This guide will show you how to use the let keyword in JavaScript when declaring a variable.
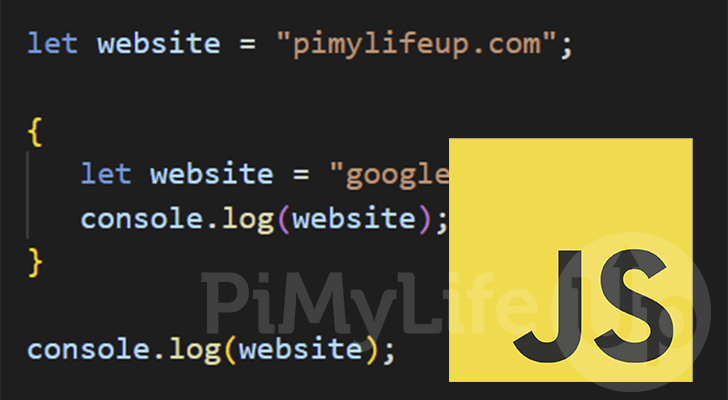
The let keyword is one of several ways to declare a variable within JavaScript.
Before the ES6 standard, you declared a variable in JavaScript using the var keyword.
Using the let keyword to declare a variable will have what is called “block scope”. This guide will explain what “block scope” is and why it is important.
When a variable is defined using the let keyword, it still has both global scope and function scope, just like you would with the var keyword.
Additionally, we show the difference in using the var keyword to declare your variables compared to the let keyword.
Hopefully, by the end of this guide, you will have a good understanding of declaring a variable using the let keyword.
Syntax of the let Keyword in JavaScript
Before we dive into things such as block scope, let us quickly go over the syntax of the let keyword in JavaScript.
To declare a variable using let in JavaScript, you only need to use “let
” followed by a variable name.
You can declare a variable without assigning it a value. However, it will be considered undefined by JavaScript until it is assigned a value.
let VARIABLENAME [= VALUE];
For example, if we declared a variable in JavaScript using the let keyword, we would use the following.
let pimylifeup;
We can also assign this a value during the assignment. For example, if we wanted to assign it the string “pimylifeup.com
” we would do the following.
let pimylifeup = "pimylifeup.com";
You Can’t Redeclare let Variables in the Same Block
One of the first things you should note is that when you declare a variable in JavaScript using let
, you cannot redeclare it within the same block scope.
This behavior is helpful because you cannot accidentally redeclare a variable declared within the same code block.
For example, if you tried to declare two variables one after another with the same variable name, you will receive an error.
let website = "pimylifeup.com";
let website = "google.com";
You will get the following error message if you run the above example.
Uncaught SyntaxError: Identifier 'website' has already been declared
Comparison to the var Keyword
However, this behavior will work if you use the older “var
” keyword to declare a variable in JavaScript.
var website = "pimylifeup.com";
var website = "google.com";
let Variables are Block Scoped in JavaScript
The key thing about declaring a variable in JavaScript with the let keyword is that they have block scope.
Block scope means JavaScript cannot access a variable declared within a code block ({ }
) outside the code block.
Let us take a quick look at the following JavaScript example to showcase this. With this, we have a code block in which we declare a variable using JavaScript’s let keyword and assign it a value.
Outside the code block, we use “console.log()
” on the variable. With this, you will see how the variable is undefined as it is outside of the variables block scope.
{
let website = "pimylifeup.com";
}
console.log(website);
If you were to run the above code, you would run into the following error.
Uncaught ReferenceError: website is not defined
Comparison to the var Keyword
The older var keyword in JavaScript doesn’t restrict itself in scope to the current block. You can see this behavior by running the following script.
{
var website = "pimylifeup.com";
}
console.log(website);
If you were to run the above example, you would see that the variable’s result is logged to the console.
pimylifeup.com
You can Redeclare a let Variable in JavaScript if they’re in Separate Blocks
You can only redeclare a variable using the let keyword in JavaScript when they are in a different scope.
The new declaration will be considered an entirely new variable if they are within a separate block. Therefore, you will lose access to the value stored within the original variable.
With this example, we use JavaScript’s let keyword to declare a variable called “website
” outside the code block. This variable will be assigned the value “pimylifeup.com
“.
Inside the code block, we declare the “website
” variable again using the let keyword. We assign this variable the value “google.com
“.
Using the “console.log()
” function after each declaration, you can see how JavaScript separates each value into its own block scope.
let website = "pimylifeup.com";
{
let website = "google.com";
console.log(website);
}
console.log(website);
Below is the output you should get after running the above example. With this result, you can see how each declaration is considered separate.
Redeclaring the variable within the code block does not affect the variable declared outside of it. JavaScript will treat them as entirely different variables.
pimylifeup.com
google.com
Comparison to the var Keyword
If you were to use this same code but use JavaScript’s var keyword instead. With this, you can see how the second declaration overrides the original.
When using “var
“, JavaScript does not take the block scope into consideration.
var website = "pimylifeup.com";
{
var website = "google.com";
console.log(website);
}
console.log(website);
Below you can see how with the var variable, the block scope wasn’t respected by JavaScript.
google.com
google.com
Variables Declared with the let Keyword Operate in the Global Scope
Now that we have gone through how the let keyword works differently. Let us quickly explain some of the things where it works the same.
The first thing is that it can be global when you declare a variable using the let keyword in JavaScript.
A variable is considered to be within the global scope when you declare outside of any function or code block.
Let us show this with a quick example. We start by declaring our “website
” variable using JavaScript’s let keyword within the global scope.
After this, we have a quick function called “printWebsite()
” which uses “console.log()
“, to log the value of the “website
” variable. Since we declared the “website
” variable in the global scope, it will be able to access its value.
Finally, we make a call to the “printWebsite()
” function.
let website = "pimylifeup.com";
function printWebsite() {
console.log(website);
}
printWebsite();
After running the JavaScript above, you can see how a variable declared in the global scope can be interacted within functions and code blocks.
pimylifeup.com
The JavaScript let Variable Respects Function Scope
In addition to global scope, the JavaScript let variable respects function scope. This means that when a variable is declared within a function, it is only available to that function.
We can quickly showcase this with the following example. First we create a quick function called “printWebsite()
“.
Within this function, we declare a variable using the let keyword called “website
” and assign it the value “pimylifeup.com
“. Additionally, we use “console.log()
” to print the variable’s value.
Outside of the function, we make a call to our new function. Immediately after, we attempt to use “console.log()
” to log the value of the “website
” variable.
Now since the variable was declared within the function scope, it won’t be able to access its value and will throw an error.
function printWebsite() {
let website = "pimylifeup.com";
console.log(website);
}
printWebsite();
console.log(website);
You will get the following result if you run the following example within your console. You can see JavaScript safely printed the original value.
However, when the second “console.log()
” function is called a reference error, JavaScript throws a “ReferenceError
“.
pimylifeup.com
Uncaught ReferenceError: website is not defined
Conclusion
This tutorial showed you how to use the let keyword within JavaScript.
The let keyword allows you to declare a more restricted variable than the standard var keyword. For example, variables declared with this keyword can be restricted to a code block.
Please comment below if you have any questions about using the let keyword in JavaScript.
Be sure to check out our many other JavaScript tutorials. We also have other coding guides if you want to learn a new language.