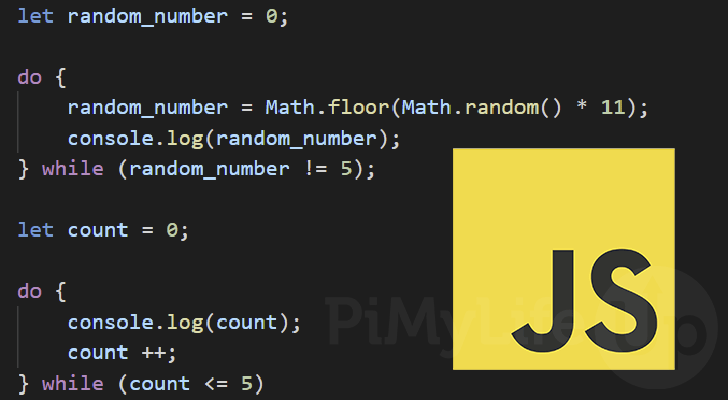
In JavaScript, the do while loop allows you to run code before the loop begins to run.
This style of loop is practical when you only want the loop to continue if an initial block of code yields a particular result.
For example, you can use a do while loop to grab paginated data from a server. You can then continue to grab more data if there is another page to process.
Over the next couple of sections, we will show you how to write a do while loop and give some examples of it in use.
Syntax of the do while Loop in JavaScript
This loop builds upon a standard JavaScript while loop, changing its characteristics slightly by allowing you to run code before the loop runs.
The do while loop in JavaScript is started off with the “do
” keyword, followed by a code block ({ }
).
Within this code block is the code that you want to run. JavaScript will run this code before the while condition is checked.
After the code block, you reference the “while
” keyword followed by the condition wrapped in brackets (( )
). If this condition is true, JavaScript will rerun your code block.
do {
//CODE TO BE RAN
} while(CONDITION);
Copy
Execution Flow of the do while Loop
The execution flow for the do while loop is still relatively simple. If you have already written a while loop before, you will be familiar with it.
It also has a far simpler execution flow than the JavaScript for loop.
To showcase the execution flow of the do while Loop within JavaScript, let us use the following example.
let random_number = 0;
do {
random_number = Math.floor(Math.random() * 11);
console.log(random_number);
} while (random_number != 5);
Copy
With our code snippet handy, let us quickly go through the loops execution flow.
- Before the loop begins, the code block (
{ }
) that is specified after thedo
keyword is executed.
In the example above, we generate a random number between0
and10
. - After the code block is run, the while condition is evaluated.
In our case, we check if our random number is not equal to5
.
If the condition istrue
, the loop will return to step 1. - Alternatively, if the while condition is
false
, the loop will terminate.
Example of the do while Loops in JavaScript
Now that we know the syntax of the do while loop, let us explore some examples of using it in JavaScript.
Both of these examples will be reasonably similar but will show different ways that you can utilize this kind of loop.
Basic Usage of a do while Loop
For this first example, we will write a straightforward do while loop in JavaScript. With this loop, we will count from 0
to 5
.
While not the best usage of a do while loop, it will give you an idea of how it operates.
We start our script by creating a variable called “count
” and assigning it the value of 0
.
In the “do{}
” code block, we log the value of our “count
” variable then increment it by one using the post-increment operator (x++
).
Finally, we have our “while()
” condition. Here we check if “count
” is less than or equal to 5
. If this is true, it will go back to the start of the loop.
let count = 0;
do {
console.log(count);
count ++;
} while (count <= 5)
Copy
Running this JavaScript, you will end up with a result similar to what we have shown below.
0
1
2
3
4
5
A do while Loop Generating a Random Number
For our second example, we will showcase a better usage of the do while loop in JavaScript. With this example, we will generate a random number for every loop, continuing to run till it hits the desired value.
We start our example by creating a variable called “random_number
” and assigning it the value of 0
.
On the following line, we start our JavaScript’s do while loop by using the “do
” keyword. Within this loop, we generate a random number between 0
and 20
. This number is generated using the “Math.random()
” functionality.
As “Math.random()
” generates a floating-point number, we use “Math.floor()
” to round it down to the closest whole number.
Finally, we use the “while()
” loop to check if “random_number
” is not equal to 10
. The loop will continue to run until the random number equals 10
.
let random_number = 0;
do {
random_number = Math.floor(Math.random() * 21);
console.log(random_number);
} while (random_number != 10)
Copy
Below you can see that the loops can end quickly or take a while. It shows you the advantage of the do while loop.
If the needed result is generated in the first run, then the loop will never need to run. It allows you to minimize repetitive tasks.
6
10
Conclusion
We have shown you how to write and use a do while loop in JavaScript in this guide.
A do while loop allows you to execute some code before the loop begins to run. This allows you to perform an action that might not necessarily need the loop to run.
If you have any issues with using this loop, please comment below.
Be sure to check out our other JavaScript coding tutorials, or if you want to explore another language, check out our other coding guides.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support