In this guide, we will be showing you how it is possible to convert a string to an Integer in Python.
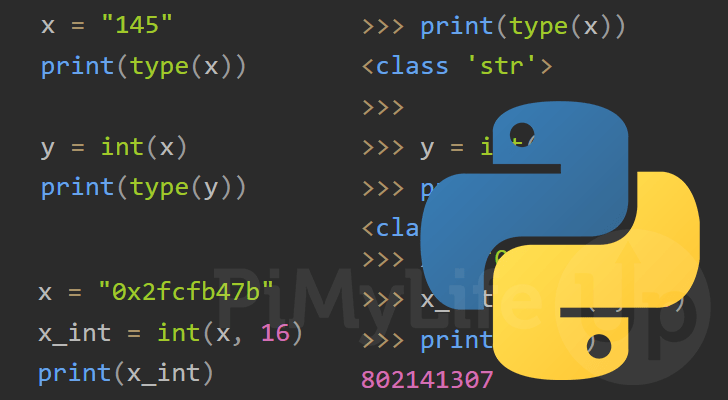
Python dynamically assigns a type to a variable upon its assignment. To change the variable type, you will need to rely on built-in functions.
The function that allows us to convert a string to an integer with Python is the “int()
” function. The int function will convert the number using “base 10” by default. Base 10 is what the decimal system is built-upon.
Unlike converting an integer to a string in Python, there is only one function that you will find helpful.
Over the next section, we will show you how it is possible to use the “int()
” function to convert a string to an int. The, we will show you various cases, including handling octal, hexadecimal, and binary numbers.
Table of Contents
- Using int() to Convert a String to an Integer in Python
- Convert a String to an Integer in Python using the Decimal System
- Using Python to Convert a String to an Integer using the Hexadecimal System
- Converting a Binary String to an Integer using Python
- Using Python to Convert an Octal String to an Integer using Python
- Conclusion
Using int() to Convert a String to an Integer in Python
The main way to convert a string to an integer in Python is by utilizing an in-built function.
The function that we are utilizing for this tutorial is called “int()
“. It will convert any string to an integer, by default using base 10.
Below you can see the syntax of the “int()
” function. We will be showing you how to use this function over the following few sections.
int(STRING, BASE)
Copy
The first parameter (STRING
) is required and is where you will reference your string either directly or by referencing a variable.
The second parameter (BASE
) is optional and is where you will define the base. You will need to adjust the base if you are dealing with a number that isn’t a decimal (base 10).
Convert a String to an Integer in Python using the Decimal System
For this example, we will be showing you the simplest way to convert a string to an integer using Python.
With a decimal number, you don’t have to worry about changing the base to utilize the following syntax.
int(STRING)
Copy
When you don’t specify the base, the int()
function will assume the string you are converting is using base 10 (Decimal).
Below we will write a quick Python script that shows this functionality.
Example of Converting a String to an Integer using the Decimal System
Start by creating a string by wrapping the number 145
in double quotes ( " "
). Then, we will assign this value to our variable called “x
“.
Using both “print()
” and “type()
“, we can verify that this is, in fact, a string before proceeding.
Finally, we can use Python’s “int()
” function to convert our “x
” variable from a string to an integer. Finally, we store the result of the “int()
” function to our “y
” variable.
We verify that our variable “y
” is now an integer by printing out its type.
x = "145"
print(type(x))
y = int(x)
print(type(y))
Copy
By using this code, you will see how the “int()
” function easily provides us with an integer.
>>> x = "145"
>>> print(type(x))
<class 'str'>
>>>
>>> y = int(x)
>>> print(type(y))
<class 'int'>
Copy
Using Python to Convert a String to an Integer using the Hexadecimal System
This section will show you how you can convert a string containing a hexadecimal number to an integer.
There are two ways that we can achieve this using the int function. Both expect different types of strings passed through to it.
Method One – Using Base 16 to Convert a Hex to Integer
The first method sets the base value on the int()
function to 16
(hex). Remember that the function’s second parameter allows you to set the baser number.
This method is the best when you expect your string always contains a hexadecimal number. It accepts a hexadecimal using the format “0x2fcfb47b
” or “2fcfb47b
“.
Below you can see the syntax for using the “int()
” function with a base of 16.
int(HEXADECIMALSTRING, 16)
Copy
Example of Converting a Hex to an Integer by Using Base 16
For this example, let us show you how setting a base 16 for Pythons int function works.
We will create two variables, one called “x
” with the hex string “0x2fcfb47b
“, and “y
” with “2fcfb47b
“.
On each of our strings, we use Python’s “int()
” function to convert them from a string to an int.
By setting the base to 16
, the “int
” function will be able to convert our hexadecimal number to a number that we can store in our integer.
We use Pythons “print()
” function so that we can see the converted values after the int function has converted our strings to an integer.
x = "0x2fcfb47b"
x_int = int(x, 16)
print(x_int)
y = "2fcfb47b"
y_int = int(y, 16)
print(y_int)
Copy
By setting the base to 16
, you can see how the int function happily handled both of our strings. Even with the hex string missing the “0x
” identifier.
>>> x = "0x2fcfb47b"
>>> x_int = int(x, 16)
>>> print(x_int)
802141307
>>>
>>> y = "2fcfb47b"
>>> y_int = int(y, 16)
>>> print(y_int)
802141307
Copy
Method Two – Using Base 0 to Convert a Hex String to an Integer
Alternatively, if your hexadecimal strings are always prefixed with “0x
” you can set the base to 0
.
With the base set to 0, the “int()
” function will attempt to work out the correct base for the number itself.
Without the “0x
” at the front of your number, the int function can’t determine that it’s a hexadecimal number.
Below you can see how you would write the “int()
” function when setting the base to 0.
int(0xHEXADECIMALSTRING, 0)
Copy
This method is useful when you know how the numbers will be formatted and might need to deal with strings containing other types of numbers.
Example of Converting a Hex to an Integer by using Base 0
For this example, we will be using the same data we used for method one. This will show how using base 0 with the “int()
” function will automatically convert a hex string when it is prefixed with “0x
“.
Start with the “x
” variable and assign it the value, “0x2fcfb47b
“. Then create the “y
” variable and give it the value “2fcfb47b
“.
We then use the “int()
” function on both of these variables to transform the strings into integers. This time we use the base 0
.
x = "0x2fcfb47b"
x_int = int(x, 0)
print(x_int)
y = "2fcfb47b"
y_int = int(y, 0)
Copy
Running this code, you can see our string prefixed with “0x
” was converted from a string to an integer.
However our other string, threw an error when ran through the “int()
” function as it was unable to determine the data type.
>>> x = "0x2fcfb47b"
>>> x_int = int(x, 0)
>>> print(x_int)
802141307
>>>
>>> y = "2fcfb47b"
>>> y_int = int(y, 0)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: invalid literal for int() with base 0: '2fcfb47b'
Copy
Converting a Binary String to an Integer using Python
You can also convert a string containing a binary number within Python. We achieve this by setting the base to “2
“, or with a formatted string, you can even use a base set to “0
“.
Method One – Converting Binary to an Integer by using Base 2
Setting the base to 2, the “int()
” function will convert your number from a binary string to a number. Ensure your number uses the “010101
” or “0b010101
” format.
Using the “int()
” function to convert your strings is useful when you know it will always contain a binary number.
int(BINARYSTRING, 2)
Copy
Example of Using Base 2 to Convert Binary to an Integer
For this example, we will create two variables, one called “x
” with the value “0b010101
” and one named “y
” that has the value “010101
“.
We then utilize the “int()
” function, setting the base value to 2
. It will convert both of our binary strings to their integer value.
x = "0b010101"
x_int = int(x, 2)
print(x_int)
y = "010101"
y_int = int(y, 2)
print(y_int)
Copy
Below you can see the result of running the above code. You can see by using base 2
, Python’s “int()
” function converted each string to an integer.
>>> x = "0b010101"
>>> x_int = int(x, 2)
>>> print(x_int)
21
>>>
>>> y = "010101"
>>> y_int = int(y, 2)
>>> print(y_int)
21
Copy
Method Two – Converting Binary to an Integer by using Base 0
Like with hexadecimal numbers, as long as you prefix the number with “0b
“, you can use the “int()
” function with a base of 0
.
The “int()
” function uses this prefix to work out how to convert the number.
int(0bBINARYSTRING, 0)
Copy
Example of Converting Binary to an Integer by using Base 0
We start this example off with two binary strings. One is prefixed with “0b
” and the other without.
You will see how setting the base to 0
will allow “int()
” to convert the string prefixed with “0b
” and throw an error on the one missing it.
x = "0b010101"
x_int = int(x, 0)
print(x_int)
y = "010101"
y_int = int(y, 0)
Copy
After running this, you can see the “int()
” function easily handled the string starting with “0b
“. But threw an error with the one missing the identifier.
>>> x = "0b010101"
>>> x_int = int(x, 0)
>>> print(x_int)
21
>>>
>>> y = "010101"
>>> y_int = int(y, 0)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: invalid literal for int() with base 0: '010101'
Copy
Using Python to Convert an Octal String to an Integer using Python
For our final section on converting a string to an integer in Python, we will be exploring dealing with octal strings.
Like the others number systems that we have explored, we will be showing two methods that you can use.
Method One – Converting an Octal String to an Integer using Base 8
This first method allows the “int()
” function to convert an octal string to an integer regardless of the format. We achieve this by setting the “base” to 8
.
Basically, this allows you to use an octal string formatted like “127
” or “0o127
” and convert it to an integer.
int(OCTALSTRING, 8)
Copy
Example of Converting an Octal to an Integer using Base 8
For this example, we will be utilizing two variables, one called “x
” with the value “0o127
” and another called “y
” with the value “127
“.
Both of these contain the same octal number. The “0o
” identifies the number in this string as an octal.
We convert both of these from an octal string to an integer by utilizing Python’s “int()
” function.
x = "0o127"
x_int = int(x, 8)
print(x_int)
y = "127"
y_int = int(y, 8)
print(y_int)
Copy
If you run these lines within your Python interpreter, you will get the following result. Both strings were easily converted from their octal value to an integer.
>>> x = "0o127"
>>> x_int = int(x, 8)
>>> print(x_int)
87
>>>
>>> y = "127"
>>> y_int = int(y, 8)
>>> print(y_int)
87
Copy
Method Two – Converting an Octal to an Integer Using Base 0
The second method means using the “int()
” function with the base set to “0
“.
This requires your string to be prefixed with “0o
“, but allows the “int()
” function to also handle any other formatted number string.
int(0oOCTALSTRING, 2)
Copy
Example of Converting an Octal to an Integer Using Base 0
In this example, we use the same variables we used for our first method. This means a variable called “x
” with the value “0o127
” and one called “y
” with the value “127
“.
The thing that makes this example different is that we will be using base 0
when using the “int()
” function. With base 0
set, the “int()
” function will only be able to convert our string containing the “0o
” prefix.
x = "0o127"
x_int = int(x, 0)
print(x_int)
y = "127"
y_int = int(y, 0)
print(y_int)
Copy
When running this code, the first string is successfully converted from an octal to an integer.
However, while the second will still convert, Python will assume that it is using the decimal format. So the second value will be printed as “127
” instead of “87
“.
>>> x = "0o127"
>>> x_int = int(x, 0)
>>> print(x_int)
87
>>>
>>> y = "127"
>>> y_int = int(y, 0)
>>> print(y_int)
127
Copy
Conclusion
Throughout this tutorial, we have shown you how you can convert a string to an integer in Python.
As a string could potentially contain various different number formats we have explored a few of the most useful ones. However, by changing the base value, you should be able to handle any number you require.
If you have had any issues understanding how to convert a string to an integer, please comment below.
To learn more about Python be sure to read up on our many other Python tutorials.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support