In this tutorial, we take you through how to use the print() function in the Python programming language.
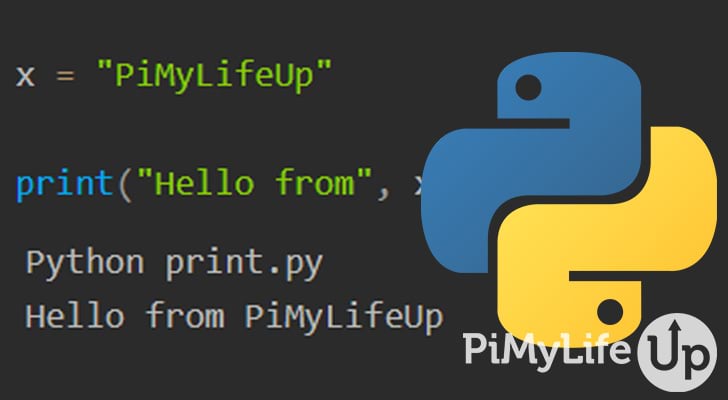
You will see and use the print function a lot in Python scripts, so it is important that you learn the basics of how you can utilize it. In this tutorial, we will touch on the syntax of the function and several examples of how you can use it in your Python script.
The print function will print a message to the terminal or selected output file. The message can be an object, string, or combination of different datatypes. Whatever you specify, the print function will convert them to a string before being outputted to the terminal or file.
In Python 3, the print statement was replaced by the print function. There are quite a few reasons why this change occurred, but it is only really a concern if you are editing a script made for Python 2.
Table of Contents
Syntax of the Python print() Function
The print function accepts five different parameters, but only the first one is required. We briefly touch on each parameter that you can use below.
print(object, sep=' ', end='\n', file=sys.stdout, flush=False)
- Object is the object that you want to print. You can use multiple objects or a single object. The print function will convert the object to a string before outputting it to the display.
- sep=’ ‘ indicates what to use to separate objects when multiple of them are specified. This parameter is optional with the default setting set to ‘ ‘.
- end=’\n’ allows you to specify what to print at the end of the message. This parameter is optional and has a default set to ‘\n’ (Newline Character).
- file=sys.stdout can be set to an object with a write method or a file with write permissions. The default is sys.stdout (standard output). This parameter is optional.
- flush=False specifies if you want the output flushed (True) or buffered (False). This parameter is optional, and the default setting is set to False.
All but the first parameter are keyword arguments, also known as named arguments. These are identified by specific names such as sep or end. Since they are identified by keyword, it does entirely matter what order they are specified. However, object(s) will need to come first as they are positional arguments.
Examples of Using the print() Function in Python
The print function is versatile, so there are quite a few different ways you can utilize this function to print data to your output. Below we go through several examples of how you can use this function in your Python program.
Printing a String
The most basic and likely the most common use of the print function is for outputting a single string or object. In the example below, we simply use the function to print the string “Welcome to PiMyLifeUp“.
print("Welcome to PiMyLifeUp")
If we run the above code, we will receive our string message in the terminal, as shown in the example below.
Python print.py
Welcome to PiMyLifeUp
Printing a Dictionary
In this example, we will print a Python dictionary using the print function. You can print other objects and data types such as tuples, lists, sets, and much more.
fruitDict = {
"fruit": "Apple",
"healthy": True,
"calories": 95,
"colors": ["red", "yellow", "green"]
}
print(fruitDict)
As you can see in our output below, Python printed the entire dictionary to the terminal when we ran the script.
Python print.py
{'fruit': 'Apple', 'healthy': True, 'calories': 95, 'colors': ['red', 'yellow', 'green']}
Printing Multiple Objects
You can output multiple objects or data types using a single print function. In addition, using a single print function can help keep code clean and readable.
Below is an example of using print to output two different strings with one stored in a variable x.
x = "PiMyLifeUp"
print("Hello from", x)
In our terminal, our output contains the two strings we specified as parameters in our Python print function.
Python print.py
Hello from PiMyLifeUp
Using the Separator and End Parameters
The print function allows you to specify a separator (sep=""
) for when multiple objects are specified. For example, you may want a newline (\n) between objects or a simple “–“. The default separator is a single space.
You can specify an ending (end=''
) that will print after the print function has outputted all the objects. By default, the end is set to a newline character (\n), but you can change it to something else if required.
The example below demonstrates how you can specify a new separator and a new ending in the print function.
x = "PiMyLifeUp"
print("Hello from", x, sep="\n", end="\nEND")
In our output below, you can see each of our strings is separated by a newline. Lastly, END is printed on a newline after both of our objects have been printed.
PS G:\Python> Python print.py
Hello from
PiMyLifeUp
END
Using a File Parameter
By default, the print function in Python will output any text to sys.stdout (standard output) which appears in your terminal. You can change this behavior by specifying a file or using sys.stderr (standard error).
In the example below, we open a file named logFile.txt
using the open function with write mode (w) enabled. Write mode will create the file if it does not exist.
Next, we specify our txt file as the file parameter in the print function. Doing this will output our string into the file rather than the terminal (sys.stdout).
Lastly, we close the file before the Python script ends.
logFile = open('logFile.txt', 'w')
print('Hello from PiMyLifeUp', file=logFile)
logFile.close()
After running the script above, you should be able to open the logFile.txt
and find the following text inside.
Hello from PiMyLifeUp
Conclusion
By now, you should have a basic understanding of how the print function works within the Python programming language. First, we touched on the function’s syntax and went through several different examples of what you can do.
There is a considerable number of different functions, methods, and data types that you can utilize within Python. I recommend checking out some of our other Python tutorials to learn more about using this programming language effectively.
If we can improve this tutorial, please do not hesitate to let us know by leaving a comment below.