This tutorial will show you how to use the const keyword to declare a variable in JavaScript.
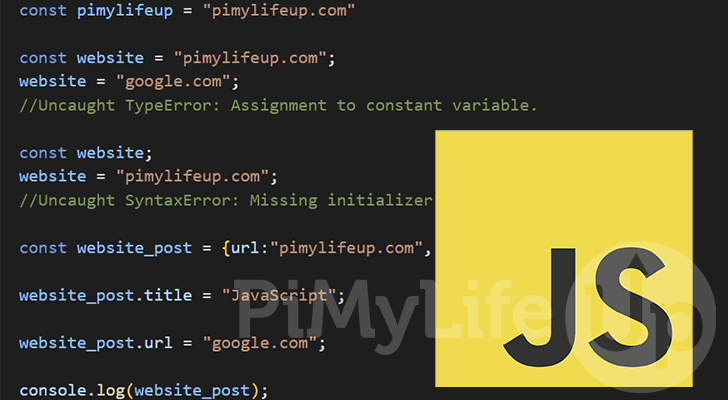
The const keyword is one of the three ways you can declare a variable in the JavaScript language.
What differentiates JavaScript’s const keyword from the others is that once a variable is declared, it cannot be assigned a new value.
This is incredibly useful when you need to declare a value that should never be changed during runtime. In fact, as a general rule, you should declare a variable using “const
” unless you expect the value to be changed.
By the end of this guide, you should have a good understanding of how to declare a variable in JavaScript using const.
Syntax of the const Keyword in JavaScript
Let us start this section by showing you the syntax for JavaScript’s const keyword. You use this keyword just like any other way you would declare a variable.
You cannot declare a variable with const without assigning it a value. An empty constant is useless as it can never be assigned a value.
To declare a constant variable with the const keyword, you only need to use “const
” followed by a variable name and then the value you want to be assigned to it.
const VARIABLENAME = VALUE;
Copy
For example, if we wanted to declare a variable called “pimylifeup
” as a const while also assigning it the value “pimylifeup.com
“, you would use the following code.
const pimylifeup = "pimylifeup.com"
Copy
A Const Variable cannot be Reassigned in JavaScript
One of the key points of using the const keyword to declare a variable in JavaScript is that you can never change its value.
The value you assign during declaration cannot be changed in almost all cases. However, there is a slight edge case that we will cover later on in this guide.
To showcase this, let us write a short JavaScript example where we declare a variable and then attempt to reassign its value.
Here we start by using the const keyword to declare our variable called “website
“. During the declaration we will assign our new variable the string "pimylifeup.com"
.
After declaring the variable, we attempt to assign it a new value.This new value is "google.com"
. Here is where an error would occur.
const website = "pimylifeup.com";
website = "google.com";
Copy
If you tried to run this example, you would recieve the following error message.
Uncaught TypeError: Assignment to constant variable.
Copy
A Const Variable Must be Assigned a Value During Declaration
Another important thing to note about declaring a variable using JavaScript’s const keyword is that it must be declared with a value.
JavaScript will throw an error if you attempt to declare a const variable without setting a value.
The following is the correct way to declare a const variable in JavaScript
const website = "pimylifeup.com";
Copy
However, if you attempt to declare the variable using const without a value like shown below, you will run into an error.
const website;
website = "pimylifeup.com";
Copy
Below is an example of the error message that JavaScript throws.
Uncaught SyntaxError: Missing initializer in const declaration
Copy
Object using JavaScript’s const Keyword
When you declare an object using JavaScript’s const keyword, you will find that you can still change the object’s properties.
This is because JavaScript declares a constant reference to the value. So while you can’t reassign the referenced value, you can adjust the properties of that referenced value.
Let us show this by writing a short example. With this example, we will declare a new object called “website_post
” and give it two properties.
We then adjust two of the object’s properties and log the result. With this example, we aim to show you how the properties of an object can be changed even if it has been declared using the const keyword.
const website_post = {url:"pimylifeup.com", title:"JavaScript const"};
website_post.title = "JavaScript";
website_post.url = "google.com";
console.log(website_post);
Copy
After running the above example, you should end up with the following within the terminal. Here you can see that even though the object was defined using “const
“, we could adjust the properties.
{
"url": "google.com",
"title": "JavaScript"
}
Copy
However, if you were to attempt to re-assign the value as we have shown below, you will run into an error.
const website_post = {url:"pimylifeup.com", title:"JavaScript const"};
website_post = {url:"pimylifeup.com"};
Copy
If you use the example above, you will see the following error thrown by JavaScript.
Uncaught TypeError: Assignment to constant variable.
Copy
Declaring an Array using JavaScript’s const Keyword
When you declare an array with the const keyword in JavaScript, you can still add, remove and change elements within the array.
While you can change elements of an array, it is crucial to note that you still can’t re-assign the variable. This is because when we change an element, we modify the referenced array, not the reference itself.
To showcase this, we will use JavaScript’s const keyword to declare an array called “ages
“. Within this array we will add a few random elements.
We then proceed to show you how you can adjust the elements within an array, by using the “.pop()
” and “.push()
” functions of the array.
const ages = [18, 23, 45, 32, 27];
ages.push(50);
console.log(ages);
ages.pop();
ages.pop();
console.log(ages);
Copy
After running the code, you should end up with the following result within the terminal. With this, you can see how we could re-adjust the elements within the array.
[18, 23, 45, 32, 27, 50]
[18, 23, 45, 32]
Copy
However, if you try re-assigning the array with a new value, you will run into an error. You can only adjust elements of the array.
const ages = [18, 23, 45, 32, 27];
ages = [50];
Copy
Below is the sort of error you will see after trying to re-assign a variable after declaring it with the const keyword in JavaScript.
Uncaught TypeError: Assignment to constant variable.
Copy
A const Variable has Block Scope
Like the let keyword, when you use const to declare a variable in JavaScript, it will have block scope.
Block scope is when JavaScript can’t access that variable when declared within a separate code block ({ }
). However, if declared in a parent code block, you can still access a const variable.
To showcase this behavior, we will write a short JavaScript example where we declare a const variable within a code block.
We will then use the “console.log()
” function to try and output the value of this variable. This function call is what will show us how we can’t access the variable.
{
const website = "pimylifeup.com";
}
console.log(website);
Copy
After running the above JavaScript, you will see the following error message.
Uncaught ReferenceError: website is not defined
One important thing to note about block scope is that you can declare a variable twice if they are within block different scopes.
While these variables can have the same name, they are considered entirely separate when defined in different blocks. Therefore, you will lose access to any variable using the same name declared in the parent block.
The example below showcases this behavior. You won’t run into a redeclaration error despite using the same variable name.
const website = "google.com";
{
const website = "pimylifeup.com";
}
console.log(website);
Copy
You will get two distinct values logged to the console if you run the above JavaScript.
google.com
pimylifeup.com
Conclusion
This tutorial showed you how to use the const keyword to declare a variable.
A variable declared with const in JavaScript cannot have its value changed after assignment. However, you can still change the properties of objects.
Please comment below if you have any questions about using const in JavaScript.
To learn more, be sure to check out our other JavaScript tutorials. We also have other guides if you want to learn a new programming language.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support