Learn how strings are handled in the Python programming language with this guide.
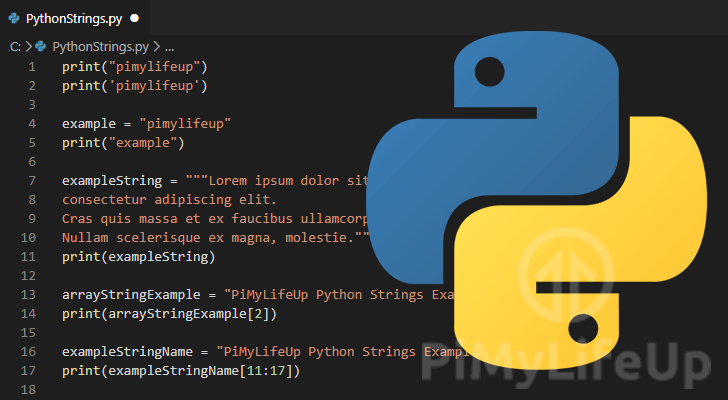
Strings are a highly important concept to learn how to deal with in any programming language, and Python is no different.
Within this guide, we hope to teach you the basics of strings in Python and how to handle them.
We will also be running through some of the helper functions available within the Python programming language.
Before you begin this tutorial we highly recommend that you check out both our getting started with Python guide and our introduction to Python syntax.
Python Strings Index
If you are after a particular topic on strings in Python, then you can make use of the index below to find it quickly.
- String Literals in Python
- Assigning a String to a Variable in Python
- Defining a Multi-line String
- Strings are Arrays
- Getting the Length of a String
- Escaping Characters in Strings
- Checking if a String Contains
- Formatting Strings
- Methods to Manipulate Strings
String Literals in Python
In Python, string literals are represented by either single quotation marks (' '
) or double quotation marks (" "
).
What this means is that if you use single or double quotation marks, it will be considered to be the same value.
For example, the string 'pimylifeup'
is deemed to be the same as "pimylifeup"
by the Python interpreter.
It is also possible to display a string literal without having to assign it a variable first by using the print()
function.
print("pimylifeup")
print('pimylifeup')
Copy
Assigning a String to a Variable in Python
Assigning a string to a Python variable is a straightforward task.
To assign a string to a variable, all you need to do is use the variable name, followed by the equals sign (=
). followed by the string you want to set ("example"
).
Just remember to define a string you need to wrap it in single or double-quotes.
In our example below you can see that we set assign our variable called example
the string value of "pimylifeup"
.
example = "pimylifeup"
print(example)
Copy
Defining a Multi-line String
With the Python programming language, it is possible to define strings that span multiple lines.
To be able to define a multi-line string you need to start and end the string with three double quotation marks("""
).
Line breaks are inserted where they exist within the defined string.
Example using Double Quotes
exampleString = """Lorem ipsum dolor sit amet,
consectetur adipiscing elit.
Cras quis massa et ex faucibus ullamcorper.
Nullam scelerisque ex magna, molestie."""
print(exampleString)
Copy
Strings are Arrays
One important thing to understand about strings in Python is that they can be treated just like an array.
Every character is a position in the array, with the first letter of a string being at index 0.
For example, let’s take a look at how a string with the value of “pimylifeup
” would look if we wanted to inspect each letter individually .
[0] [1] [2] [3] [4] [5] [6] [7] [8] [9]
P I M Y L I F E U P
Copy
Grabbing a Single Letter from a String
As you can treat a string as an array, it is fairly straightforward to grab a single letter from it.
exampleStringName[Index Position]
Copy
All you need to do is make use of your string variable, the square brackets ([ ]
), and the index position of the letter you want to grab.
Example of Retrieving a Single Character
For example, let’s say we have a string called arrayStringExample
with a value of "PiMyLifeUp Python Strings Example"
.
If we want to retrieve the third letter of this string, we would need to use the index position 2
within the square brackets([ ]
).
Remember that when counting, 0 is the first letter of the string.
arrayStringExample = "PiMyLifeUp Python Strings Example"
print(arrayStringExample[2])
Copy
Slicing a String
Just like dealing with arrays in Python, it is possible to slice a string.
By slicing a string, you can retrieve a selection of characters instead of just one.
exampleStringName[StartIndexPosition<strong>:</strong>EndIndexPosition]
Copy
To slice a string, you will need to know the start and end position of the text you want.
The end position should be the index after the last character you want. You will see this behavior in the following example.
Example of using Slice on a String
For this example, we are going to assume we have a variable called “exampleStringName
” with the string "PiMyLifeUp Python Strings Example"
set to it.
exampleStringName = "PiMyLifeUp Python Strings Example"
Copy
With this, we are going to take the word "Python"
out of our string.
To get this word, we need to slice from index 11 (Start of the word) to index 17 (Just after the word).
exampleStringName = "PiMyLifeUp Python Strings Example"
print(exampleStringName[11:17])
Copy
If we have gotten the two index positions correct, you should see the following result be printed out.
Python
Copy
Getting the Length of a String
There are various moments where you might want to get the length of a string within Python.
To be able to get the length of a string, we need to make use of the len()
function.
length = len(VariableName)
Copy
When the len()
function is used on a variable that is a string, it returns the number of characters present in it.
Example of Using len()
to Get the Length of a String
In this example, we are going to get the length of a string with the value of "PiMyLifeUp Python Strings Example"
.
We are going to achieve this by making use of the len()
function.
exampleStringName = "PiMyLifeUp Python Strings Example"
print(len(exampleStringName))
Copy
Upon running this code sample you should get back the length of our string.
33
One thing you should know is that the len()
method returns the number of characters, counting from 1, not 0.
If you want to use this method to get the last character in a string, you will need to subtract one from the result.
Escaping Characters in Strings
Sometimes there are certain characters you might want to use in your string that might break the string. To solve this issue, we are going to dive into something called escaping.
Within this section, you will learn some of the usages of the escape character (\
) in Python.
Escaping can also be used to define certain things such as newlines (\n
).
Example of an Illegal Character
One of the first things you should learn to identify is the use of an “illegal” character.
An illegal character is any character that breaks the definition of that string, making it impossible for the interpreter to understand it.
To showcase this, we are going to use a sample string where we use the double quotes to define the string while also trying to use them within the string.
sampleString = "Hello this is a simple sample <strong>"</strong>string<strong>"</strong> that showcases escaping"
Copy
If you try to run this code within Python, you will see the following error.
SyntaxError: invalid syntax
This error indicates that the Python interpreter was unable to understand what’s written as it doesn’t match the expected syntax.
To solve this error, you can make use of the escape character (\
) next to each of the double-quotes.
By doing this, Python will interpret those as the literal double-quote rather than part of the programming syntax.
sampleString = "Hello this is a simple sample <strong>\"</strong>string<strong>\"</strong> that showcases escaping"
Copy
Other uses of the Escape character
Below is a table that shows you the other uses of the escape character within a string in Python.
Code | Result |
---|---|
\' | Single Quote (' ) |
\" | Double Quote (" ) |
\\ | Back Slash (\ ) |
\n | New Line |
\r | Carriage Return |
\t | Tab |
\b | Backspace |
\f | Form Feed |
\ooo | Octal Value |
\xhh | Hex Value |
Checking if a String Contains
If you want to check whether a string contains or doesn’t contain a certain phrase, Python has two keywords that come in handy.
These two keywords are in
and not in
. Both of these keywords are case sensitive when they compare, so the word “Hello
” will not match “hello
“.
"Hello" in samplePhrase
"Hello" not in samplePhrase
Copy
Both of these keywords work by you first specifying the character (or string) you want to check for, followed by the keyword (in
or not in
), followed by the string that you want to check.
For our two examples, we will be making use of a variable called examplePhrase
that has the value of "Pi My Life Up's Tutorial on String in Python"
.
The in
Keyword and Strings
By making use of the in
keyword, you are able to see if a character or string is present in another string.
"Hello" <strong>in</strong> samplePhrase
Copy
The in
keyword will return True when the phrase or character is found and False when i’ts not.
Example of Using the in
Keyword on Strings
To show you how the in
keyword works, we are going to check our samplePhrase
variable to see if the word “Tutorial
” exists within it.
samplePhrase = "Pi My Life Up's Tutorial on String in Python"
hasTutorial = "Tutorial" in samplePhrase
print(hasTutorial)
Copy
As we are using the in
keyword, we should see a True
result be printed out by the example above as the word Tutorial
exists in the phrase.
The not in
Keyword and Strings
The not in
keyword can be used to check whether a particular string or character is not present within another string.
"Hello" <strong>not in</strong> samplePhrase
Copy
Using the not in
keyword will return True
when the phrase or character doesn’t exist in the string, and False
when it does exist.
Example of Using the in
Keyword on Strings
To give you an idea of how the not in
keyword works, we are going to be checking to see if our sample phrase contains the word "Raspberry"
.
samplePhrase = "Pi My Life Up's Tutorial on String in Python"
doesntHaveRaspberry = "Raspberry" in samplePhrase
print(doesntHaveRaspberry)
Copy
As our phrase doesn’t contain the word Raspberry
, you should see the result True
be printed out by Python.
Formatting Strings
One of the most useful things to learn about handling strings in Python is how to format them.
This section will quickly run you through one of the most useful string functions, the format()
function, and the curly bracket placeholders ({ }
).
If you have followed our guide on Python variables, you should have already learned that you can’t add a numerical number to a string.
Even though there is a way to convert a number to a string, there is a much cleaner way of achieving this.
The format()
Method
Using the format()
method, you can take a string in Python that contains placeholders ({ }
) and insert data into it.
"My Example String with {}".format("Replacements Go Here")
Copy
For example, using this method, we can take a string like "My age is { }"
and insert the value 20
.
"My age is {}".format(20)
Copy
Each additional placeholder you add requires you to specify more parameters within the .format()
method.
How to use Empty Placeholders
The easiest way of using placeholders with the .format()
method is to make use of the empty {}
placeholders.
The order in which the placeholders are defined is the same order in which their values need to be specified in the format()
method.
To show you how this works, we are going to give you an example of how to use empty placeholders in Python.
Example of Empty Placeholders
For this example, we are going to start with a variable called exampleString
.
This variable will store a string with the phrase "My name is {}, and I am {}"
.
Into this string, we are going to insert the word "PiMyLifeUp"
and the number 5
by using the format() method.
exampleString = "My name is <strong>{}</strong>, and I am <strong>{}</strong>"
exampleString.format(<strong>"PiMyLifeUp"</strong>, <strong>5</strong>)
print(exampleString)
Copy
From this code, you should see a result as we have below.
My name is PiMyLifeUp, and I am 5
Using this, you can tell that our placeholders were replaced in the same order in which we passed them in.
So if we flipped the parameter order, we would end up with the following string instead.
exampleString = "My name is {}, and I am {}"
exampleString.format(5, "PiMyLifeUp")
My name is 5, and I am PiMyLifeUp
Copy
Numbered Placeholders in Python
In Python, you can also number each of your placeholders. By giving it a number, you can dictate the order in which you specify its value.
You can specify a number by placing it in between the two curly brackets ({0}
).
"My age is {1}, my name is {0}".format("PiMyLifeUp", 5)
Copy
Always remember when specifying the position that you start counting from 0, not 1.
Using numbered placeholders can be useful when you are formatting different strings where the placement of the placeholders is different.
It is also helpful if you want to use the value for that placeholder in multiple places within the same string.
Example of using Numbered Placeholders
In this example, we are going to show you how numbered placeholders work by showing you two different strings that we pass the name format()
parameters in to.
To show this we are going to use string "My age is {0}, my name is {1}"
and the string "My name is {1}, my age is {0}"
.
exampleString1 = "My age is {0}, my name is {1}"
exampleString2 = "My name is {1}, my age is {0}"
print(exampleString1.format(5, "PiMyLifeUp"))
print(exampleString2.format(5, "PiMyLifeUp"))
Copy
If you were to run this code snippet within Python you should end up getting a result like we have below.
My age is 5, my name is PiMyLifeUp
My name is PiMyLifeUp, my age is 5.
Copy
As you can see, despite the parameters being used in the same order, both strings are still inserted at their correct spots thanks to the use of the numbered placeholders.
Using Named Placeholders
The third and final way of using placeholders in Python is to use named placeholders.
What this entails is that you specify a name within the curly brackets ({ NAME }
).
This name you specify is crucial as you will need to use it when setting it a value within the format()
method.
For each placeholder, you need to use the placeholder name, followed by the equals sign (=
) then the value you want to be assigned to it.
"Welcome to {website}".format(website="PiMyLifeUp.com")
Copy
One of the biggest reasons you might want to use this method is that when used correctly, it can dramatically improve the readability of the code.
When you are formatting a string that contains placeholders, it becomes self-evident on what is going to be placed in that position.
Example of using Named Placeholders
Below we are going to give you a quick idea of how to make use of a named placeholder.
For this example, we are going to use the string "Hello {name}, welcome to {website}"
At a glance, you can see that this string has two named placeholders, name
and website
.
exampleString = "Hello {name}, welcome to {website}"
print(exampleString.format(name="World", website="PiMyLifeUp.com"))
Copy
Using the above code, we insert the text "World"
in place of name and the string "PiMyLifeUp.com"
in place of website.
If you run the code above in Python you should end up with the following result.
Hello World, welcome to PiMyLifeUp.com
Methods to Manipulate Strings
Within this section, we are going to run through some of the various methods that you can use to manipulate strings.
You can make use of these methods by using the dot (.
) on the variable followed by the method name.
Removing Whitespace using strip()
The strip()
method is used to remove any whitespace from the begining or end of a string.
stringVariableName = stringVariableName.strip()
Copy
Using this method, you can take a string like " PiMyLifeUP String Example "
and turn it into "PiMyLifeUp String Example"
.
This method can be useful for processing strings where extra whitespace may have been added.
Example of Using the strip()
Method
Below we are going to show you an example of how to use the strip()
function.
We are going to define a string called exampleString
with the value of " Hello I Have Unneeded Whitespace "
.
As you can see, we have added two characters of whitespace to the start and the end of the string that we don’t want.
exampleString = " Hello I have Unneeded Whitespace "
print(exampleString.strip())
Copy
By making use of the strip method, we can output the following string.
Hello I have Unneeded Whitespace
You will see that both pieces of whitespace at either end of the string has been stripped out.
Converting a String to Lowercase using lower()
The lower()
method can be used to convert a string entirely to lowercase.
stringVariableName = stringVariableName.lower()
Copy
By using this method, you can convert a string from uppercase to lowercase.
For example, the string "PiMyLifeUP"
would become "pimyifeup"
.
Example of Using the lower()
Method
To show you how this works, we are going to use the lower()
method on our variable called exampleString
with the value of "MiXeD CaSE StRiNG ExAMPLE"
.
exampleString = "MiXeD CaSE StRiNG ExAMPLE"
print(exampleString.lower())
Copy
Upon running this Python code snippet, you should get a result as we have below.
mixed case string example
You will see that each letter has been converted to lowercase.
Converting a String to Uppercase using upper()
The upper()
method is the opposite of the lower()
method in that it converts all letters to their uppercase equivalent.
stringVariableName = stringVariableName.upper()
Copy
Using this method, you can convert a string so that every letter is uppercase.
For example, we can change the string "pimylifeup"
to "PIMYLIFEUP"
with ease by using this method.
Example of Using the upper()
Method
Making use of the upper()
method is a reasonably straightforward process.
For this example, we are going to show you how to use the method to convert our example string from "all lower case example string"
to uppercase.
exampleString = "all lower case example string"
print(exampleString.upper())
Copy
By running the code from above, you should see the following result. Each letter should now be in uppercase.
ALL LOWER CASE EXAMPLE STRING
Replacing Within a String by using replace()
If you would like to replace an element within a string, you can make use of the replace()
method.
The method takes in three different parameters. The first two are required, the third is optional.
The first two required parameters ask you to specify the text you want to find, and the text you want to replace it with.
The third parameter allows you to specify how many occurrences you want to replace.
stringVariableName = stringVariableName.replace("FIND", "REPLACE", [OCCURRENCES])
Copy
Using this method, you can take a string like "Two Two Three Four Five"
and replace the first occurrence of Two
with One
.
Example of Using the replace()
Method
Below we are going to give you two different examples of how you can use the replace()
method.
Example 1
In this first example, we are going to use the example string value of “Two Two Three Four Five
” and replace the word “Two
” with “One
“.
We do this by using the replace()
method and parsing in "Two"
for the first parameter and "One"
for the second parameter.
So "Two"
is the value we are looking for, and "One"
is the string we want to replace it with
exampleString = "Two Two Three Four Five"
print(exampleString.replace("Two", "One"))
Copy
Using this code snippet, you should end up seeing the result below.
One One Three Four Five
From the result above, you can see that all occurrences of Two
were replaced with One
.
Example 2
With this example, we are going to take the same example string as above but this time only replace one occurrence of "Two"
We achieve this by making use of the third, optional parameter. With this parameter. we will pass the number 1
in.
exampleString = "Two Two Three Four Five"
print(exampleString.replace("Two", "One", 1))
Copy
From this code, you should see the following text printed out.
One Two Three Four Five
As you can see, only one of the occurrences of "Two"
has been replaced, with the second one remaining in the string.
Splitting a String using the split()
Method
The split()
method can take a string and split it into a list.
This method features an optional parameter that lets you define what you want to use for the separator. By default, any whitespace is used as the separator between elements.
stringVariableName = stringVariableName.split([<strong>SEPERATOR</strong>])
Copy
For example, using this method you can take the text "one,two,three,four"
and split it into a list like so ["one", "two", "three", "four"]
Example of Using the split()
Method
Below we are going to give you two examples of using the split()
method, one without using the parameter and one with the separator parameter defined.
Example 1
With this example, we are going to take the following string "one two three four"
and convert it into a list.
As each element is only separated by whitespace, we can make use of the split()
method without making use of the optional parameter.
exampleString = "one two three four"
print(exampleString.split())
Copy
From this code snippet, you should see the following list print out.
['one', 'two', 'three', 'four']
Copy
Example 2
Let’s now take another string, this time, one that uses a comma (,
) to separate each element instead of whitespace.
This example string is set to "one,two,three,four"
.
exampleString = "one,two,three,four"
print(exampleString.split(','))
Copy
Using this example code snippet, you should see the following result.
['one', 'two', 'three', 'four']
Copy
Hopefully, you now have an understanding of how you can deal with strings within the Python programming language
If you have found issues with this guide or have any suggestions, feel free to leave a comment below.
So far a nice little tutorial, checked it out because I’ve never used Python until now, only used different kinds of Basic in the past.
Some things I’ve noticed:
[[SNIPPED]]
Hi Konfuzius,
Thank you very much for reporting those mistakes, I have now corrected them.
Cheers,
Emmet